I have a dom element that contains the string or a url that I would like to visit. I have labelled the dom element with a data attribute for easy reference.
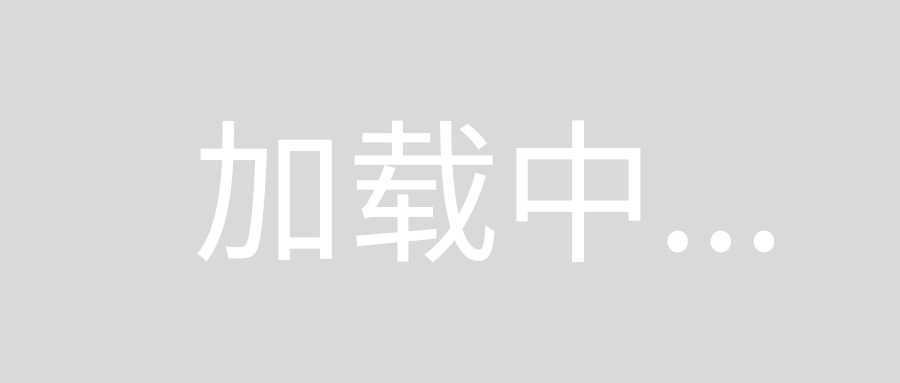
Above where it says 'Create Topic' in bold is the string and in the console, you can see it has a data-test='topicUrl
attribute.
I want to capture this string value so that I can visit the url a a later point.
I followed the docs on Variables and Aliases and tried
cy.get('[data-test="topicUrl"]').invoke('text').as('Url')
so that I could visit the page by using
cy.visit(this.Url)
But that doesn't work, it errors out with TypeError: Cannot read property 'Url' of undefined
in the console.
How do I grab the text in a DOM element so that I can use it to visit a url at a later point?
You can cache it to a variable and issue the cy.visit
in a callback to ensure the variable is populated:
let url;
cy.get('[data-test="topicUrl"]').invoke('text')
.then( value => {
url = value;
});
cy.then(() => {
return cy.visit(url);
});
Using cy.then()
directly (instead of chaining it off of another command) isn't documented and might be removed in the future when cy.resolve( promise )
is implemented.
Maybe it's better to use cy.wrap().then(() => {})
which should work forever (?).
(going entirely from memory, so lemme know if it's not working and I'll update when I get a chance to run it.)
See this question using-aliases-in-cypress of a few days ago, I don't think the this.Url
syntax works universally. The docs you referenced refer to 'mochas shared context object' and 'aliases are automatically cleaned up between tests'.
This leads me to think that you can only use this.Url
when the alias is set in a beforeEach()
You could try the following
cy.get('[data-test="topicUrl"]').invoke('text').as('Url')
...
cy.get('@Url').then(url => cy.visit(url))
Although, this feels more like a click than a visit as the url looks relative to the current site.
cy.get('[data-test="topicUrl"]').click()
cy.wait(100) // a wait might be needed
or from line 847 of the cypress example_spec.js,
cy.get('[data-test="topicUrl"]').as('Url')
...
cy.get('@Url').click()