I need to implement the following markup:
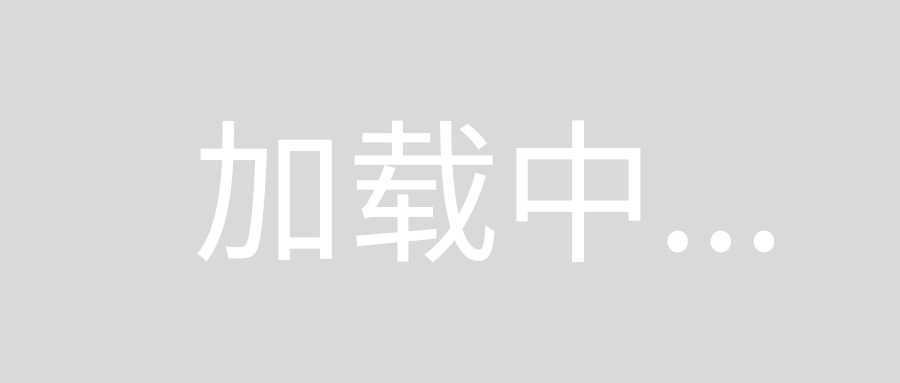
The problem is that I can use only HTML+CSS and XSLT to produce it.
I thought of writing a template that would split the text into lines with XSL and print each line as a different paragraph <p>
with border-bottom
set. Is there a simpler way to achieve this by means of HTML+CSS?
A small update: The point here is to have this underline extend past the text and take all the available width. So all lines are of the same length (like lines in a copybook), except the first one which may be shorter
You can use an inline element such as <span>
which will treat border-bottom
like underline:
<p>
<span>
<del>Lorem ipsum dolor sit amet, consectetur adipisicing elit,</del> sed do
eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam
</span>
</p>
and CSS:
span {
border-bottom: 4px solid black;
}
del {
color: red;
}
Demo here.
Result using the markup above:
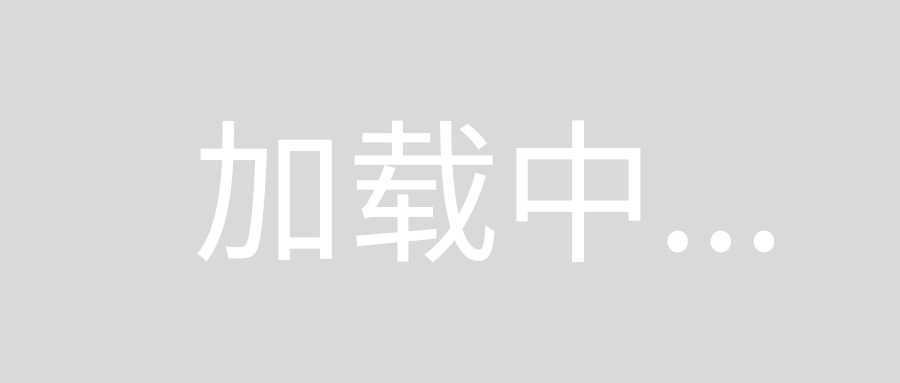
EDIT:
1.
Extending @aefxx's answer, if you can use CSS3 try this:
.strike {
background-image: -moz-linear-gradient(top , rgba(255, 255, 255, 0) 34px, #000000 34px, #000000 38px);
background-image: -webkit-linear-gradient(rgba(255, 255, 255, 0) 34px, #000000 34px, #000000 38px);
background-image: linear-gradient(to bottom, rgba(255, 255, 255, 0) 34px, #000000 34px, #000000 38px);
background-repeat: repeat-y;
background-size: 100% 38px;
}
p {
line-height: 38px;
}
p:before {
background: #fff;
content:"\00a0";
display: inline-block;
height: 38px;
width: 50px;
}
del span {
color: red;
}
Demo here - this will only work in the latest browsers including Firefox and Chrome.
Result in Chrome:
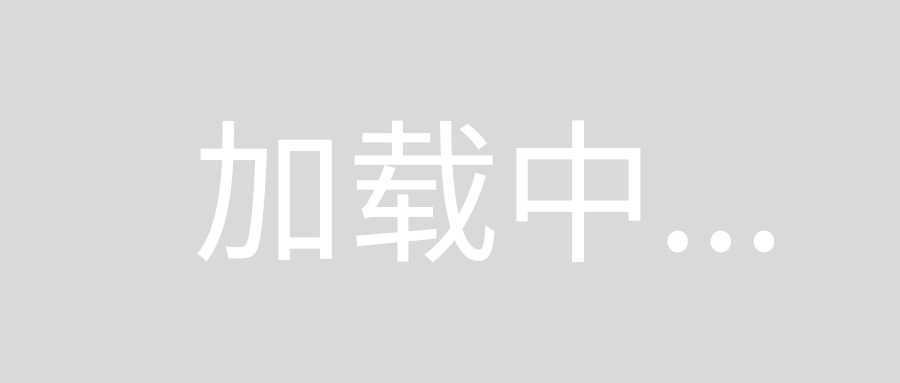
2.
If you're happy with justified text:
p,span {
border-bottom: 4px solid black;
line-height: 30px;
text-align: justify;
text-indent: 50px;
}
p>span {
padding-bottom: 5px;
vertical-align: top;
}
del span {
border-bottom: 0 none;
color: red;
}
Demo here. There are some issues with line-height but should be easy to figure out.
Result in Chrome:
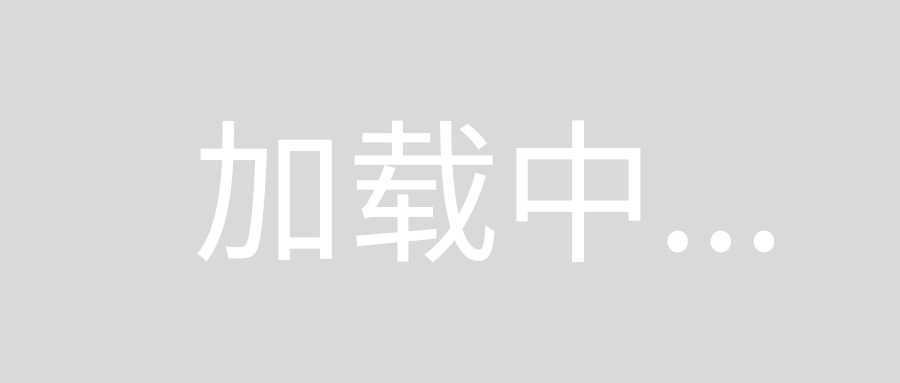
Other than that, I'm afraid you might have to wrap your lines in some containers.
Probably won't get any better than this with pure markup: jsfiddle demo.
EDIT
Update based on the questionaire's comment:
Preview:
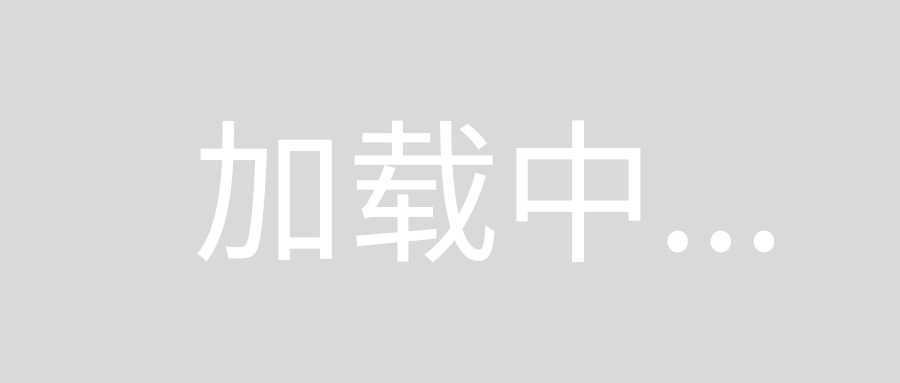
p span.indent {
width: 160px; height: 30px;
vertical-align: top;
background: #fff;
display: inline-block;
}
p span.strike {
color: #000;
text-decoration: line-through;
}
p del {
color: #ff0000;
text-decoration: none;
}
p {
width: 490px;
font-family: Arial, sans-serif;
line-height: 30px;
background: url('http://img84.imageshack.us/img84/1889/63051094.gif') left top;
}
<p>
<span class="indent"></span><span class="strike"><del>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod</del></span> tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation
</p>
Make a div around the content for which you want that formatting and use that div inside a CSS to make necesssary formatting.
HTML
<div id="content">
<p><span id="strike">some content that you need to display</span>
<span id="underline">Some more content that will come here</span></p>
</div>
CSS
#content
{
height:auto;
width:200px;
}
#content #strike
{
text-decoration:line-through;
color:Red;
}
#content #underline
{
text-decoration:underline;
}
span
{
border-bottom:4px solid Black;
}
Live Sample
You don’t actually need each line to be in its own HTML element to have a bottom border. You can apply borders to inline elements, and the border will be applied to each line in the element.
In order to make the strike-through colour be different from the text colour, you need an additional HTML element in there as well. (Although Firefox has implemented -moz-text-decoration-color
.)
HTML
<span class="fake-underline">
<del><span>Final blitz by Obama and Romney as election arrives.</span></del> Candidates attend rallies late into the night as US prepares to go to the polls.
</span>
CSS
.fake-underline {
line-height: 2;
border-bottom: solid 5px black;
}
del {
text-decoration: line-through;
}
del span {
color: red;
}
- http://jsfiddle.net/g5bRc/