可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have a UIWebView included in a UIViewController which is a descendant of UINavigationController. It looks like this:
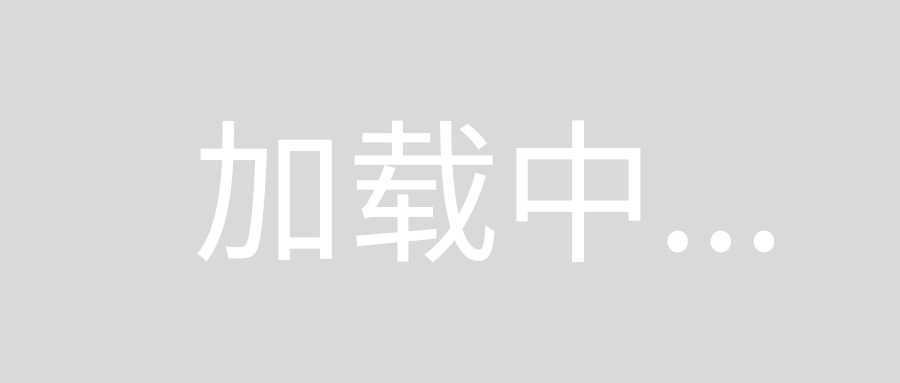
The app is portrait only. When I play the video I want the user to be able to rotate the device and see the video in landscape mode. I use this code to allow it:
- (NSUInteger)application:(UIApplication *)application supportedInterfaceOrientationsForWindow:(UIWindow *)window
{
id presentedViewController = [self topMostController];
NSString *className = presentedViewController ? NSStringFromClass([presentedViewController class]) : nil;
if ([className isEqualToString:@"MPInlineVideoFullscreenViewController"] ||
[className isEqualToString:@"MPMoviePlayerViewController"] ||
[className isEqualToString:@"AVFullScreenViewController"]) {
return UIInterfaceOrientationMaskAllButUpsideDown;
}
return UIInterfaceOrientationMaskPortrait;
}
- (UIViewController *)topMostController {
UIViewController *topController = [UIApplication sharedApplication].keyWindow.rootViewController;
while (topController.presentedViewController) {
topController = topController.presentedViewController;
}
return topController;
}
And then in my UINavigationController (so when the video finishes the view is not presented in landscape but only in portrait):
- (BOOL)shouldAutorotate
{
return NO;
}
- (NSUInteger)supportedInterfaceOrientations
{
return UIInterfaceOrientationMaskPortrait;
}
- (UIInterfaceOrientation)preferredInterfaceOrientationForPresentation
{
return UIInterfaceOrientationPortrait;
}
Everything works perfectly:
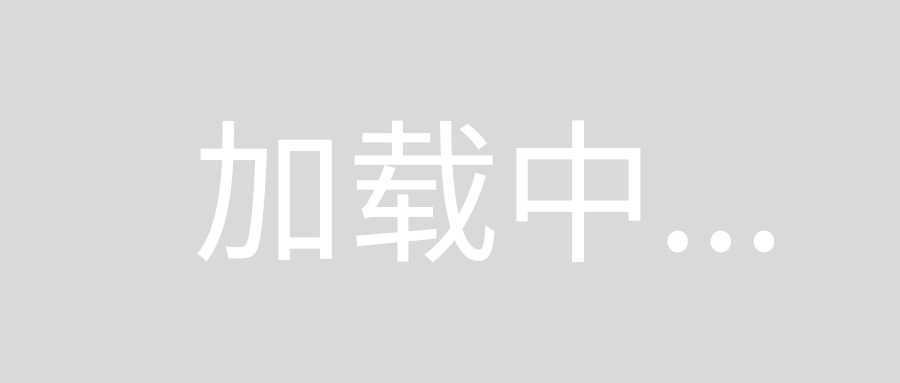
But then the video is done playing (or the user taps ‘Done’) and the screens return to the underlying view, this is what happens:
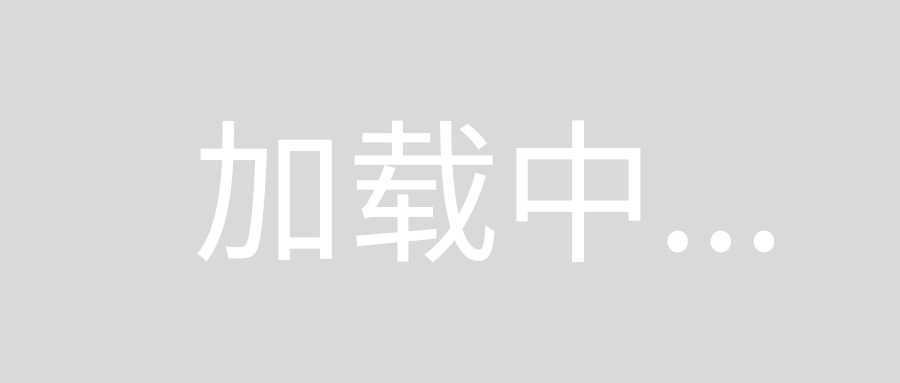
As you can see, the navigation bar slips under the status bar.
Additionally, I get a lot of auto-layout errors in the logs: http://pastebin.com/09xHzmgJ
Any idea about how to solve this?
回答1:
I temporarily solved (through a hack) with the following code in the viewDidLoad
of my controller. I have to specify that the code is specifically made for my case: since I explicitly disallow landscape orientation of my UINavigationController (see code above), the usual notification “UIDeviceOrientationDidChange” is not called when the playback finished and the window goes back to portrait. However, I hope there is a better option and this is a bug of the SDK, since it does not appear on iOS 7 and given the amount of auto-layout errors I get related to the video player (on which I have no control).
- (void)viewDidLoad
{
[super viewDidLoad];
// […]
/*
Hack to fix navigation bar position/height on iOS 8 after closing fullscreen video
Observe for “UIWindowDidRotateNotification” since “UIDeviceOrientationDidChangeNotification” is not called in the present conditions
Check if the notification key (“UIWindowOldOrientationUserInfoKey”) in userInfo is either 3 or 4, which means the old orientation was landscape
If so, correct the frame of the navigation bar to the proper size.
*/
[[NSNotificationCenter defaultCenter] addObserverForName:@"UIWindowDidRotateNotification" object:nil queue:nil usingBlock:^(NSNotification *note) {
if ([note.userInfo[@"UIWindowOldOrientationUserInfoKey"] intValue] >= 3) {
self.navigationController.navigationBar.frame = (CGRect){0, 0, self.view.frame.size.width, 64};
}
}];
}
And then…
- (void)dealloc
{
[[NSNotificationCenter defaultCenter] removeObserver:self forKeyPath:@"UIWindowDidRotateNotification"];
}
回答2:
I encountered exactly the same issue and used the same code as @entropid did. However the accepted solution did not work for me.
It took me hours to come up with the following one-line fix that made things working for me:
- (void)viewWillLayoutSubviews {
[[UIApplication sharedApplication] setStatusBarHidden:NO withAnimation:UIStatusBarAnimationNone];
}
回答3:
I faced this problem yesterday, where @entropid answer worked for iOS 9 and below, but for iOS 10 it didn't (since iOS 10 did actually hide the status bar, where on iOS 9 and below it was just the UINavigationBar
that changed its frame without hiding the status bar and, thus, it overlapped that bar).
Also, subscribing to MPMoviePlayerControllerDidExitFullScreen
notification didn't work either, sometimes it simply wasn't called (in my particular case, it was because it was a video from a UIWebView
, which used a different class of player which looks similar to MPMoviePlayerController
).
So I solved it using a solution like the one @StanislavPankevich suggested, but I subscribed to notifications when a UIWindow
has become hidden (which can be in several cases, like when a video has finished, but also when a UIActivityViewController
dismisses and other cases) instead of viewWillLayoutSubviews
. For my particular case (a subclass of UINavigationController
), methods like viewDidAppear
, viewWillAppear
, etc. simply weren't being called.
viewDidLoad
- (void)viewDidLoad {
[super viewDidLoad];
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(videoDidExitFullscreen:)
name:UIWindowDidBecomeHiddenNotification
object:nil];
// Some other logic...
}
dealloc
- (void)dealloc {
[[NSNotificationCenter defaultCenter] removeObserver:self];
}
And finally, videoDidExitFullscreen
- (void)videoDidExitFullscreen:(NSNotification *)notification {
// You would want to check here if the window dismissed was actually a video one or not.
[[UIApplication sharedApplication] setStatusBarHidden:NO
withAnimation:UIStatusBarAnimationFade];
}
I used UIStatusBarAnimationFade
because it looks a lot smoother than UIStatusBarAnimationNone
, at least from a user perspective.
回答4:
Swift version:
//AppDelegate:
func application(application: UIApplication, supportedInterfaceOrientationsForWindow window: UIWindow?) -> Int {
var presentedVC = application.keyWindow?.rootViewController
while let pVC = presentedVC?.presentedViewController
{
presentedVC = pVC
}
if let pVC = presentedVC
{
if contains(["MPInlineVideoFullscreenViewController", "MPMoviePlayerViewController", "AVFullScreenViewController"], pVC.nameOfClass)
{
return Int(UIInterfaceOrientationMask.AllButUpsideDown.rawValue)
}
}
return Int(UIInterfaceOrientationMask.Portrait.rawValue)
}
//Extension:
public extension NSObject{
public class var nameOfClass: String{
return NSStringFromClass(self).componentsSeparatedByString(".").last!
}
public var nameOfClass: String{
return NSStringFromClass(self.dynamicType).componentsSeparatedByString(".").last!
}
}
//View controller:
override func supportedInterfaceOrientations() -> Int {
return Int(UIInterfaceOrientationMask.Portrait.rawValue)
}
override func preferredInterfaceOrientationForPresentation() -> UIInterfaceOrientation {
return UIInterfaceOrientation.Portrait
}
override func shouldAutorotate() -> Bool {
return false
}
override func viewWillLayoutSubviews() {
UIApplication.sharedApplication().setStatusBarHidden(false, withAnimation: UIStatusBarAnimation.None)
}
回答5:
It's very simple, as it says @Stanislav Pankevich, but in
swift 3 version
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews();
UIApplication.shared.isStatusBarHidden = false
}
回答6:
I was having the same issue and using @entropid solution I was able to fix the navigation bar problem. But my view remains below the nav bar which I fix using "sizeToFit".
[[NSNotificationCenter defaultCenter] addObserverForName:@"UIWindowDidRotateNotification" object:nil queue:nil usingBlock:^(NSNotification *note) {
if ([note.userInfo[@"UIWindowOldOrientationUserInfoKey"] intValue] >= 3) {
[self.navigationController.navigationBar sizeToFit];
self.navigationController.navigationBar.frame = (CGRect){0, 0, self.view.frame.size.width, 64};
}
}];
I haven't tested it properly but its working for me right now
回答7:
On iOS 11 accepted solution didn't work for me. Seems that navigation bar stops reflecting for frame changes. But there is a workaround. At first, we need to modify supportedInterfaceOrientationsForWindow
method to return UIInterfaceOrientationMaskLandscape
for video controllers instead of UIInterfaceOrientationMaskAllButUpsideDown
. In my case when I tap on embedded YouTube video, system always opens AVFullScreenViewController, so I removed other checks from original example. Code:
- (UIInterfaceOrientationMask)application:(UIApplication *)application supportedInterfaceOrientationsForWindow:(UIWindow *)window {
__kindof UIViewController *presentedViewController = [self topMostController];
// Allow rotate videos
NSString *className = presentedViewController ? NSStringFromClass([presentedViewController class]) : nil;
if ([className isEqualToString:@"AVFullScreenViewController"]) {
return UIInterfaceOrientationMaskLandscape;
}
return UIInterfaceOrientationMaskPortrait;
}
This didn't changes behaviour of AVFullScreenViewController on iOS 10 and less, but fixes navigation bar on iOS 11, so there is no need to update frame (also there is a side-effect on iOS 11 that video rotates from landscape when starts playing, but it's a tradeoff). Next, we need to add check in UIWindowDidBecomeHiddenNotification
method:
- (void)videoDidExitFullscreen {
if (@available(iOS 11, *)) {
// Fixes status bar on iPhone X
[self setNeedsStatusBarAppearanceUpdate];
} else {
self.navigationController.navigationBar.frame = CGRectMake(0, 0, self.view.bounds.size.width, statusAndNavBarHeight);
}
}
Without setNeedsStatusBarAppearanceUpdate
text in status bar will not appear on iPhone X, for other devices it's not needed.
回答8:
My answer on this question works great. Here It will play the video inside your WebView normally but if you tilt your phone it will play in Landscape!
Also important to note is if you include youtube.com as the Base URL it will load much quicker.
Make a UIWebView in your storyboard and connect the @property to it, then reference below.
CGFloat width = self.webView.frame.size.height;
CGFloat height = self.webView.frame.size.width;
NSString *youTubeVideoCode = @"dQw4w9WgXcQ";
NSString *embedHTML = @"<iframe width=\"%f\" height=\"%f\" src=\"http://www.youtube.com/embed/%@\" frameborder=\"0\" style=\"margin:-8px;padding:0;\" allowfullscreen></iframe>";
NSString *html = [NSString stringWithFormat:embedHTML, width, height, youTubeVideoCode];
self.webView.scrollView.bounces = NO;
[self.webView loadHTMLString:html baseURL:[NSURL URLWithString:@"http://www.youtube.com"]];