Selenium fails to locate the iframe by ID
and Name
.
This is for an automated checkout test on Shopify. The specific issue lies within the payment field. I found the ID and name of the iframe
, which is card-fields-number-b1kh6njydiv00000
.
iframe Image:
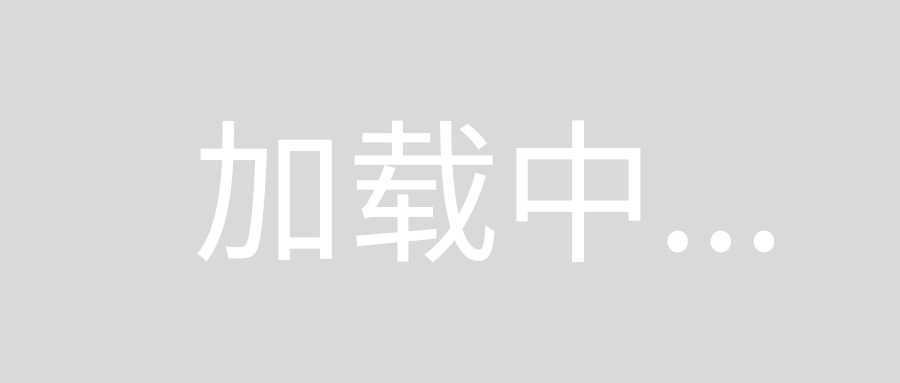
Text Field Image:
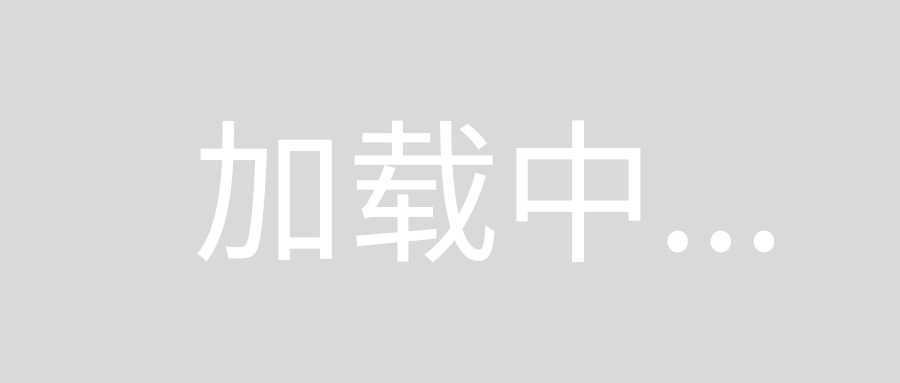
Code trials:
driver.switchTo().frame("card-fields-number-b1kh6njydiv00000");
System.out.println("Found iframe");
The error is:
org.openqa.selenium.NoSuchFrameException: No frame element found by name or id card-fields-number-b1kh6njydiv00000
It is possible to use XPath for this I believe. You will need to find the IFrame IWebElement with XPath, and then pass the IWebElement into the SwitchTo().Frame()
var ele = driver.FindElement(By.XPath("//iframe[contains(id, 'card-fields-number')]"));
driver.switchTo().frame(ele);
Are you tried to use driver.switchTo().defaultContent(); before switchTo.frame ?
Maybe you aren't out of all the frames
driver.switchTo().defaultContent();
driver.switchTo().frame("card-fields-number-b1kh6njydiv00000");
System.out.println("Found iframe");
I guess the frame name or ID is dynamic each time.In that case use index to identify the frame.
int size = driver.findElements(By.tagName("iframe")).size();
for(int i=0; i<=size; i++){
driver.switchTo().frame(i);
//Do necessary operation here.
driver.switchTo().defaultContent();
}
Hope this helps
As per the images you have shared the <iframe>
is having dynamic attributes so to locate and switch to the desired <iframe>
you have to:
Here you can find a relevant discussion on Ways to deal with #document under iframe
My solution was to look for keywords that are the exact same for different dynamic id's. In this case, it was "card-fields-name". I did this by using the XPath locator.
driver.switchTo().frame(driver.findElement(By.xpath("//iframe[contains(@id,'card-fields-number')]")));