I'm new to wpf and oxyPlot.
Now, I want create a dynamic line chart like an oscilloscope, but I don't know how to lock the axis on a value when mouse wheel zooms.
Example:
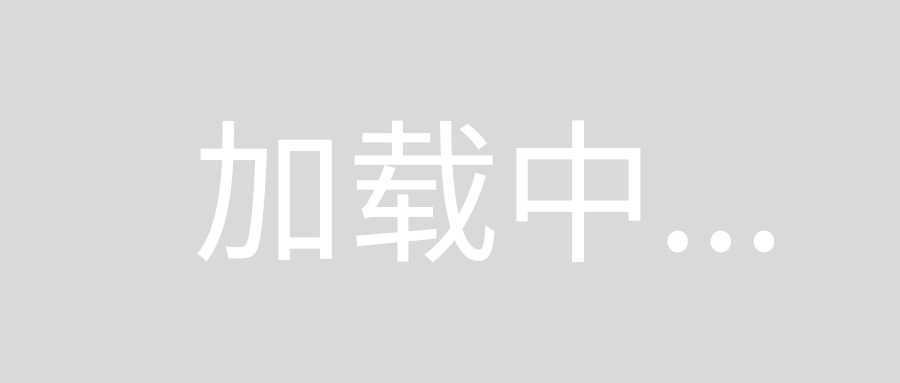
The red point is mouse location. In normal, zoom A -> B, zoom C -> D. now, I want to zoom C ->E, like mouse location at 0 center.
I've found a solution that works for blocking an axis zoom center. You have to create a custom LinearAxis
to achieve this:
public class FixedCenterLinearAxis : LinearAxis
{
double center = 0;
public FixedCenterLinearAxis() : base(){}
public FixedCenterLinearAxis(double _center) : base()
{
center = _center;
}
public override void ZoomAt(double factor, double x)
{
base.ZoomAt(factor, center);
}
}
You have to use it like that:
var bottomAxis = new FixedCenterLinearAxis(0.5) //specify the center value here
{
Position = AxisPosition.Bottom,
};
plotModel.Axes.Add(bottomAxis);
If you dont specify a value on the constructor, center value will be 0.