可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have a PDF file that I have imported in as a resource into my project. The file is a help document so I want to be able to include it with every deployment. I want to be able to open this file at the click of a button.
I have set the build action to "Embedd Resource". So now I want to be able to open it. However, When I try accessing the resource - My.Resources.HelpFile
- it is a byte array. How would I go about opening this if I know that the end-user has a program suitable to opening PDF documents?
If I missed a previous question please point me to the right direction. I have found several questions about opening a PDF within an application, but I don't care if Adobe Reader opens seperately.
回答1:
Create a new Process:
string path = Path.Combine(Directory.GetCurrentDirectory(), "PDF-FILE.pdf");
Process P = new Process {
StartInfo = {FileName = "AcroRd32.exe", Arguments = path}
};
P.Start();
In order for this to work, the Visual Studio setting Copy to Output Directory
has to be set to Copy Always
for the PDF file.
回答2:
Check this out easy to open pdf file from resource.
private void btnHelp_Click(object sender, EventArgs e)
{
String openPDFFile = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments) + @"\HelpDoc.pdf";//PDF DOc name
System.IO.File.WriteAllBytes(openPDFFile, global::ProjectName.Properties.Resources.resourcePdfFileName);//the resource automatically creates
System.Diagnostics.Process.Start(openPDFFile);
}
回答3:
If the only point of the PDF is to be opened by a PDF reader, don't embed it as a resource. Instead, have your installation copy it to a reasonable place (you could put it where the EXE is located), and run it from there. No point in copying it over and over again.
回答4:
"ReferenceGuide" is the name of the pdf file that i added to my resources.
using System.IO;
using System.Diagnostics;
private void OpenPdfButtonClick(object sender, EventArgs e)
{
//Convert The resource Data into Byte[]
byte[] PDF = Properties.Resources.ReferenceGuide;
MemoryStream ms = new MemoryStream(PDF);
//Create PDF File From Binary of resources folders helpFile.pdf
FileStream f = new FileStream("helpFile.pdf", FileMode.OpenOrCreate);
//Write Bytes into Our Created helpFile.pdf
ms.WriteTo(f);
f.Close();
ms.Close();
// Finally Show the Created PDF from resources
Process.Start("helpFile.pdf");
}
回答5:
File.Create("temp path");
File.WriteAllBytes("temp path", Resource.PDFFile)
回答6:
You need to convert the resource into format acceptable for the program supposed to consume your file.
One way of doing this is to write the content of the resource to a file (a temp file) and then launch the program pointing it to the file.
Whether it is possible to feed the resource directly into the program depends on the program. I am not sure if it can be done with the Adobe Reader.
To write the resource content to a file you can create an instance of the MemoryStream class and pass your byte array to its constructor
回答7:
This should help - I use this code frequently to open various executable, documents, etc... which I have embedded as a resource.
private void button1_Click(object sender, EventArgs e)
{
string openPDFfile = @"c:\temp\pdfName.pdf";
ExtractResource("WindowsFormsApplication1.pdfName.pdf", openPDFfile);
Process.Start(openPDFfile);
}
void ExtractResource( string resource, string path )
{
Stream stream = GetType().Assembly.GetManifestResourceStream( resource );
byte[] bytes = new byte[(int)stream.Length];
stream.Read( bytes, 0, bytes.Length );
File.WriteAllBytes( path, bytes );
}
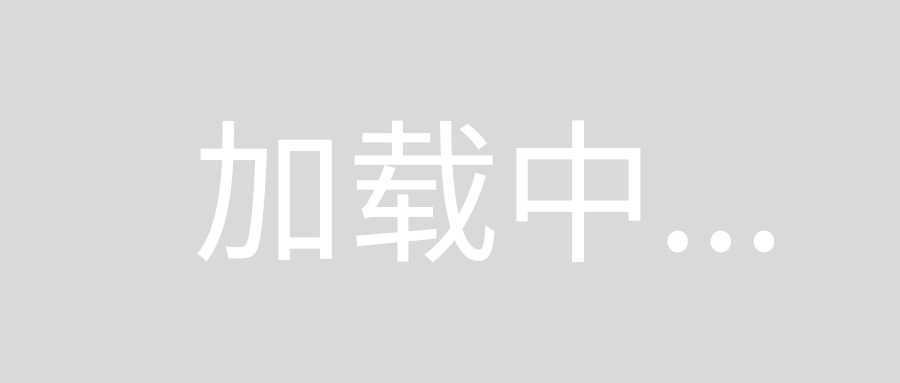