可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am having a slight issue with the use of ValidationSummary(true)
to display model level errors. If the ModelState does not contain model errors (i.e. ModelState.AddModelError("", "Error Description")
) but contains property errors (added using data annotations) it displays the validation summary with no error information (when you view the source). My css is therefore displaying an empty red box like so:
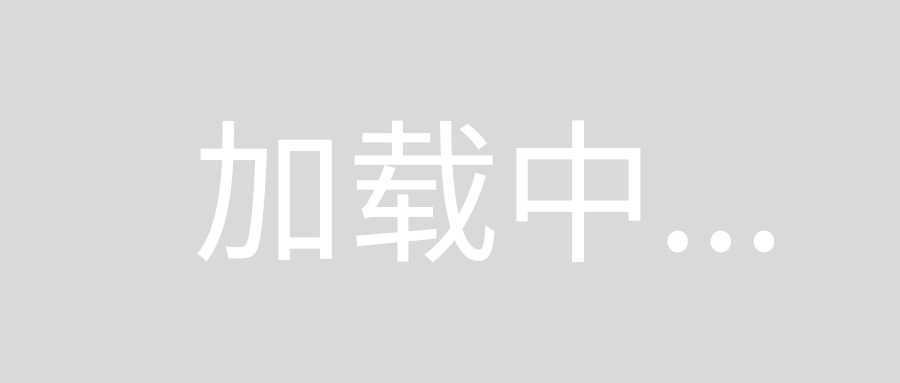
If there are no property errors then no validation summary is displayed. With ValidationSummary(true)
I would expect it to only display validation errors if there are model errors. What have I misunderstood?
I have a basic project as follows:
Controller:
public class HomeController : Controller
{
public ViewResult Index()
{
return View();
}
[HttpPost]
public ActionResult Index(IndexViewModel model)
{
return View();
}
}
Model:
public class IndexViewModel
{
[Required]
public string Name { get; set; }
}
View:
@model IndexViewModel
@Html.ValidationSummary(true)
@using(@Html.BeginForm())
{
@Html.TextBoxFor(m => m.Name)
<input type="submit" value="submit" />
}
回答1:
I think there is something wrong with the ValidationSummary
helper method. You could easily create a custom helper method that wraps the built-in ValidationSummary
.
public static MvcHtmlString CustomValidationSummary(this HtmlHelper htmlHelper, bool excludePropertyErrors)
{
var htmlString = htmlHelper.ValidationSummary(excludePropertyErrors);
if (htmlString != null)
{
XElement xEl = XElement.Parse(htmlString.ToHtmlString());
var lis = xEl.Element("ul").Elements("li");
if (lis.Count() == 1 && lis.First().Value == "")
return null;
}
return htmlString;
}
Then from your view,
@Html.CustomValidationSummary(true)
回答2:
@if (ViewContext.ViewData.ModelState.Where(x => x.Key == "").Any())
{
@Html.ValidationSummary(true, null, new { @class = "ui-state-error" })
}
This checks if there are any model wide errors and only renders the summary when there are some.
回答3:
Check this question too.
You could hide the summary with CSS:
.validation-summary-valid { display:none; }
Also, you could put the validation summary before the Html.BeginForm()
.
回答4:
Another way of doing it is to check if there are any li elements, if not just hide validation-summary-errors
<script type="text/javascript">
$(document).ready(function () {
if ($(".validation-summary-errors li:visible").length === 0) {
$(".validation-summary-errors").hide();
}
});
</script>
回答5:
I know a workaround has been found but I had a similar problem with an easier solution.
Is your validation summary rendering with css class "validation-summary-valid" or "validation-summary-errors"? The first class is applied with the validation summary is empty, the second is applied when it's populated.
I've noticed that a placeholder div is rendered if the validation summary contains no errors so client side validation can display it if any fields fail validation.
In the default MVC stylesheet 'Site.css' they just suppress displaying the empty validation summary with 'display:none;'
e.g. .validation-summary-valid { display: none; }
回答6:
Another variation of the fix with Bootstrap classes is:
public static class ValidationSummaryExtensions
{
public static MvcHtmlString CleanValidationSummary(this HtmlHelper htmlHelper, bool excludePropertyErrors, string message = null)
{
if(htmlHelper == null) throw new ArgumentNullException("htmlHelper");
MvcHtmlString validationSummary = null;
if (htmlHelper.ViewData.ModelState.ContainsKey(string.Empty))
{
var htmlAttributes = new { @class = "alert alert-danger" };
validationSummary = htmlHelper.ValidationSummary(excludePropertyErrors, message, htmlAttributes);
}
return validationSummary;
}
}
回答7:
I was having this empty validation summary issue. I just set excludePropertyErrors
to false - and it added the errors to the validation summary.
@Html.ValidationSummary(false)
I realise this isn't necessarily what is being asked here - although this does solve the empty validation summary issue - and is an option if you're having this problem.
回答8:
@if (ViewContext.ViewData.ModelState.Count > 0)
{
//Your content
}
Would work like charm.
回答9:
It's the validation scripts doing this.
Change the following the web.config
<add key="ClientValidationEnabled" value="false" />
It should be false