I am trying to click on dropdown value to select city in from field in Make my trip http://www.makemytrip.com/. But getting Stale element reference exception. Ids are getting changed on page load.
Tried below code:
driver.findElement(By.xpath(".//*[@id='hp-widget__sfrom']")).clear();
driver.findElement(By.xpath(".//*[@id='ui-id-1']"));
driver.findElement(By.xpath(".//*[@id='hp-widget__sfrom']")).click();
WebDriverWait wait = new WebDriverWait(driver, 30);
wait.until(ExpectedConditions.elementToBeSelected(driver.findElement(By.xpath(".//*[@class='ui-menu-item'][2]"))));
To click on a dropdown value e.g. Mumbai you can use the following solution:
Code Block:
driver.get("https://www.makemytrip.com/")
new WebDriverWait(driver, 20).until(ExpectedConditions.elementToBeClickable(By.xpath("//input[@class='input_fromto checkSpecialCharacters ui-autocomplete-input' and @id='hp-widget__sfrom']"))).click();
List<WebElement> myList = new WebDriverWait(driver, 20).until(ExpectedConditions.visibilityOfAllElementsLocatedBy(By.xpath("//li[@class='ui-menu-item'][starts-with(@id,'ui-id-')]//span[@class='autoCompleteItem__label']")));
for (WebElement element:myList)
if(element.getText().contains("Mumbai"));
element.click();
Browser Snapshot:
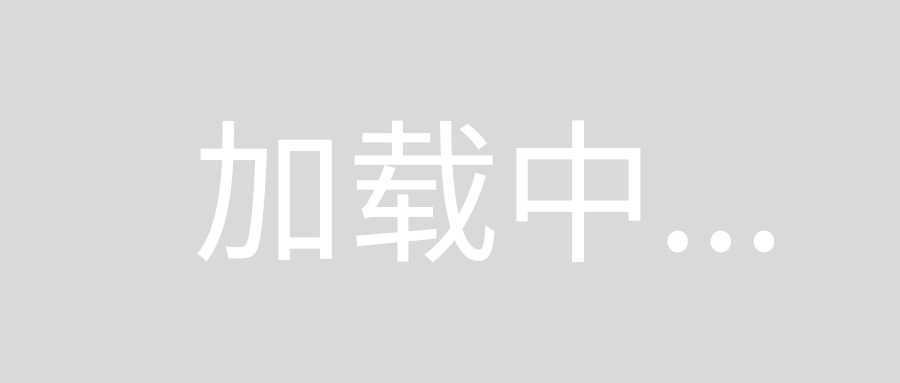
You can use this working code:
WebDriver driver = new ChromeDriver();
driver.get("https://www.makemytrip.com/");
driver.findElement(By.xpath(".//*[@id='hp-widget__sfrom']")).clear();
driver.findElement(By.xpath(".//*[@id='hp-widget__sfrom']")).click();
WebDriverWait wait = new WebDriverWait(driver, 30);
wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//*[@class='ui-menu-item'][2]/div/p[1]/span[1]"))).click();
I have fixed the xPath of dropdown list element. Always try to specify the exact element yo want to interact with. For example if you want to click on button, try to find <span>
or <button>
tag, for a link <a>
tag and for input fields <input>
tag.
You can try this code :
I do not see any use of xpath in this scenario. I have converted some of the xpath to either css selector or id. and have kept only one. Though I have not faced any stale element reference.
System.setProperty("webdriver.chrome.driver", "D:\\Automation\\chromedriver.exe");
driver = new ChromeDriver();
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver, 30);
driver.get("https://www.makemytrip.com/");
WebElement from = wait.until(ExpectedConditions.elementToBeClickable(By.id("hp-widget__sfrom")));
from.click();
from.clear();
wait.until(ExpectedConditions.visibilityOfElementLocated(By.cssSelector("ul[class*='ui-widget-content hp-widget__sfrom']")));
wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//li[contains(@aria-label,'Top Cities : Mumbai, India ')]"))).click();
The below code works fine for me and it is parameterized as well, it works for any input value without changing the xpath. In this example, I took mumbai as test data.
driver.get("https://www.makemytrip.com/");
driver.manage().timeouts().pageLoadTimeout(10, TimeUnit.SECONDS);
driver.findElement(By.xpath("//input[contains(@id,'hp-widget__sfrom')]")).clear();
driver.findElement(By.xpath("//input[contains(@id,'hp-widget__sfrom')]")).click();
driver.findElement(By.xpath("//input[contains(@id,'hp-widget__sfrom')]")).sendKeys("Mumbai");
Thread.sleep(2000);
WebDriverWait wait = new WebDriverWait(driver, 30);
By option = By.xpath("//div[@class='autoCompleteItem']/p/span[contains(text(),'Mumbai')]");
wait.until(ExpectedConditions.elementToBeClickable(option));
driver.findElement(option).click();