In OS X Yosemite, Apple introduced a new class NSVisualEffectView
. Currently, this class is undocumented, but we can use it in Interface Builder.
How can I use NSVisualEffectView
in the window's title bar?
Here's example: in Safari, when you scroll, the content appears under the toolbar and titlebar with a vibrance and blur effect.
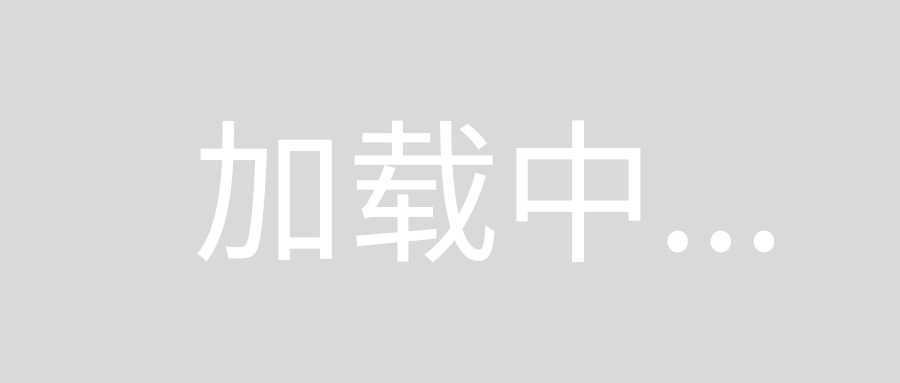
@sgonzalez's answer forced me to explore NSWindow.h
file where i found titlebarAppearsTransparent
property.
So we get:
class BluredWindow: NSWindow {
override func awakeFromNib() {
let visualEffectView = NSVisualEffectView(frame: NSMakeRect(0, 0, 300, 180))
visualEffectView.material = NSVisualEffectView.Material.dark
visualEffectView.blendingMode = NSVisualEffectView.BlendingMode.behindWindow
visualEffectView.state = NSVisualEffectView.State.active
self.styleMask = self.styleMask | NSFullSizeContentViewWindowMask
self.titlebarAppearsTransparent = true
//self.appearance = NSAppearance(named: NSAppearanceNameVibrantDark)
self.contentView.addSubview(visualEffectView)
self.contentView.addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "V:|-0-[visualEffectView]-0-|",
options: NSLayoutConstraint.FormatOptions.directionLeadingToTrailing,
metrics: nil,
views: ["visualEffectView":visualEffectView]))
self.contentView.addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "H:|-0-[visualEffectView]-0-|",
options: NSLayoutConstraint.FormatOptions.directionLeadingToTrailing,
metrics: nil,
views: ["visualEffectView":visualEffectView]))
Also you can setup NSVisualEffectView in IB it will be expanded on titlebar.
You need to modify your window's stylemask to include NSFullSizeContentViewWindowMask
so that its content view can "overflow" into it.
You can easily accomplish this by adding this line to your AppDelegate:
self.window.styleMask = self.window.styleMask | NSFullSizeContentViewWindowMask;
If you want it to appear dark, like in FaceTime, you need to also add this line of code:
self.window.appearance = NSAppearance(named: NSAppearanceNameVibrantDark)
Step by step Tutorial with examples:
http://eon.codes/blog/2016/01/23/Chromeless-window/
Setting up translucency:
- Set the styleMask of your NSWindow subclass to
NSFullSizeContentViewWindowMask
(so that the translucency will also be visible in the titlebar area, leave this out and the titlebar area will be blank)
- Set the
self.titlebarAppearsTransparent = true
(hides the titlebar default graphics)
- Add the code bellow to your NSWindow subclass: (you should now have a translucent window)
.
let visualEffectView = NSVisualEffectView(frame: NSMakeRect(0, 0, 0, 0))//<---the width and height is set to 0, as this doesn't matter.
visualEffectView.material = NSVisualEffectMaterial.AppearanceBased//Dark,MediumLight,PopOver,UltraDark,AppearanceBased,Titlebar,Menu
visualEffectView.blendingMode = NSVisualEffectBlendingMode.BehindWindow//I think if you set this to WithinWindow you get the effect safari has in its TitleBar. It should have an Opaque background behind it or else it will not work well
visualEffectView.state = NSVisualEffectState.Active//FollowsWindowActiveState,Inactive
self.contentView = visualEffectView/*you can also add the visualEffectView to the contentview, just add some width and height to the visualEffectView, you also need to flip the view if you like to work from TopLeft, do this through subclassing*/
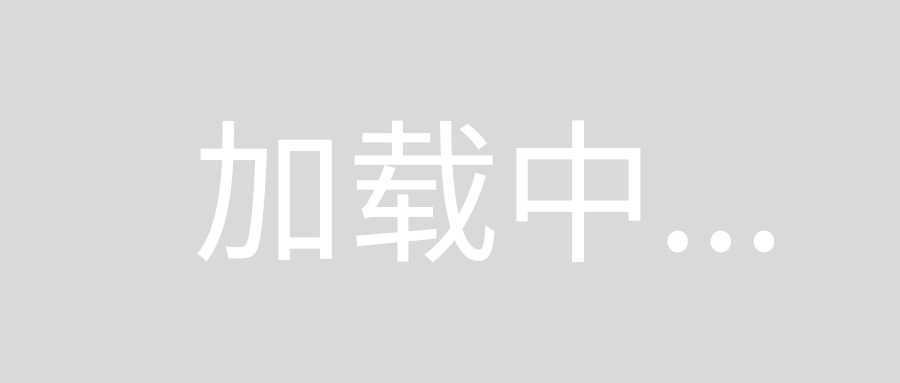
Also allows you to create chrome-less windows that are translucent:
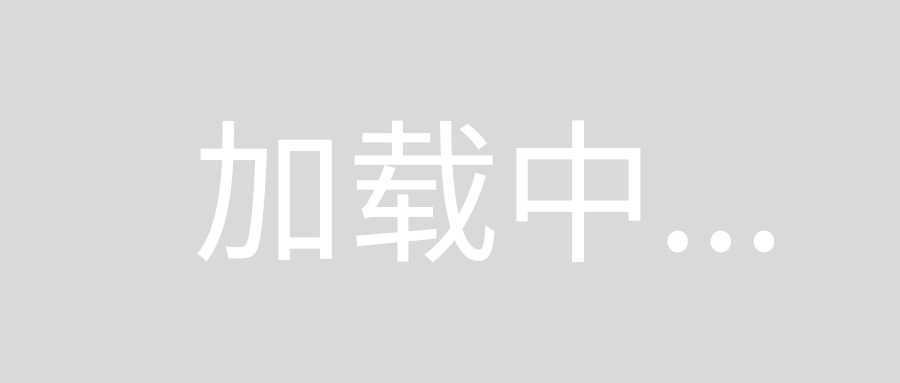