i am trying to add errorbars using plt.errorbar
to a pointplot
in seaborn:
import matplotlib
import matplotlib.pylab as plt
import seaborn as sns
import pandas
sns.set_style("white")
data = pandas.DataFrame({"x": [0.158, 0.209, 0.31, 0.4, 0.519],
"y": [0.13, 0.109, 0.129, 0.250, 1.10],
"s": [0.01]*5})
plt.figure()
sns.pointplot(x="x", y="y", data=data)
plt.errorbar(data["x"], data["y"], yerr=data["s"])
plt.show()
however the two plots look totally different even though the identical data is being plotted. what explains this and how can errorbars be added to a pointplot?
It seems that sns.pointplot
simply uses an array of [0...n-1]
as x values and then uses the x values that you provide to label the ticks on the x-axis. You can check that by looking at ax.get_xlim()
which outputs [-0.5, 4.5]
.
Therefore, when you provide the actual x values to plt.plot
they just seem to be at a wrong position.
I wouldn't say that this is a bug, since seaborn considers the input to pointplot
to be categorial (here's the documentation for more info)
You can solve this for your simple example by mimicking seaborn
s behavoir:
import matplotlib
import matplotlib.pylab as plt
import seaborn as sns
import pandas
import numpy as np
sns.set_style("white")
data = pandas.DataFrame({"x": [0.158, 0.209, 0.31, 0.4, 0.519],
"y": [0.13, 0.109, 0.129, 0.250, 1.10],
"s": [0.05]*5})
plt.figure()
sns.pointplot(x="x", y="y", data=data)
plt.errorbar(np.arange(5), data["y"], yerr=data["s"], color='r')
plt.show()
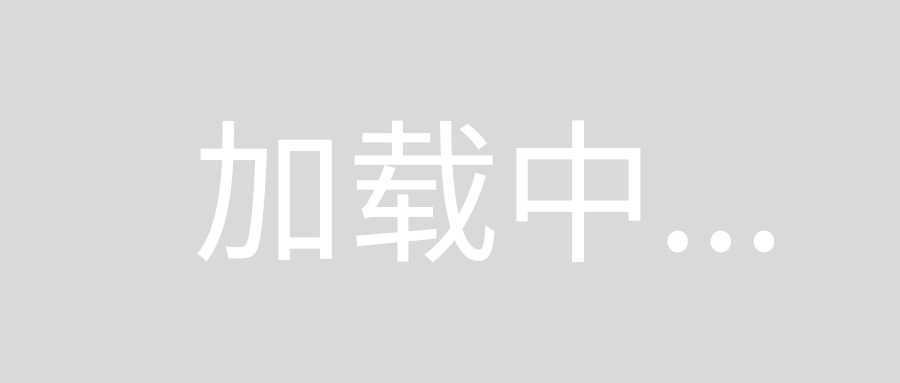