I have already used ng build -prod -aot -vc -cc -dop --buildOptimize
to reduce the size of the builded app.
Now this is my situation:
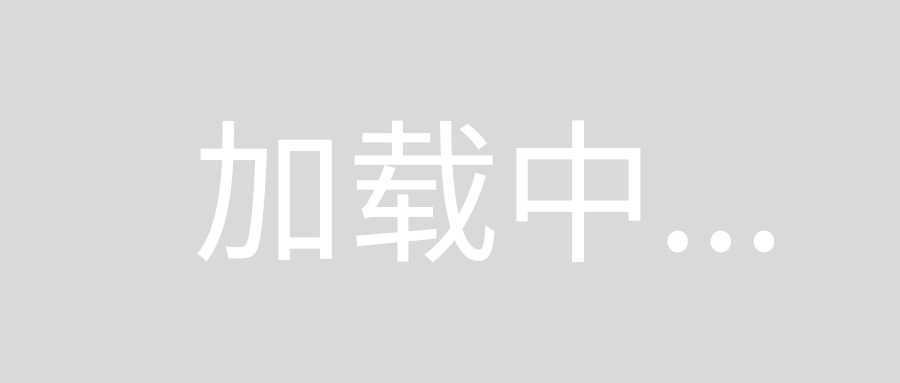
Is it possible to reduce (or maybe split) these bigger chunks?
Is there something that can help me to inspect inside these modules?
P.s. I have already used webpack-analyzer to inspect the situation but it can't go deep into these files.
Build your app with sourcemaps, then use a tool like source-map-explorer that details the size of every part of library that you're using.
Some tips with common libraries :
- If you're using RxJS 5.5 or higher, use the
.pipe(operator1(), operator2())
syntax, it significantly reduces RxJS size when you don't use many different operators
- If you're using moment.js be sure to import only the locales you're using
- If you're using Angular Material, be sure you don't import unused modules
- If you're using bootstrap and SASS, import only the needed parts of
.scss
files
If you didn't already do it, divide your app into lazy modules, it will reduce the size of the initial chunk.
I had a similar issue with large prod bundles and fixed the issue with this command;
npm run build
I had been using
npm run ng build --prod
but the --prod flag doesn't execute and I was left with a vendor.bundle.js which was 2.9mbs
chunk {inline} inline.bundle.js, inline.bundle.js.map (inline) 3.89 kB [entry] [rendered]
chunk {main} main.bundle.js, main.bundle.js.map (main) 122 kB [initial] [rendered]
chunk {polyfills} polyfills.bundle.js, polyfills.bundle.js.map (polyfills) 349 kB [initial] [rendered]
chunk {styles} styles.bundle.js, styles.bundle.js.map (styles) 16.6 kB [initial] [rendered]
chunk {vendor} vendor.bundle.js, vendor.bundle.js.map (vendor) 2.96 MB [initial] [rendered]
With just npm run build (no ng) the whole dist folder is 400kb
chunk {0} polyfills.67d5a7eec41ba0d20dc4.bundle.js (polyfills) 101 kB [initial] [rendered]
chunk {1} main.3874ea54d23e70290fa8.bundle.js (main) 327 kB [initial] [rendered]
chunk {2} styles.0aad5eda327b47b9e9fa.bundle.css (styles) 1.56 kB [initial] [rendered]
chunk {3} inline.318b50c57b4eba3d437b.bundle.js (inline) 796 bytes [entry] [rendered]
Sorry For Late Reply.
This answer will work for all newer versions of Angular.
- If you are using Angular Material, Then import from the specific package exporter.
Ex:
import {MatButtonModule} from '@angular/material/button';
istead of
import {MatButtonModule} from '@angular/material';
- Avoid using Common Material Module throughout your application.
If you are using LazyLoading module, Import required packages in the specific module.
I followed these steps, I got reduced bundle size from 9.0MB to 1.45MB.
Hope it will work for you guys also :).