可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
In my Flutter app, I have this AppBar
Widget setAppBar(){
return new AppBar(
title: addAppBarTextWidget('Explore'),
elevation: 0.0,
leading: addLeadingIcon(),
actions: <Widget>[
addAppBarActionWidget(Constant.iconNotification, 22.0, 16.0, 8.0),
addAppBarActionWidget(Constant.iconProfile, 30.0, 30.0, 15.0)
],
);
}
Widget addLeadingIcon(){
return new Container(
height: 25.0,
width: 25.0,
padding: const EdgeInsets.fromLTRB(0.0, 0.0, 0.0, 0.0),
margin: const EdgeInsets.fromLTRB(0.0, 0.0, 0.0, 0.0),
child: new Stack(
alignment: AlignmentDirectional.center,
children: <Widget>[
new Image.asset(
Constant.iconNotification,
width: 25.0,
height: 25.0,
),
new FlatButton(
onPressed: (){
onLeadingShowCategoryClick();
}
)
],
),
);
}
it looks like:
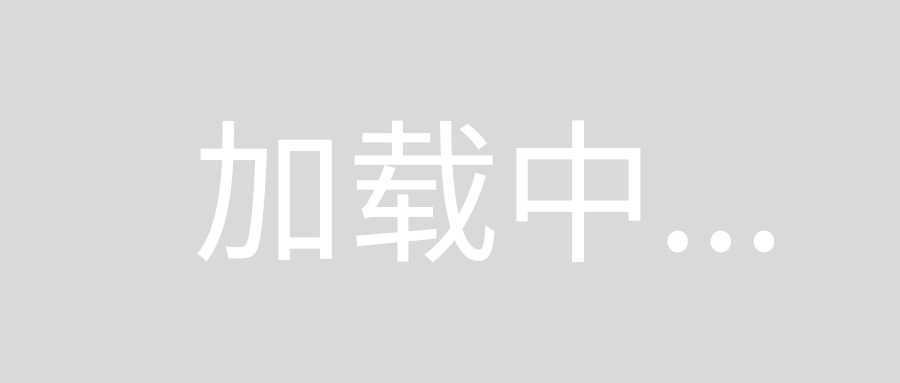
As you can see on the AppBar, there is some extra padding around the
leading icon. How can I remove this extra padding.
回答1:
You can't do this because it is a predefined widget.
You can, however, do this:
appBar: AppBar(
automaticallyImplyLeading: false, // Don't show the leading button
title: Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
IconButton(
onPressed: () => Navigator.pop(context),
icon: Icon(Icons.arrow_back, color: Colors.white),
),
// Your widgets here
],
),
),
Where automaticallyImplyLeading: true
hides the leading IconButton
so you can add your own widgets.
回答2:
Just add a property called titleSpacing,
Sample
appBar: new AppBar(
leading: new Icon(Icons.android),
titleSpacing: 0.0,
title: new Text(widget.title),
),
回答3:
Complete working workaround with some input from Adrian.
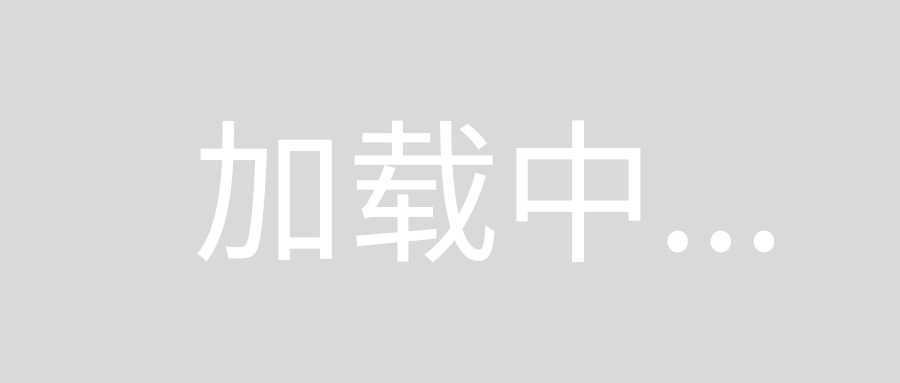
return Scaffold(
key: _scaffoldKey,
appBar: AppBar(
titleSpacing: 0.0,
title: Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
IconButton(
icon: Icon(Icons.menu),
onPressed: () => _scaffoldKey.currentState.openDrawer(),
),
Stack(
alignment: Alignment.center,
children: <Widget>[
IconButton(
icon: Icon(Icons.mail_outline),
onPressed: _onClickNotification,
),
Positioned(
top: 12.0,
right: 10.0,
width: 10.0,
height: 10.0,
child: Container(
decoration: BoxDecoration(
shape: BoxShape.circle,
color: AppColors.notification,
),
),
)
],
),
Expanded(
child: Center(child: Text('test')),
)
],
),
automaticallyImplyLeading: false,
centerTitle: true,
actions: <Widget>[
Row(
children: <Widget>[
Text('Online'),
Switch(
value: true,
onChanged: (bool value) {},
)
],
)
],
),
drawer: Drawer(
child: _buildDrawer(),
),
body: Container(
color: Colors.orange,
),
);
回答4:
If you use Widgets from Material package, they are defined respecting Material Design specification document. So if your modification violates this spec, you have to build your own Widget instead of using the Material Widgets.
回答5:
If you just need to move the icon a bit to the left like I did, you can still use the leading property and just set the alignment on the IconButton:
leading: Builder(
builder: (BuildContext context) {
return IconButton(
onPressed: () => Navigator.pop(context),
icon: MyIcon(),
alignment: Alignment(-0.5, 0.0), // move icon a bit to the left
);
},
),