I'm trying to write a tampermonkey script which gathers the document.location and headers in a dictionary. Googled a bit and figured I was supposed to use a global variable of some sort, but it's not working as I want.
Here's the script:
// ==UserScript==
// @name My Fancy New Userscript
// @namespace http://your.homepage/
// @version 0.1
// @description enter something useful
// @author You
// @match *://*/*
// @grant none
// ==/UserScript==
if (unsafeWindow.resources == undefined) {
var unsafeWindow.resources = [];
}
var host = window.location;
unsafeWindow.resources.push(host);
console.log(unsafeWindow.resources);
When running it I get the following error:
ERROR: Execution of script 'My Fancy New Userscript' failed! unsafeWindow is not defined
Maybe what I'm trying to do is not even possible?
Update:
Trying to be a bit clearer. The end result should result in a dictionary that would have the document.location as key and a dictionary containing the header name and header value of said location as the value.
{document.location = {"Headername" = "Header value", "Headername" = "Header value"}}
The end result will be used to generate a table with the information in the dictionary. Something like this:
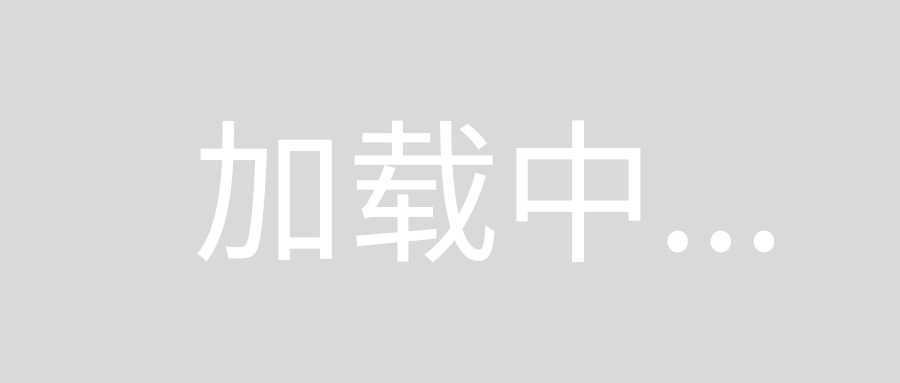
/Patrik
Please take this as a brief EXAMPLE of what you should accomplish, and be aware that you may encounter some issues while moving on with your project, because sometimes document.location can be a bit tricky to retrieve.
That apart, the code:
// ==UserScript==
// @name My Fancy New Userscript
// @namespace http://use.i.E.your.homepage/
// @version 0.1
// @description enter something useful
// @match *://*/*
// @copyright 2015+, You
// ==/UserScript==
var storage = (function(win){
var localDrive = win.localStorage;
return {
save: function (/* <string> */ key, /* <string | JSONstringified object> */ value) {
localDrive.setItem(key, value);
},
destroy: function (/* <string> */ key) {
return localDrive.removeItem(key) ? true : false;
},
get: function (/* <string> */ key) {
return localDrive.getItem(key) == '' || localDrive.getItem(key) == null ? false : localDrive.getItem(key);
}
}
})(window);
window.storage = storage;
document.addEventListener("DOMContentLoaded", function(e) {
// Dom ready, start:
// Check whether the array exists or not :
if (!storage.get("myDataList")) {
storage.save("myDataList", JSON.stringify(
[{
'href' : document.location.href,
'location' : document.location,
'test1' : 'test',
'test2' : 'test2'
}]
)
);
}
else {
// If exists, log every single object:
var currentStorageList = JSON.parse(storage.get("myDataList"));
for (var i = 0; i < currentStorageList.length; ++i) {
console.log(currentStorageList[i]);
}
}
// Check whether this element exists in the current list, else add :
var currentStorageList = JSON.parse(storage.get("myDataList"));
var elementExists = currentStorageList.some(function(el,i,arr) {
return el.href === document.location.href;
});
if (!elementExists) {
console.log("current elements doesn't exist, let's push it!");
storage.save("myDataList", JSON.stringify(JSON.parse(storage.get("myDataList")).push({
'href' : document.location.href,
'location' : document.location,
'test1' : 'test',
'test2' : 'test2'
})));
}
});
This is pure javascript, since I didn't see you using jQuery.
I have provided there:
- A comfortable DOM object (storage) with three main methods: save (stores key => value, where value must be a string or a json stringified element, because you can't store arrays in local storage), get (get from key) and destroy (from key).
- A constructor right at the beginning of domready: if the key holding the elements does NOT exist, it will create it and fill with the current document location, identifying it using the document's href.
- A few examples to work with in order to save / retrieve what you need.
Please note that this is just an example (it does work, though).
Also, in your tampermonkey script settings, don't forget to set it to run at document-end.
The output on xkcd (for testing) is this:
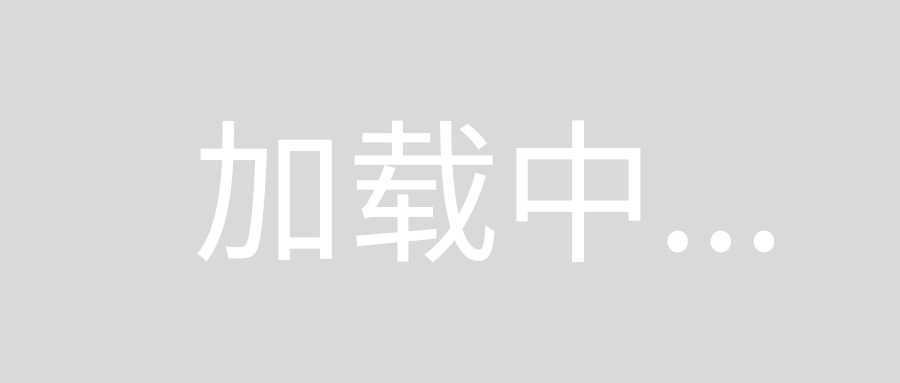
http://prntscr.com/784zw7 (picture direct link)
Hope this is helpful for your project ;)