可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I'm trying to align an HTML button exactly at the centre of the page irrespective of the browser used. It is either floating to the left while still being at the vertical centre or being somewhere on the page like at the top of the page etc..
I want it to be both vertically and horizontally be centered. Here is what I have written right now:
<button type="button" style="background-color:yellow;margin-left:auto;margin-right:auto;display:block;margin-top:22%;margin-bottom:0%">
mybuttonname
</button>
回答1:
Here's your solution: http://jsfiddle.net/7Laf8/
Basically, place your button into a div with centred text:
<div class="wrapper">
<button class="button">Button</button>
</div>
With the following styles:
.wrapper {
text-align: center;
}
.button {
position: absolute;
top: 50%;
}
There are many ways to skin a cat, and this is just one.
回答2:
You can center a button without using text-align
on the parent div
by simple using margin:auto; display:block;
For example:
HTML
<div>
<button>Submit</button>
</div>
CSS
button{
margin:auto;
display:block;
}
SEE IT IN ACTION: http://codepen.io/maudulus/pen/avBdpL
回答3:
I've really taken recently to display: table
to give things a fixed size such as to enable margin: 0 auto
to work. Has made my life a lot easier. You just need to get past the fact that 'table' display doesn't mean table html.
It's especially useful for responsive design where things just get hairy and crazy 50% left this and -50% that just become unmanageable.
style
{
display: table;
margin: 0 auto
}
http://jsfiddle.net/Z52eR/
In addition if you've got two buttons and you want them the same width you don't even need to know the size of each to get them to be the same width - because the table will magically collapse them for you.
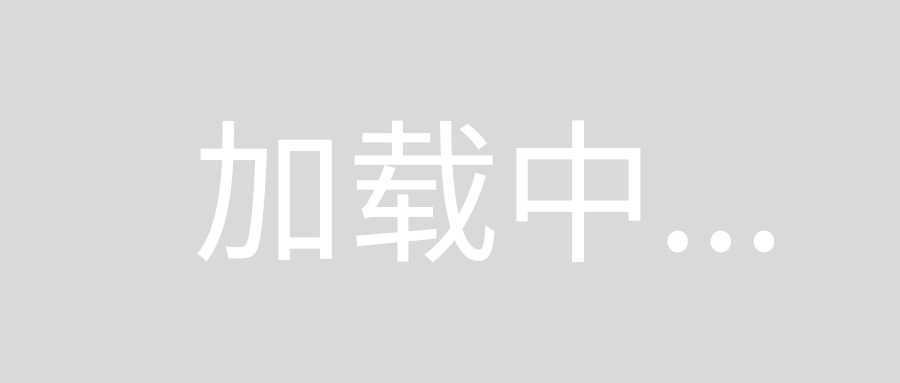
http://jsfiddle.net/AK674/2/
(this also works if they're inline and you want to center two buttons side to side - try doing that with percentages!).
回答4:
try this it is quite simple and give you cant make changes to your .css file this should work
<p align="center">
<button type="button" style="background-color:yellow;margin-left:auto;margin-right:auto;display:block;margin-top:22%;margin-bottom:0%"> mybuttonname</button>
</p>
回答5:
Here is the solution as asked
<button type="button" style="background-color:yellow;margin:auto;display:block">mybuttonname</button>
回答6:
For me it worked using flexbox.
Add a css class around the parent div / element with :
.parent {
display: flex;
}
and for the button use:
.button {
justify-content: center;
}
You should use a parent div, otherwise the button doesn't 'know' what the middle of the page / element is.
回答7:
Place it inside another div, use CSS to move the button to the middle:
<div style="width:100%;height:100%;position:absolute;vertical-align:middle;text-align:center;">
<button type="button" style="background-color:yellow;margin-left:auto;margin-right:auto;display:block;margin-top:22%;margin-bottom:0%">
mybuttonname</button>
</div>
Here is an example: http://jsfiddle.net/U2B3x/
回答8:
There are multiple ways to fix the same. PFB two of them -
1st Way using position: fixed - position: fixed; positions relative to the viewport, which means it always stays in the same place even if the page is scrolled. Adding the left and top value to 50% will place it into the middle of the screen.
button {
position: fixed;
left: 50%;
top:50%;
}
2nd Way using margin: auto -margin: 0 auto; for horizontal centering, but margin: auto; has refused to work for vertical centering… until now! But actually absolute centering only requires a declared height and these styles:
button {
margin: auto;
position: absolute;
top: 0;
left: 0;
bottom: 0;
right: 0;
height: 40px;
}