In Visual Studio when debugging C# code, can I export a Dictionary<string,string>
in xml,csv or text format easily?
I would like to export a Dictionary<string,string>
in excel and see 2 columns of strings.
Debugging such dict in VS is cumbersome.
If there s any add on that simplifies visualization that s ok too.
You can add a watch to your Dictionary (or List), then under the Watch Window you can then expand the entire dictionary (or List), right click, select-all, copy
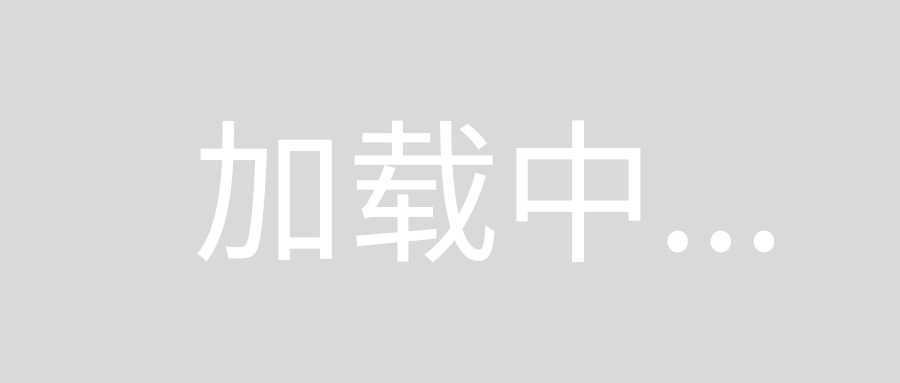
Then in Excel you can paste the data and it should auto-format:
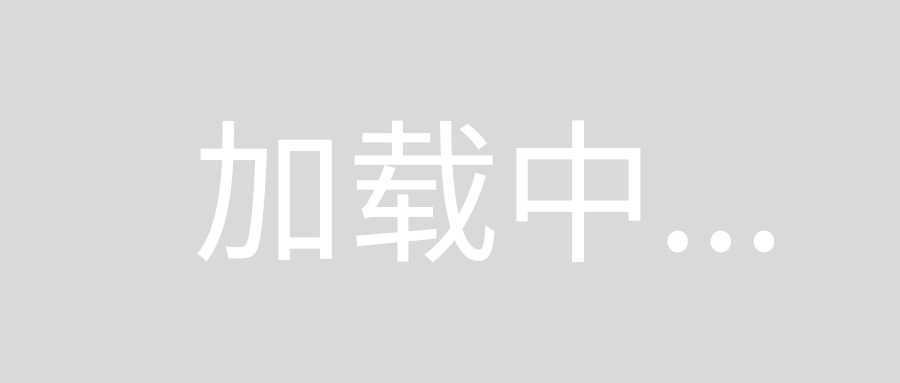
You could also just paste this data directly into another text-editor (or just view the data directly in the watch window too).
Hope that can help.
You could use a simple snippet like this:
var output = dict.Select(kv => string.Format("{0},{1}", kv.Key, kv.Value));
File.WriteAllLines("output.csv", output);
As noted in the comments, if your dictionary keys or values contain any commas or newlines, you'll need something slightly more complex to generate valid a CSV file.
Dictionary<TKey, TValue>
implements IEnumerable<KeyValuePair<TKey, TValue>>
. For debugging purposes, you can convert dictionary to multiline string using LINQ query:
string description =
string.Join(Environment.NewLine,
dict.Select(tuple => $"{tuple.Key} => {tuple.Value}").ToArray());
Maybe this will be enough for debugging.
Use The Immediate Window
Don't underestimate the power of the immediate window for this kind of thing.
My advice is to add an extension method to Dictionary which creates your csv file, then, in the Immediate window, you can call it whenever and wherever you need.
public static class DictionaryExtensions
{
public static string ToCsvFormat<TK,TV>(this IDictionary<TK,TV> dict)
{
var sw = new StringWriter();
foreach(var kv in dict)
{
sw.WriteLine($"{kv.Key}, {kv.Value}");
}
return sw.ToString();
}
}
Here's a C# fiddle of the extension method in action:
https://dotnetfiddle.net/f8xQjs
And to use it in the immediate window:
foo.ToCsvFormat(),nq
the ",nq" option causes it to properly handle multi-lines. Then you can copy/paste from the immediate window and you're good to go.