I have a Button
that is defined in a RelativeLayout
as follows:
<Button
android:id="@+id/search_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/current_location"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:paddingBottom="30dp"
android:paddingTop="30dp"
android:text="@string/search" />
I would like to add a ProgressBar behind the Button that occupies the exact same space as the Button, so that when the button is disabled (translucent), you can see the progress bar behind it. How would I do this?
To do this I had to define the button in my main.xml
first, followed by the progress bar as follows:
<Button
android:id="@+id/search_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/current_location"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:paddingBottom="30dp"
android:paddingTop="30dp"
android:text="@string/search" />
<ProgressBar
android:id="@+id/cooldown_bar"
style="@android:style/Widget.ProgressBar.Horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignBottom="@id/search_button"
android:layout_below="@id/current_location"
android:layout_marginBottom="6dp"
android:layout_marginLeft="9dp"
android:layout_marginRight="9dp"
android:layout_marginTop="1dp"
android:indeterminate="false"
android:progressDrawable="@drawable/progress_bar" />
Then, once I had initialized all the GUI components, I had to bring the button back in front of the progress bar with:
searchButton.bringToFront();
The end result
Button when enabled:
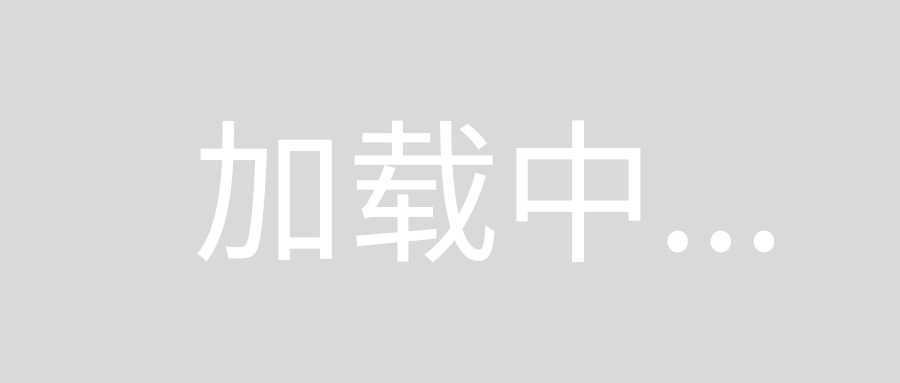
Button when disabled with progress bar:
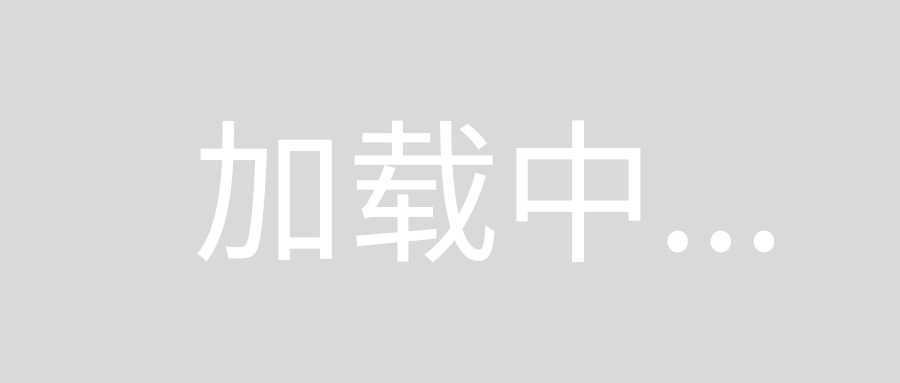
put this right before the button inside the relative layout in your xml:
<ProgressBar
android:id="@+id/descriptive_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/current_location"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
android:paddingBottom="30dp"
android:paddingTop="30dp"
/>
by using all of the same layotu and padding attributes we can make sure the View will be in the same spot and roughly the same size (height could be different because of wrap_content) I think if you put the progress bar first in the layout file then the button should appear on top.
But also you can enforce whatever policy you want with view.setVisibility(visibility-ness)
just make sure whenever you make one visible you make the other invisible.
that way in-case I am wrong about how they stack by default based on their position in the file (which I could be, I didn't verify it just now) and in the event you don't want to move the progress bar to bellow the button in the file, you could still achieve the same thing from the users perspective.