I have a problem understanding an UML below:
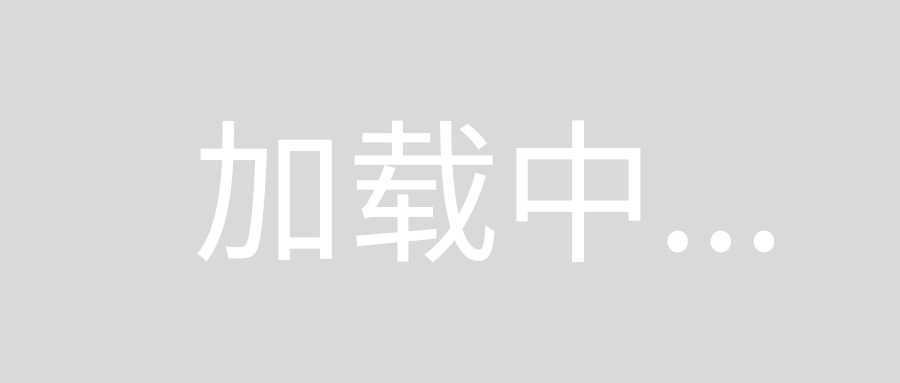
Specifically, what is the relationship between PersistentSet
and ThirdPartyPersistentSet
?
What is the relationship between PersistentObject
and ThirdPartyPersistentSet
?
Please note that the UML is from Agile Principles, Patterns, and Practices in C#
By Martin C. Robert, Martin Micah 2006. Chapter 10
Thanks in advance!
The relationship between PersistentSet and ThirdPartyPersistentSet is an Aggregation, which means the PersistentSet contains one or more ThridPartyPersistenSet instances. This is a "weak" relationship, meaning the instances of ThirdPartyPersistentSet can exist outside of the PersistentSet.
The relationship between PersistentObject and ThirdPartyPersistentSet is a Dependency, meaning basically ThirdPartyPersistentSet needs a PersistentObject to do it's work.
So, to translate this to code, your PersistentSet would contain something like this:
public class PersistentSet
{
public List<ThirdPartyPersistentSet> Items { get; }
...
}
And your ThirdPartyPersistentSet would look something like this:
public class ThirdPartyPersistentSet
{
private PersistentObject _object;
public ThirdPartyPersistentSet(PersistentObject obj)
{
_object = obj;
}
...
}
Specifically, what is the relationship between PersistentSet and ThirdPartyPersistentSet?
The solid diamond <|>-----> is Composition
("has a") where the "parts" are destroyed when the "whole" is. In the image below, if you destroy a Car, you destroy the Carburetor.
The empty diamond < >-----> is Aggregation
("has a") where the "parts" might are not destroyed when the "whole" is. In the image below, if you destroy a pond, you don't necessarily destroy the ducks (they move to a different pond if they are smart).
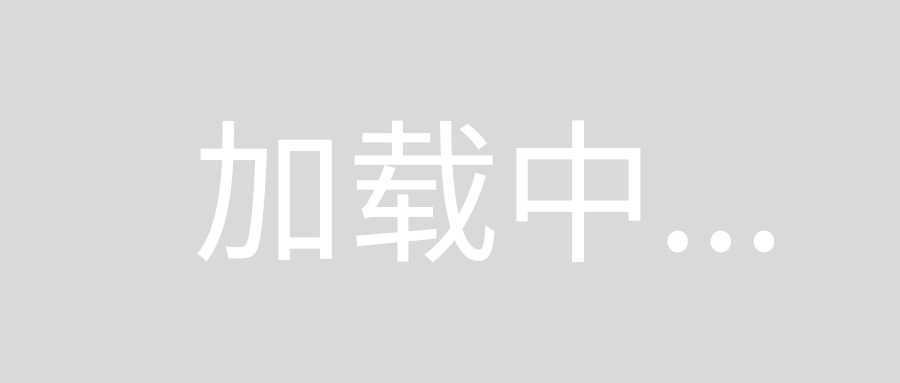
(source: wikimedia.org)
What is the relationship between PersistentObject and ThirdPartyPersistentSet?
This is a dependency relationship. See my answer here for further information.
So when does a dependency relationship change to an association relationship when using parameter passing?
If you store the parameter locally, then it changes from a dependency relationship, to an association relationship. If you only use the parameter locally, then it stays a dependency.
C# Code example:
// Association
public class ThirdPartyPersistentSet
{
private PersistentObject _object;
public ThirdPartyPersistentSet(PersistentObject obj)
{
_object = obj; // Store it to a local variable.
// Now ThirdPartyPersistentSet 'knows' about
// the PersistentObject.
}
}
// Dependency
public class ThirdPartyPersistentSet
{
public ThirdPartyPersistentSet(PersistentObject obj)
{
obj.GetSomething(); // Do something with obj,
// but do not store it to a local variable.
// You only 'use' it and ThirdPartyPersistentSet
// does not 'know' about it.
}
}
Specifically, what is the relationship between PersistentSet and ThirdPartyPersistentSet?
PersistentSet has-many ThirdPartyPersistentSets
What is the relationship between PersistentObject and ThirdPartyPersistentSet?
ThirdPartyPersistentSet is dependent on (uses-a) PersistentObject
all of the lines in a uml class diagram indicate dependencies of one sort or another except for the dashed like to a comment (dog-eared box). a solid line with no arrows indicates a two (2) way (bi-directional) dependency.
The black diamond represents composition, containment (as in having a field of the type pointed by the arrow):
PersistentSet
entities contain ThirdPartyPersistentSet
entities
When PersistenSet is destroyed, all ThirdPartyPersistenSet objects contained will also be destroyed.
The dashed line represents dependency, as in makes a function call that has a parameter of the type pointed by the arrow):
ThirdPartyPersistentSet
depends on PersistentObject
Look at the Wikipedia entry for more details
Specifically, what is the relationship between PersistentSet and ThirdPartyPersistentSet?
http://en.wikipedia.org/wiki/Class_diagram#Aggregation
What is the relationship between PersistentObject and ThirdPartyPersistentSet?
http://en.wikipedia.org/wiki/Class_diagram#Dependency