I want to enclose a matInput of angular material within a component to reuse it in other places of my application because I need to manage its internal state to change the input type from text to password and viceversa.
I managed to do it by implementing ControlValueAccessor but the styles of validation errors are not being displayed.
Password field component:
export class PasswordFieldComponent
implements OnInit, ControlValueAccessor {
@ViewChild(DefaultValueAccessor) private valueAccessor: DefaultValueAccessor;
@Input() customClass: string;
@Input() customPlaceholder: string;
@Input() required = true;
hide = true;
constructor() { }
ngOnInit() {
}
private propagateChange = (_: any) => { };
private onChange(event) {
this.propagateChange(event.target.value);
}
private onTouch() { }
registerOnChange(fn: any): void {
this.valueAccessor.registerOnChange(fn);
}
registerOnTouched(fn: any): void {
this.valueAccessor.registerOnTouched(fn);
}
setDisabledState(isDisabled: boolean): void {
this.valueAccessor.setDisabledState(isDisabled);
}
writeValue(value: any): void {
this.valueAccessor.writeValue(value);
}
}
Password field template:
<mat-form-field class="full-width {{ customClass }}">
<input
matInput
ngDefaultControl
placeholder="{{ customPlaceholder }}"
[required]="required"
[type]="hide ? 'password' : 'text'"
(input)="onChange($event)">
<button mat-icon-button matSuffix (click)="hide = !hide" [attr.aria-label]="'Hide password'" [attr.aria-pressed]="hide">
<mat-icon>{{hide ? 'visibility_off' : 'visibility'}}</mat-icon>
</button>
</mat-form-field>
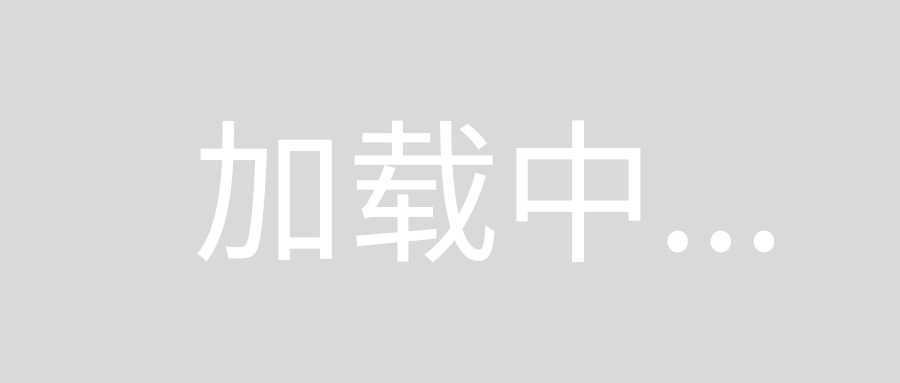
The code from my comments is make the "most simple custom form control that has a material input inside". The idea is create custom ErrorStateMatcher that ask about the control itself. So, out inner material input show errors not when it was invalid else when our custom control was invalid
This ErrorStateMatcher need the know about our control, so we are going to create a constructor to inject this control (I inject in constructor another object "errors" to alow make "invalid" the material input)
class CustomFieldErrorMatcher implements ErrorStateMatcher {
constructor(private customControl: FormControl,private errors:any) { }
isErrorState(control: FormControl | null, form: FormGroupDirective | NgForm | null): boolean {
return this.customControl && this.customControl.touched &&(this.customControl.invalid || this.errors);
}
}
The .html is like
<mat-form-field>
<input #input="ngModel" [ngModel]="value" (ngModelChange)="value=$event;onChange($event)"
matInput
[errorStateMatcher]="errorMatcher()"
[placeholder]="placeholder"
[type]="hide ? 'password' : 'text'"
(blur)="onTouched()"
>
<button mat-icon-button matSuffix (click)="hide = !hide" [attr.aria-label]="'Hide password'" [attr.aria-pressed]="hide">
<mat-icon>{{hide ? 'visibility_off' : 'visibility'}}</mat-icon>
</button>
<mat-error *ngIf="control?.errors?.required">
Please enter a {{placeholder}}
</mat-error>
<mat-error *ngIf="errors?.errorMatch">
Must match
</mat-error>
</mat-form-field>
The most important part is this
[errorStateMatcher]="errorMatcher()"
See that use [ngModel] and (ngModel), (blur) mark the custom formControl "touched". I add a mat-error *ngIf="errors?.errorMatch. This is a @Input()
that get the value of error of Form. This is because we are make a FormGroup that has an custom error if the two fields "password" and "repeatpassword" not match.
Our custom form control is like
export class CustomSelectComponent implements AfterViewInit, ControlValueAccessor {
control: FormControl
onChange: any = () => { };
onTouched: any = () => { };
value: any;
@Input() disabled: boolean;
@Input() placeholder = '';
@Input() errors:any=null;
errorMatcher() {
return new CustomFieldErrorMatcher(this.control,this.errors)
}
constructor(public injector: Injector) {
}
ngAfterViewInit(): void {
const ngControl: NgControl = this.injector.get(NgControl, null);
if (ngControl) {
setTimeout(() => {
this.control = ngControl.control as FormControl;
})
}
}
See how get the ngControl in the ngAfterViewInit, how errorMatcher() return a new CustomFieldErrorMatcher and how pass the values of "control" and "errors".
Well our app.component is like
ngOnInit() {
this.myForm = new FormGroup(
{
password: new FormControl("", Validators.required),
repeatpassword: new FormControl("", Validators.required)
},
this.matchControls("password", "repeatpassword")
);
}
matchControls(field1, field2) {
return (group: FormGroup) => {
const control1 = group.get(field1);
const control2 = group.get(field2);
return control1 && control2 &&
control1.value && control2.value &&
control1.value != control2.value
? { errorMatch: "must match" }: null;
};
}
The .html of the app.component is
<form [formGroup]="myForm" autocomplete="off">
<app-custom-input placeholder="Password" formControlName="password" >
</app-custom-input>
<app-custom-input placeholder="Repeat password" formControlName="repeatpassword" [errors]="myForm.errors?.errorMatch?myForm.errors:null" >
</app-custom-input>
</form>
The stackblitz
added this listener on the custom component. You can also do it 'blur' event.
https://stackoverflow.com/a/59086644/12425844
@HostListener('focusout', ['$event.target'])
onFocusout() {
this.onTouched();
}
And also calling onTouched when setting any value.
writeValue(value: any) {
this.onTouched();
this.Value = value ? value : '';
}