I have used SDWebImage with Objective C and it worked great for me but now I am learning Swift and trying to integrate the latest version of the API but I am stucking at every step as API is in Objective C and there are no steps mentioned to use the API with Swift.
I read the documents and created the bridge header file and included the required file like below:
#ifndef MyProject_Bridging_Header_h
#define MyProject_Bridging_Header_h
#import <SDWebImage/UIImageView+WebCache.h>
#import "UIImageView+WebCache.h"
#endif
I have added the frameworks as well and dragged the SDWebImage project within my app as explained here
I am really struggling in this. Please help! For reference I have added an image showing the error!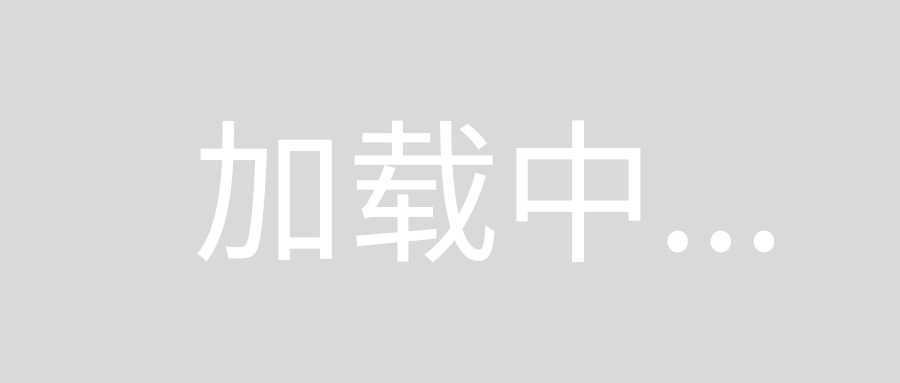
Here is a code example that should work :
let block: SDWebImageCompletionBlock! = {(image: UIImage!, error: NSError!, cacheType: SDImageCacheType!, imageURL: NSURL!) -> Void in
println(self)
}
let url = NSURL(string: "http://placehold.it/350x150")
self.imageView.sd_setImageWithURL(url, completed: block)
and in your bridging header file :
#import "UIImageView+WebCache.h"
So your bridging header file should work, but sometimes I had trouble with the bridging header and in theses cases I just delete it, and add it again and everything works fine after.
The best option will be to drag and drop SDWebImage folder to project. Make sure 'copy items if needed' is ticked on.
Make a Obj C Bridging:File -> New -> Source -> Header File -> Name as AppName-Bridging-Header.
Add the following:
#ifndef AppName_AppName_Bridging_Header_h
#define AppName_AppName_Bridging_Header_h
#import <SDWebImage/UIImageView+WebCache.h>
#import "UIImageView+WebCache.h"
#endif
or
#import "UIImageView+WebCache.h"
Reference: https://developer.apple.com/library/ios/documentation/Swift/Conceptual/BuildingCocoaApps/MixandMatch.html
Note: Build Settings, in Swift Compiler - Code Generation, make sure the Objective-C Bridging Header build setting under has a path to the bridging header file. - its like testSD/testSD-Bridging-Header.h or testSD-Bridging-Header.h (Open the Project folder and find the header file path)
Now try with this code:
let block: SDWebImageCompletionBlock! = {(image: UIImage!, error: NSError!, cacheType: SDImageCacheType!, imageURL: NSURL!) -> Void in
println(self)
}
let url = NSURL(string: "http://arrow_upward.com/350x150")
self.imageView.sd_setImageWithURL(url, completed: block)
Suppose if you are using a UICollectionView to populate Cache imaging, try with this code.
func collectionView(collectionView: UICollectionView, cellForItemAtIndexPath indexPath: NSIndexPath) -> UICollectionViewCell {
let cell = photoListCollectionView.dequeueReusableCellWithReuseIdentifier("scoutimagecellidentifier", forIndexPath: indexPath) as! ScoutImageCell
//Loading image from server using SDWebImage library
let thumbImageUrl = NSURL(string: self.photoPropertyArray[indexPath.row] as String)
//Image Fetching is done in background GCD thread
SDWebImageManager.sharedManager().downloadImageWithURL(thumbImageUrl, options: [],progress: nil, completed: {[weak self] (image, error, cached, finished, url) in
if let wSelf = self {
//On Main Thread
dispatch_async(dispatch_get_main_queue()){
cell.scoutimage.image = image
cell.photoloader.stopAnimating()
}
}
})
return cell
}
swift 3.0 code
import SDWebImage
let url = URL.init(string:"https://vignette3.wikia.nocookie.net/zelda/images/b/b1/Link_%28SSB_3DS_%26_Wii_U%29.png")
imagelogo.sd_setImage(with: url , placeholderImage: nil)