I want to use "swipe to delete" option in my project.
-(void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
if (editingStyle == UITableViewCellEditingStyleDelete)
{
NSDictionary *userData = [_contactsArray objectAtIndex:indexPath.row];
NSLog(@"delete row %@",userData);
}
}
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath
{
return YES;
}
I am using this code but it gives following output which I don't want.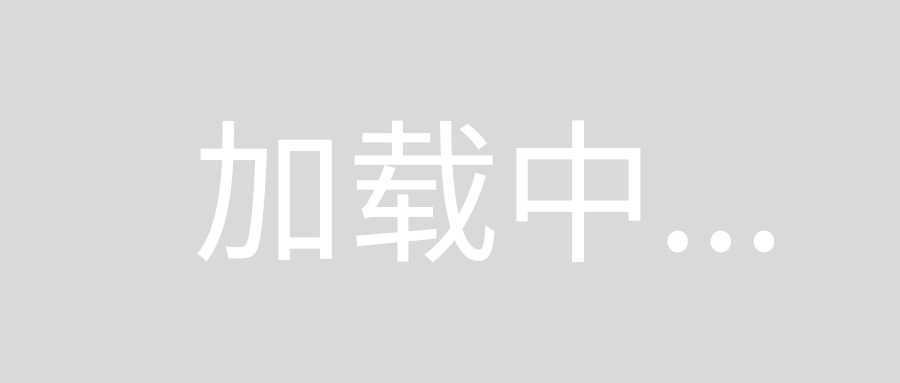
I don't want that left side minus sign on cell.
I just want swipe and show delete button.
The same code I have used in my previous project and it works fine (i.e only swipe to show delete button, no minus sign on left side)
Please help me to solve this.
You are overriding the correct delegate methods for 'swipe to delete' functionality. Regarding the minus signs:
Hide minus sign like this:
self.yourTableView.editing = NO;
//or simply remove this line of code altogether because this property is NO by default
Show minus sign like this:
self.yourTableView.editing = YES;
First create your table view and set delegate and datasource method to that.
self.contacts=[[UITable alloc]init];
self.contacts.dataSource=self;
self.contacts.delegate=self;
self.contacts.userInteractionEnabled=YES;
//self.contacts.editing = YES;
[self.view addSubview:self.contacts];
And then Override the Delegate and data source methods, for delete override the following methods.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath {
// Return YES if you want the specified item to be editable.
return YES;
}
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath {
if (editingStyle == UITableViewCellEditingStyleDelete) {
//add code here for when you hit delete
}
}
For minus sign on left side use self.contacts.editing = YES; In my case I don't want this minus sign so simply don't use that property or set self.contacts.editing = NO;
Thank you hw731 for reminding me this property, I have wasted my half day on this.
Try this:
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath {
// Return YES if you want the specified item to be editable.
return YES;
}
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath {
if (editingStyle == UITableViewCellEditingStyleDelete) {
//add code here for when you hit delete
}
}
Sounds like you are turning the tableView.editing
flag on so the rows show up with the minus sign. try searching for self.tableView.editing = YES;
and make it NO
or simply comment that line out.