This is the result that I want.
The number of bits resolution are 256 x 256
.
// assign default background to white.
img = ones(256, 256);
Example Result:
0 1 1 1
0 0 1 1
0 0 0 1
0 0 0 0
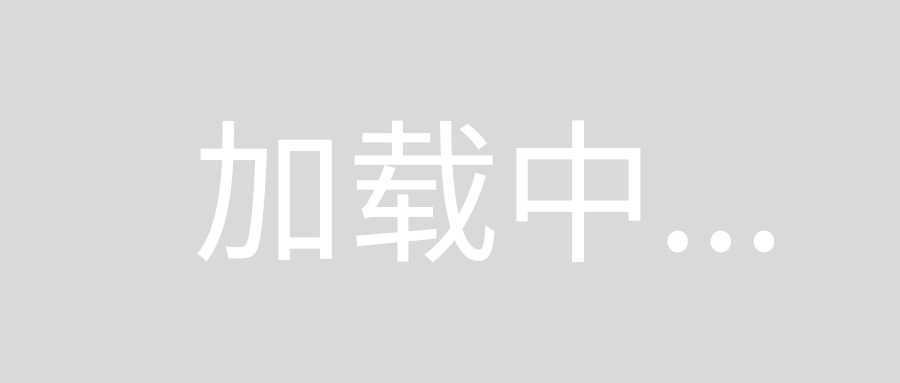
Is there a way that I can use the zeros()
and ones()
function in MATLAB to achieve this result? How should I do the looping?
The result is something that eye()
function can do, but it only do a diagonal lines. I want a diagonal lines that separate zeros and ones.
You are looking for the triu
function
img = triu( ones( 256 ), 1 );
If you care about performance, you can try a bsxfun
based method -
n = 256; %// resolution of img would be nxn
img = bsxfun(@le,[1:n]',0:n-1);
Benchmarks comparing BSXFUN and TRIU -
num_runs = 50000; %// Number of iterations to run benchmarks
n = 256; %// nxn would be the resolution of image
%// Warm up tic/toc.
for k = 1:50000
tic(); elapsed = toc();
end
disp(['For n = ' num2str(n) ' :'])
disp('---------------------- With bsxfun')
tic
for iter = 1:num_runs
out1 = bsxfun(@le,[1:n]',0:n-1); %//'
end
time1 = toc;
disp(['Avg. elapsed time = ' num2str(time1/num_runs) ' sec(s)']),clear out1
disp('---------------------- With triu')
tic
for iter = 1:num_runs
out2 = triu( true( n ), 1 );
end
time2 = toc;
disp(['Avg. elapsed time = ' num2str(time2/num_runs) ' sec(s)']),clear out2
Results
For n = 256 :
---------------------- With bsxfun
Avg. elapsed time = 0.0001506 sec(s)
---------------------- With triu
Avg. elapsed time = 4.3082e-05 sec(s)
For n = 512 :
---------------------- With bsxfun
Avg. elapsed time = 0.00035545 sec(s)
---------------------- With triu
Avg. elapsed time = 0.00015582 sec(s)
For n = 1000 :
---------------------- With bsxfun
Avg. elapsed time = 0.0015711 sec(s)
---------------------- With triu
Avg. elapsed time = 0.0019307 sec(s)
For n = 2000 :
---------------------- With bsxfun
Avg. elapsed time = 0.0058759 sec(s)
---------------------- With triu
Avg. elapsed time = 0.0083544 sec(s)
For n = 3000 :
---------------------- With bsxfun
Avg. elapsed time = 0.01321 sec(s)
---------------------- With triu
Avg. elapsed time = 0.018275 sec(s)
Conclusions from benchmarks
For your 256x256
sized problem, triu
might be the preferred approach, but for decently big enough datasizes, one can look into bsxfun
for upto 50% performance improvement.