可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am trying to create fixed position div inside relative container. I am using bootstrap css framework. I am trying to create a fixed position cart. So whenever user scroll page it will show cart contents. but now problem is, it ran outside that container div.
This has to work in responsive mode.
Here my try:
<div class="wrapper">
<div class="container">
<div class="element">
fixed
</div>
</div>
</div>
CSS Code:
.wrapper {
width:100%
}
.container {
width:300px;
margin:0 auto;
height:500px;
background:#ccc;
}
.element {
background:#f2f2f2;
position:fixed;
width:50px;
height:70px;
top:50px;
right:0px;
border:1px solid #d6d6d6;
}
See live example here.
回答1:
Screenshot:
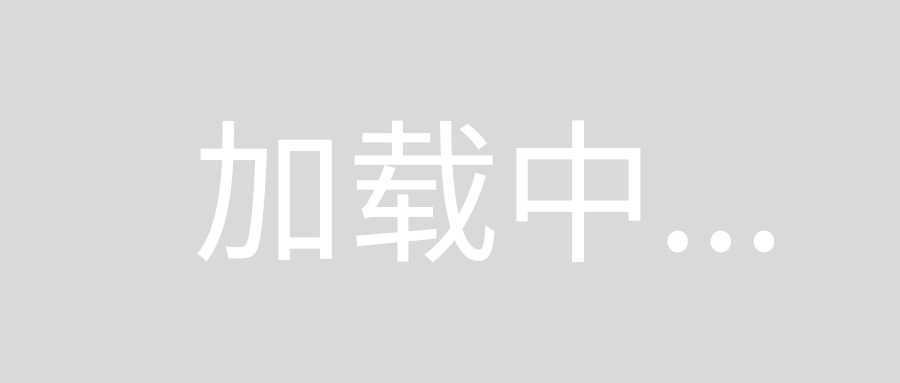
This is how position: fixed;
behaves:
MDN link
Do not leave space for the element. Instead, position it at a
specified position relative to the screen's viewport and doesn't move
when scrolled. When printing, position it at that fixed position on
every page.
Hence, to get what you want you have to use something more than fixed positioning:
Probably this: demo
.wrapper {
width:100%
}
.container {
width:300px;
margin:0 auto;
height:500px;
background:#ccc;
}
.element {
background:#f2f2f2;
position:fixed;
width:50px;
height:70px;
margin-left:250px;
border:0px solid #d6d6d6;
}
回答2:
I found the answer to that :
<div class="container">
<div class="inContainer">
<p> coucou </p>
</div>
<div>
<p> other thing</p>
</div>
</div>
You want that class=inContainer are in class=Container in fixed position but if you scroll with the navigator scroll you don't want that the class=inContainer move outside the class=container
So you can make something like that
.container{
height: 500px;
width: 500px;
overflow-y:scroll;
}
.inContainer {
position: absolute;
}
So class=inContainer will be always on the top of you're class=Container and move with you're class=container if you scroll with navigator scroll =)
(tested only with chrome)
回答3:
No it's impossible because fixed property throws the element out of the flow so it doesn't depend to anything on the document and yes it is no more contained in your container : )
回答4:
Yes, you can do it, just use margin-top
property instead of top
property.
When you use position: fixed and specify a top and or left position,
you'll find that the element will be fixed relative to the window, and
not to any other element of position: relative.
There is a way around this and that is not to specify top and left
positions but instead to use margin-left and margin-top on the
position: fixed element.
Source: https://www.gravitywell.co.uk/latest/design/posts/css-tip-fixed-positioning-inside-a-relative-container/
回答5:
Make the element's parent container have position: relative
Instead of using top
or left
use margin-top
and/or margin-left
If you only use top
that will position the element based on the window, but if you use margin-top
that will position based on the parent element. Same goes for left
or right
<div class="parent">
<div class="child"></div>
</div>
.parent {
position: relative;
}
.child {
position: fixed;
margin-top: 30px;
margin-left: 30px;
}
回答6:
The behavior of the positioning is mentioned in the AdityaSaxena's answer https://stackoverflow.com/a/18285591/5746301
For creating a fixed position cart, you can also do it with using the jquery.
If we apply the Left or right value or margin, we may face some issue while responsive.
In the below snippet, I have placed the fixed element at the right of the container.
Even if the width of the container increased the fixed element placed accordingly.
Here is the jsfiddle Demo URL
//Jquery
$(document).ready(function(){
var containerWidth = $(".container").outerWidth();
var elementWidth = $(".element").outerWidth();
var containerOffsetLeft = $(".container").offset().left;
var containerOffsetRight = containerOffsetLeft + containerWidth - elementWidth;
$(".element").css("left", containerOffsetRight);
});
//CSS
.wrapper {
width:100%
}
.container {
width:300px;
margin:0 auto;
height:900px;
background:#ccc;
}
.element {
background:#f2f2f2;
position:fixed;
width:50px;
height:70px;
top:50px;
border:1px solid #d6d6d6;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="wrapper">
<div class="container">
<div class="element">
fixed
</div>
</div>
</div>
Hope this may help you.
Thanks
回答7:
If you are looking to show the cart even when the user scrolls that is fixed then you should use position:fixed
for the cart (if .container
is your cart), because it should be shown with respect to screen/viewport. Your current code will only show the element
which is positioned absolutely inside the container
. If you want it to be like that then give :
.container {
position:relative;
}
.element {
position:absolute;
top:50px;
right:0px;
}
回答8:
<div style="position: fixed;bottom: 0;width: 100%;">
<div class="container" style="position: relative;">
<div style="position: absolute;right: 40px;bottom: 40px;background:#6cb975;border-radius: 50%;width: 40px;text-align: center;height: 50px;color: #fff;line-height: 50px;">
F
</div>
</div>
</div>
回答9:
You can just add
.element {
left:368px;
}
Fiddle: http://jsfiddle.net/UUgG4/