可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I'm using asp.net mvc 3.
I have this dropdownlist of countries and I want to add a placeholder for it. Here's my code:
@Html.DropDownList("country",
new SelectList(ViewBag.countries as System.Collections.IEnumerable,
"name", "name"), new { @class="chzn-select", @placeholder="-select-",
@style="width:160px;" } )
But the placeholder doesn't work, while the style works.
How do I set a placeholder for this?
PS. I just want to use @Html.DropDownList
, not @Html.DropDownListFor
回答1:
In your collection ViewBag.Countries just insert a dummy record at the from of the collection with the name "-select-". You should be able to force the selected item with an alternate constructor like this:
@Html.DropDownList("country",
new SelectList(ViewBag.countries as System.Collections.IEnumerable,
"name", "name", "-select-"), new { @class="chzn-select", @style="width:160px;" } )
回答2:
You could try this:
@Html.DropDownList("country", new SelectList(ViewBag.countries), "-select- ", new { @class="chzn-select", @style="width:160px;" })
回答3:
A quick and (not so) dirty solution involving jQuery.
Instead of adding a dummy item at the start of the list, prepend a new option that is disabled. The main advantage is that you don't have to mess with a dummy item in your list, and most important, you won't be able to select that dummy item in the page:
@Html.DropDownList("yourName", yourSelectList, new { @class = "form-control select-add-placeholder" })
Then somewhere after:
$(".select-add-placeholder").prepend("<option value='' disabled selected>Select an option...</option>");
Which looks like:
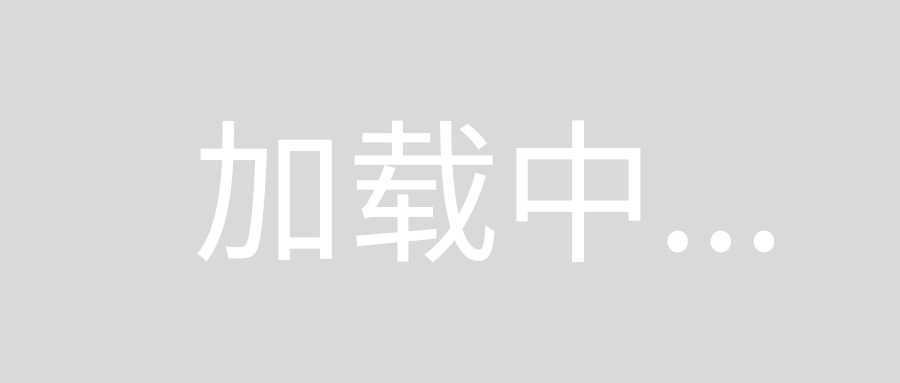
回答4:
@Html.DropDownListFor(m => m.SelectedProductIDs,
Model.AvaliableProducts.ToSelectList("ID","Name"),
new { @class = "chzn-select",
data_placeholder="Please select a product" })...
Please see in more details : http://code.stylenet.ca/mvc3-data-placeholder-html5-attribute/
回答5:
I can see that there is not an easy answer.
I´ve developed a simple solution but it gives some work. This will work for DropDownList and DropDownListFor
First create an Extension Class where ever you want. (Must be Static with static methods)
And Then add the following extension method
public static MvcHtmlString DropDownList(this HtmlHelper htmlHelper,string name, IEnumerable<SelectListItem> selectList, string optionLabel, bool disableLabel)
{
MvcHtmlString mvc = htmlHelper.DropDownList(name, selectList, optionLabel);
if (disableLabel)
{
string disabledOption = mvc.ToHtmlString();
int index = disabledOption.IndexOf(optionLabel);
disabledOption = disabledOption.Insert(index - 1, " disabled");
return new MvcHtmlString(disabledOption);
}
else
{
return mvc;
}
}
Now Note the this Extension only add a new attribute, the disableLabel
This will check if you want to disable or not the label.
Now You let´s go to view´s side
first declare that you want to use the extension class you have created
ex: @using WF.Infrastructure.Extension;
Now you use like it: @Html.DropDownList("Unidade", lstUnidade, "Text of Option Disabled",true)
Note: if you want it to work with DropDownListFor, just add another extension method for your Extension Class with this signature:
public static MvcHtmlString DropDownListFor<TModel, TProperty>(this HtmlHelper<TModel> htmlHelper, Expression<Func<TModel, TProperty>> expression, IEnumerable<SelectListItem> selectList, string optionLabel,bool disableLabel)
PS: If you declare your extension methods in a separated project like me, you will need to add assemblies ( System.Web.Mvc, System.Web)
and in your Extension Class you will need to declare:
using System.Web.Mvc;
using System.Web.Mvc.Html;
回答6:
Maybe if you want, try this:
@Html.DropDownList("country",null,"-SELECT-",new { @class="chzn-select",@style="width:160px;" })
回答7:
@Html.DropDownList("MetadataId", (MultiSelectList)ViewData["category"], "All Categories", new { @class = "chk_dropdown d-none", @id = "categories",multiple = "multiple" })
回答8:
Try this
@Html.DropDownListFor(m => m.SelectedProductIDs, Model.AvaliableProducts.ToSelectList("ID","Name"), new { @class = "chzn-select", data-placeholder="Please select a product" })
Or
@Html.DropDownListFor(model => model.SelectedItemID, new SelectList(Model.employeeList, "Value", "Text"), new Dictionary<string, object> { { "data-placeholder", "Choose a sandbox..." }, { "class", "chzn-select" }, { "style", "width:200px;" } })
回答9:
Best way
@Html.DropDownListFor(m => m.SelectedProductIDs,
Model.AvaliableProducts.ToSelectList("ID","Name"),
"Please select a product",
new { @class = "chzn-select"})
回答10:
@Html.DropDownListFor(m => m.SelectedProductIDs,
Model.AvaliableProducts.ToSelectList("ID","Name"),
"Please select a product",
new {enter code here @class = "chzn-select"})