I have a Razor helper function that creates a re-usable HTML panel that saves me writing the same HTML over and over again.
@helper DefaultPanel(string panelTitle) {
<div class="panel">
<div class="panel-logo"><img src="/logo.png"></div>
<div class=panel-inner">
<p class="panel-title">@panelTitle</p>
<div class="panel-content">
/* Can I pass content to be rendered in here here? */
</div>
</div>
</div>
</div>
}
I'm wondering, is it possible to re-use this helper to fill .panel-content
with more HTML to allow further flexibility and code reuse - similar to something like below:
@LayoutHelpers.DefaultPanel("Welcome back") {
<div class="panel-content-inner">
<p>Welcome back, please select from the following options</p>
<a href="#">Profile</a>
<a href="#">My Defails</a>
</div>
}
Whilst using .NET MVC I've noticed the Html.BeginForm()
does a similar thing when wrapping the code within the @using
statement within the Html.BeginForm
declaration, like so:
@using (Html.BeginForm("Index", "Login", FormMethod.Post))
{
<div>This content gets rendered within the <form></form> markup.</div>
}
But can this done using @helper
methods? If not, is it possible to create a HtmlHelper
extension to do a similar thing the way the Html.BeginForm()
method does?
You can do a very similar thing using the @section
syntax as seen here
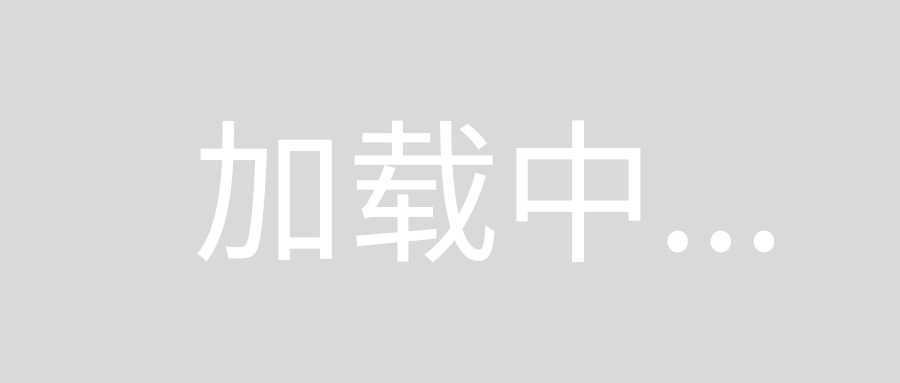
This seems like something that would be really useful to be able to do, and odd that there's no easy way to do it on a component level.
There are two ways to achieve the required functionality.
1. @helper
Create @helper which accepts whatever parameters you need plus a function (single object parameter, returns object):
@helper DefaultPanel(string panelTitle, Func<object, object> content)
{
<div class="panel">
<div class="panel-logo">
<img src="/logo.png" />
</div>
<div class="panel-inner">
<p class="panel-title">@panelTitle</p>
<div class="panel-content">
@content(null)
</div>
</div>
</div>
}
Usage:
@DefaultPanel("title",
@<div class="panel-content-inner">
<p>Welcome back, please select from the following options</p>
<a href="#">Profile</a>
<a href="#">My Defails</a>
</div>
)
Your function may also accepts parameters, example here.
2. HtmlHelper extension method
Add the following code anywhere in your project:
namespace System.Web.Mvc
{
public static class HtmlHelperExtensions
{
public static HtmlDefaultPanel DefaultPanel(this HtmlHelper html, string title)
{
html.ViewContext.Writer.Write(
"<div class=\"panel\">" +
"<div class=\"panel-inner\">" +
"<p class=\"panel-title\">" + title + "</p>" +
"<div class=\"panel-content\">"
);
return new HtmlDefaultPanel(html.ViewContext);
}
}
public class HtmlDefaultPanel : IDisposable
{
private readonly ViewContext _viewContext;
public HtmlDefaultPanel(ViewContext viewContext)
{
_viewContext = viewContext;
}
public void Dispose()
{
_viewContext.Writer.Write(
"</div>" +
"</div>" +
"</div>"
);
}
}
}
Usage:
@using (Html.DefaultPanel("title2"))
{
<div class="panel-content-inner">
<p>Welcome back, please select from the following options</p>
<a href="#">Profile</a>
<a href="#">My Defails</a>
</div>
}
The extension method writes directly to the context. The trick is to return a disposable object, which Dispose method will be executed at the end of using
block.
I don't know if @helper
methods can do this but HtmlHelper
extensions certainly can. You've mentioned the Html.BeginForm()
example which is probably the most well known - all that does is return an object which implements IDisposable
which means that when the Dispose()
method is called it just calls the complimentary Html.EndForm()
method to add the appropriate closing tags.
It would be very simple to do something similar for your HTML code. You can view the source code to the ASP.NET MVC HtmlHelpers at http://aspnetwebstack.codeplex.com/ - the BeginForm()
code can be specifically be viewed here.