I'm going to set the child GameObject
's SetActive
to true when the parent GameObject
is triggered.
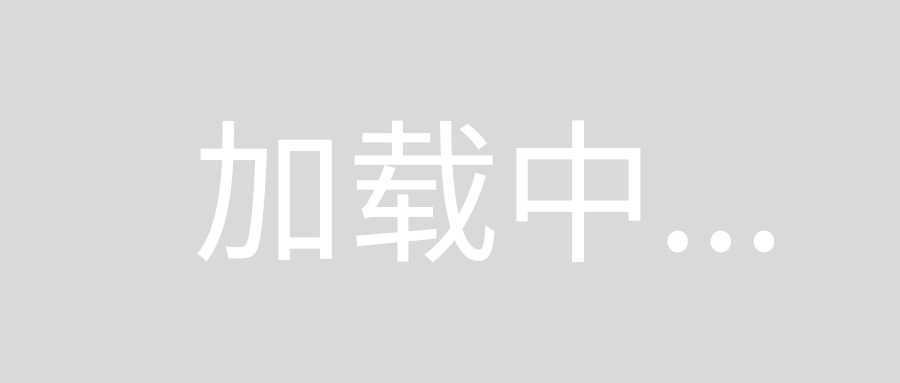
Like this picture, BallIndigo1
is the parent GameoOject
and x
is the child GameObject
which is in SetActive(false)
.
and I wrote code like this:
void OnTriggerEnter2D(Collider2D col)
{
if (col.gameObject.activeSelf == true)
{
if (col.transform.GetChild(0) != null)
{
col.transform.GetChild(0).gameObject.SetActive(true);
}
else
{
Debug.Log("No child");
}
}
}
But the result says:
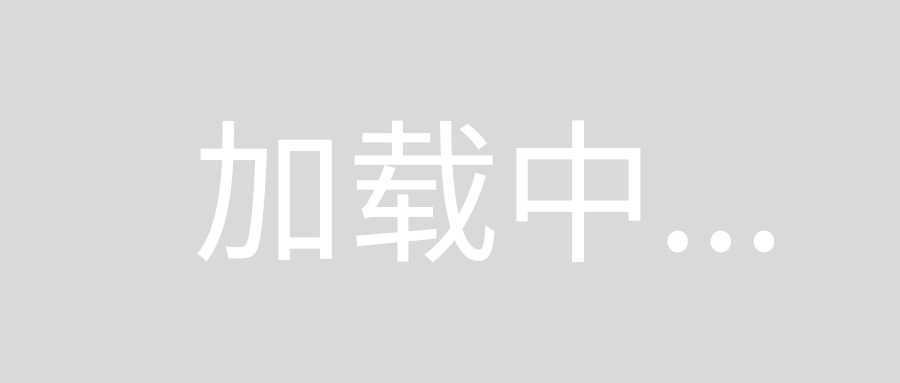
I don't know why this happened and how to fix it.
You are getting this because the GameObject passed to the OnTriggerEnter2D
function col.transform
doesn't have a child. You can fix that by checking if col.transform.childCount > 0
before calling col.transform.GetChild(0)
.
void OnTriggerEnter2D(Collider2D col)
{
if (col.gameObject.activeSelf == true)
{
if (col.transform.childCount > 0 && col.transform.GetChild(0) != null)
{
col.transform.GetChild(0).gameObject.SetActive(true);
}
else
{
Debug.Log("No child");
}
}
}
If you are 100% sure that this Object has a child then there is another GameObject that is being detected that doesn't have a child. You must filter this GameObject out by tag or layer before calling col.transform.GetChild(0)
. Create a tag named "Ball" set the Objects you want to detect to this tag. Make sure that the this "Ball" object has a child. The code below should check for that tag and should get ride of that error.
void OnTriggerEnter2D(Collider2D col)
{
if (col.CompareTag("Ball"))
{
if (col.gameObject.activeSelf == true)
{
if (col.transform.GetChild(0) != null)
{
col.transform.GetChild(0).gameObject.SetActive(true);
}
else
{
Debug.Log("No child");
}
}
}
}