Want to improve this question? Update the question so it focuses on one problem only by editing this post.
Closed 6 years ago.
i want create reviews for users from users, how better create db and Active Record Associations, i am thinking of create model reviews, reviews table with content string and table users_users with 2 strings user_id, user_id, first string who create review and second for whom created review, or this wrong way?
Although your question is very vague, I'm guessing you're quite new to Rails, so I thought I'd write this post for you
User has_many Reviews
Sounds to me like you need help with ActiveRecord associations
, which will allow you to create Reviews from Users
Specifically, you'd want to use the has_many
relationship, so that a user has_many reviews
:
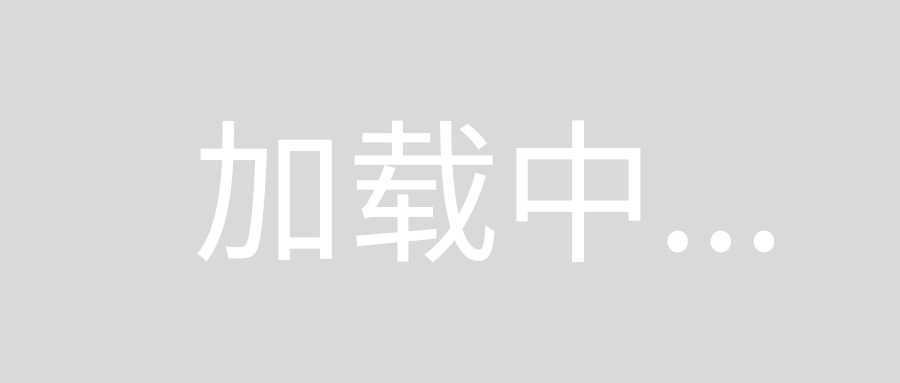
You'd set up 2 models like this:
#app/models/review.rb
class Review < ActiveRecord::Base
belongs_to :user
end
#app/models/user.rb
class User < ActiveRecord::Base
has_many :reviews
end
This will allow you to call the User's ActiveRecord objects like this:
#app/controllers/users_controller.rb
def index
@user = User.find(params[:id])
@reviews = @user.reviews
end
Saving Reviews to Users
Having this setup will allow you to save reviews to specific users
To do this, you'll be able to either use accepts_nested_attributes_for
or in the reviews
controller, you can merge the user_id
of the current user to assign a value to the "foreign key" (user_id
) column ActiveRecord uses in its association
The latter is the most straightforward to explain, so I'll give you a demo:
#app/controllers/reviews_controller.rb
def new
@review = Review.new
end
def create
@review = Review.new(review_params)
@review.save
end
private
def review_params
params.require(:review).permit(:title, :body).merge(:user_id => current_user.id)
end
#app/views/reviews/new.html.erb
<%= form_for @review do |f| %>
<%= f.text_field :title %>
<%= f.text_field :body %>
<% end %>
This will save the review you post with an attached user_id
, which you can then call with something like @user.reviews
Hope this helps?
I think you can limit this to one new model UserReview
, something like:
rails g model UserReview for_user:references by_user:references content:text stars:integer
And the model definition with two scopes:
def UserReview
belongs_to :for_user, class_name: 'User'
belongs_to :by_user, class_name: 'User'
scope :reviews_for, ->(user) { where("for_user = ?", user) }
scope :reviews_by, ->(user) { where("by_user = ?", user) }
end
The scope methods allow for easier querying of reviews like:
user_reviews = UserReview.reviews_for(current_user)