I have implemented a UICollectionView, the cell size is w90 h90. When i run it on iPhone 6 i get the proper spacing between cell but when i do it on iPhone 5s i get more spacing between cell. My question is how do i change cell size based on the device screen size, suppose if the cell size was w80 h80 i get proper results on iPhone 5s too. what I'm presently doing is
override func viewWillAppear(animated: Bool) {
var scale = UIScreen.mainScreen().scale as CGFloat
println("Scale :\(scale)")
var cellSize = (self.collectionViewLayout as UICollectionViewFlowLayout).itemSize
println("Cell size :\(cellSize)")
imageSize = CGSizeMake(cellSize.width * scale , cellSize.height * scale)
println("Image size : \(imageSize)")
}
// sizeForItemAtIndexPath
func collectionView(collectionView: UICollectionView,layout collectionViewLayout: UICollectionViewLayout,sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize
{
return imageSize!
}
Result on iPhone 6 :
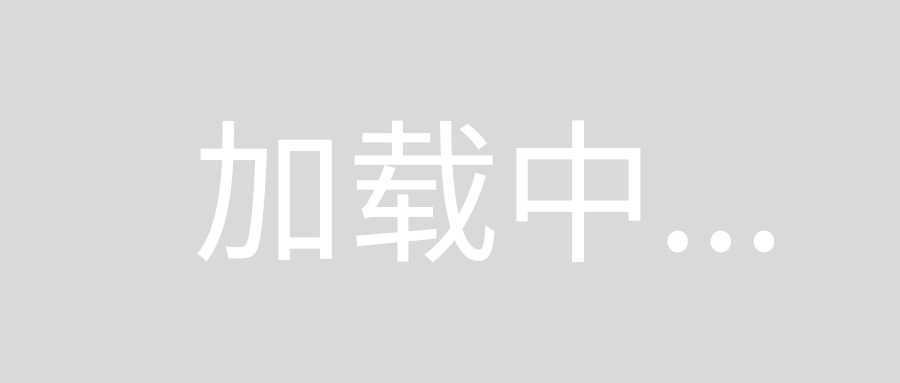
Result on iPhone 5s
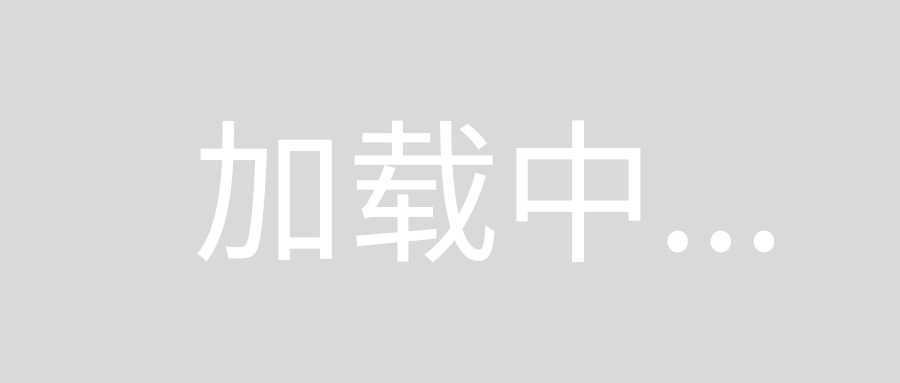
Objective-C / Swift both fine for solution.
Thanks.
Step 1: Implement UICollectionViewDelegateFlowLayout(if not done).
Step 2: Use delegate method of UICollectionViewDelegateFlowLayout.
func collectionView(collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize
{
return CGSizeMake((UIScreen.mainScreen().bounds.width-15)/4,120); //use height whatever you wants.
}
Step 3: Go to XIB or StoryBoard where you have your CollectionView.
Step 4: In XIB or StoryBoard where you have your CollectionView click on CollectionView.
Step 5: Go to InterfaceBuilder, then in second last tab (ie: Size Inspector) set Min Spacing
For Cells = 5
For Lines = 5
That it.
Note:
- This solution is for if you wants to make spacing between cell = 5.
- If your spacing is different then 5, then you need to change value 15 in delegate method.
- Ex: Spacing between cell = 10, then change delegate 15 to 10x3 = 30
return CGSizeMake((UIScreen.mainScreen().bounds.width-30)/4,120);
For Cells = 10
For Lines = 10
and vice versa.
Swift 3 Version
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
let width = UIScreen.main.bounds.width
return CGSize(width: (width - 10)/3, height: (width - 10)/3) // width & height are the same to make a square cell
}
For a slightly more flexible answer
Allows you to adjust number of columns and spacing, expanding on Zaid Pathan's answer
// MARK: UICollectionViewDelegateFlowLayout delegate method
func collectionView(collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize
{
let cellSpacing = CGFloat(2) //Define the space between each cell
let leftRightMargin = CGFloat(20) //If defined in Interface Builder for "Section Insets"
let numColumns = CGFloat(3) //The total number of columns you want
let totalCellSpace = cellSpacing * (numColumns - 1)
let screenWidth = UIScreen.mainScreen().bounds.width
let width = (screenWidth - leftRightMargin - totalCellSpace) / numColumns
let height = CGFloat(110) //whatever height you want
return CGSizeMake(width, height);
}
I wanted 3 item in a section in Both IPad and iPhone. I worked out using the following code. Hope it helps!
1 ) Implement UICollectionViewDelegateFlowLayout delegate
2 ) var device = UIDevice.currentDevice().model
func collectionView(collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize
{
if (device == "iPad" || device == "iPad Simulator")
{
return CGSizeMake((UIScreen.mainScreen().bounds.width-15)/3,230)
}
return CGSizeMake((UIScreen.mainScreen().bounds.width-10)/3,120)
}
Objective-c
example:
use this function
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout *)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath {
return CGSizeMake(Your desired width, Your desired height);
// example: return CGSizeMake(self.view.frame.size.width-20, 100.f);
}
To expand on Tim's answer, there is a small caveat relating to partial point widths: Rounding of partial point widths might cause the width to be wider than will allow the number of desired columns. One way to work around this is to truncate the width using trunc():
return CGSizeMake(trunc(width), height)