I have Tab Bar Controller with few tabs.
I am ready to create images with colors needed for selected and non-selected items. And I know how to change this colors for all tabs from AppDelegate
UITabBar.appearance().tintColor = UIColorFromRGB(rgbValue: MY_COLOR, aplhaValue: 1.0)
UITabBarItem.appearance().setTitleTextAttributes([NSForegroundColorAttributeName: UIColorFromRGB(rgbValue: MY_COLOR, aplhaValue: 1.0)], for: UIControlState.selected)
UITabBarItem.appearance().setTitleTextAttributes([NSForegroundColorAttributeName: UIColor.white], for: UIControlState.normal)
But I want to make different colors for different items.
In your Storyboard select your TabBarController and give it a custom class: TabBarController
in this example:
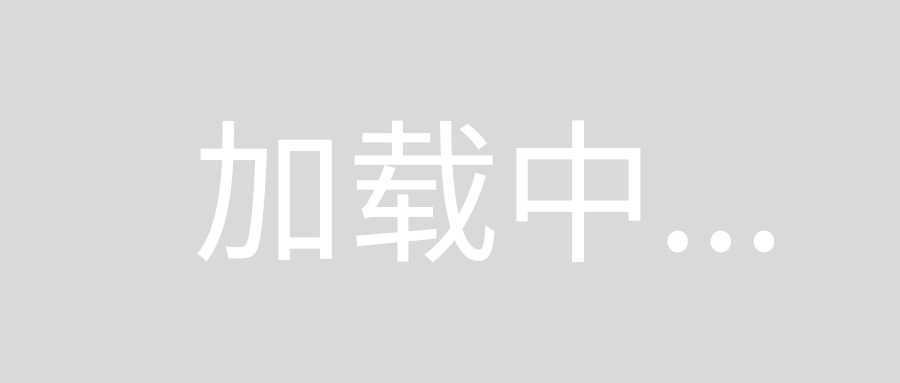
And then create the custom TabBarController file:
// Copyright © 2017 Erick Vavretchek. All rights reserved.
import UIKit
class TabBarController: UITabBarController {
enum tabBarMenu: Int {
case home
case list
case settings
}
// MARK: UITabBarController
override func viewDidLoad() {
super.viewDidLoad()
guard let tabBarMenuItem = tabBarMenu(rawValue: 0) else { return }
setTintColor(forMenuItem: tabBarMenuItem)
}
override func tabBar(_ tabBar: UITabBar, didSelect item: UITabBarItem) {
guard
let menuItemSelected = tabBar.items?.index(of: item),
let tabBarMenuItem = tabBarMenu(rawValue: menuItemSelected)
else { return }
setTintColor(forMenuItem: tabBarMenuItem)
}
// MARK: Private
private func setTintColor(forMenuItem tabBarMenuItem: tabBarMenu) {
switch tabBarMenuItem {
case .home:
viewControllers?[tabBarMenuItem.rawValue].tabBarController?.tabBar.tintColor = UIColor.green
case .list:
viewControllers?[tabBarMenuItem.rawValue].tabBarController?.tabBar.tintColor = UIColor.red
case .settings:
viewControllers?[tabBarMenuItem.rawValue].tabBarController?.tabBar.tintColor = UIColor.red
}
}
}
It is also important that in your Assets.xcassets folder you select the each image you are using for your TabBarItem and set them to Render As: Template Image
. That's how you can simply change its tintColor to whatever you like:
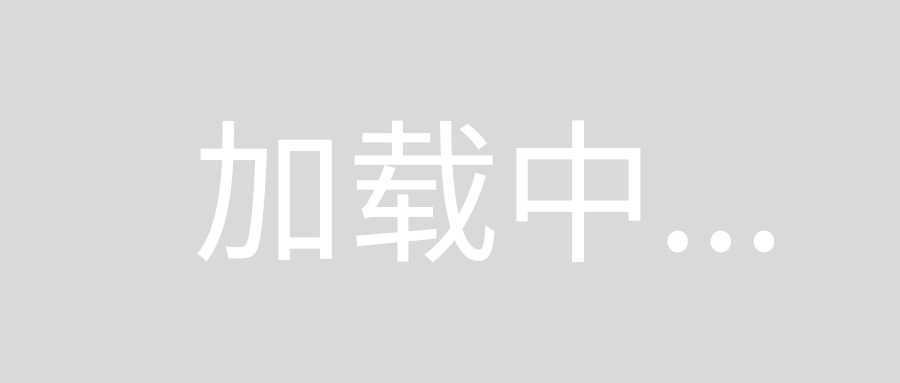