I am reading content of a local file using jquery
and I get undefined
.
I feel the problem is related to synchronization of URL loading. But I don't have any idea about how to fix this code:
function url_content(url)
{
var content;
$.get(url,function( data ) {content=data});
return content;
}
var content=url_content("local_templates/template1.html");
alert(content);
Its because of the asynchronous nature of javascript. You have to wait for the data to come back before you can use it. You would need to do something like this:
function url_content(url){
return $.get(url);
}
url_content("local_templates/template1.html").success(function(data){
alert(data);
});
or just
$.get("local_templates/template1.html").success(function(data){
alert(data);
doSomeStuffWithTheResult(data);
});
function doSomeStuffWithTheResult(content) {
// code that depends on content goes here...
}
If you absolutely must keep in synchronous you can do this(NOT RECOMMENDED)
function getRemote(remote_url) {
return $.ajax({
type: "GET",
url: remote_url,
async: false
}).responseText;
}
jQuery: Performing synchronous AJAX requests
Asynchronous vs Synchronous
The "A" in "Ajax" is for "Asynchronous". The underlying meaning of this word was hard to figure out for me, I know it can take some time to become familiar with, but it's a critical subject in modern web development and computing in general, hence it's worth a little effort.
Most of the time your code runs in a synchronous way, thus you don't have to worry about whether your environment is ready or not. Considering the following code, it's obvious that "v" is ready for being used at the time you need it, you don't have to wait for it to be initialized.
var v = 1; // no delay during initialization
var u = v + 1; // you can use "v" immediately
Even if there was a delay at this point, a synchronous processing would wait for the instruction to be done before executing the next line. Hence, you can be sure that "v" will be ready when you'll need it. Now, let's see how this code behaves if we introduce an asynchronous task.
function ajax (url) {
// 1. go to url asynchronously
// 2. bring back the value
// 3. return the value
};
var v = ajax('mywebsite.com'); // undefined
var u = v + 1; // "v" is not ready -> NaN
The way the tasks are numbered reflects what's going on in your mind, but it's not correct, actually 3 happens before 2. Roughly speaking, ajax()
won't get the value fast enough and returns before the first task completes, hence, the value is not available and the program fails.
More precisely, the program starts talking to a remote computer as soon as ajax()
is called. Such a task can last a couple of seconds, but the program is aware of its asynchronous nature, hence, it doesn't wait for the result and jumps to the next line immediately.
Now I think you should better understand what's going on. The main point is that a program isn't necessarily executed in a single flow, there might be some tasks executed apart from the rest, in parallel. If you are still confused, take some time to think of it before going further :-)
How to deal with asynchronous tasks ?
So, how to avoid crashing your program while performing asynchronous tasks ? Using callbacks. A callback is a function which is passed to another function as a parameter and called internally. Let's see how to fix the previous code block with a callback :
function ajax (url, callback) {
// 1. go to url asynchronously
// 2. bring the value
// 3. when previous tasks done, do callback(value)
};
ajax('mywebsite.com', function (value) {
var v = value;
var u = v + 1;
});
To fully understand how it works, you need to know that there is an internal mechanism which acts as a middle man between your program and the remote computer. As soon as the response of the remote computer is available, this mechanism calls the callback function.
Well, I think it's enough for now, I won't bother you no more ;-) For details you should browse jQuery's docs on Ajax, it can be a good place to start. As a conclusion, here is a possible implementation of ajax()
(not bullet proof). Enjoy.
function ajax (url, callback) {
var req = new XMLHttpRequest();
req.open('GET', url, true); // true = asynchronous
req.onload = function () {
var value = JSON.parse(this.responseText);
callback(value);
};
req.send(null);
}
Additional stuffs (click to zoom)
Synchronous : the main flow is paused during remote queries.
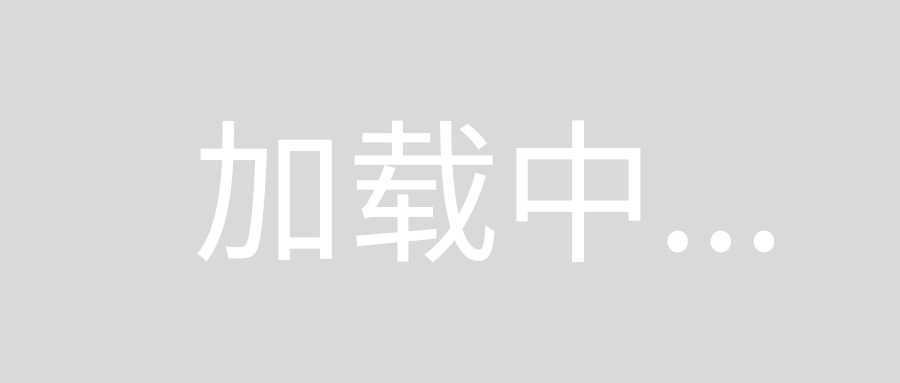
Asynchronous : the main flow keeps going during remote queries.
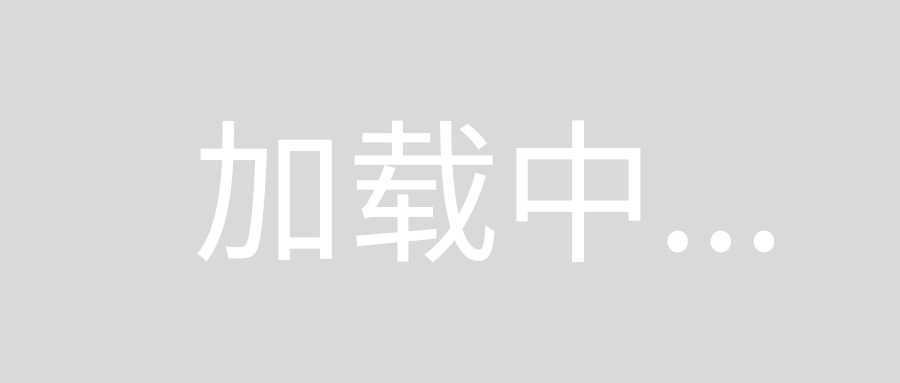