I want to align UIAlertAction
text alignment to Left and add the icon as in image shown. I spent lot of time on google to get the solution but not found the right answer. Every body post for the alert title not for the alert action title.
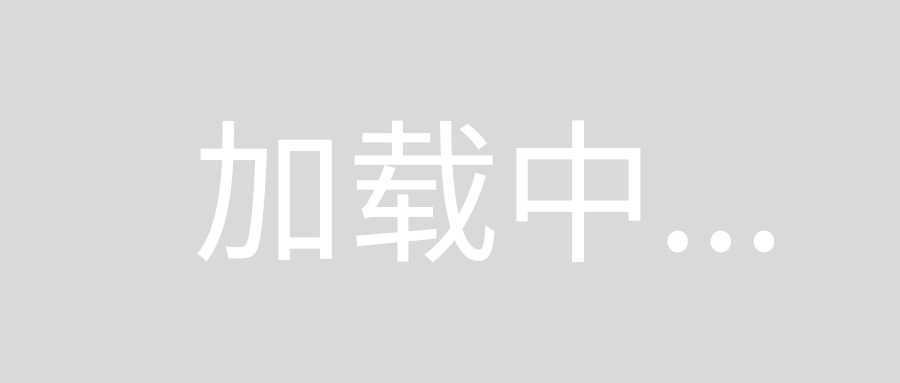
Please suggest me the right way for swift.
Thanks!!
You can do that with bellow example code. Easy and Simple.
Swift 4
let actionSheetAlertController: UIAlertController = UIAlertController(title: nil, message: nil, preferredStyle: .actionSheet)
let cancelActionButton = UIAlertAction(title: "Cancel", style: .cancel, handler: nil)
actionSheetAlertController.addAction(cancelActionButton)
let documentsActionButton = UIAlertAction(title: "Documents", style: .default, handler: nil)
actionSheetAlertController.addAction(documentsActionButton)
documentsActionButton.setValue(#imageLiteral(resourceName: "doccument"), forKey: "image")
documentsActionButton.setValue(kCAAlignmentLeft, forKey: "titleTextAlignment")
let cameraActionButton = UIAlertAction(title: "Camera", style: .default, handler: nil)
actionSheetAlertController.addAction(cameraActionButton)
cameraActionButton.setValue(#imageLiteral(resourceName: "camera"), forKey: "image")
cameraActionButton.setValue(kCAAlignmentLeft, forKey: "titleTextAlignment")
let galleryActionButton = UIAlertAction(title: "Gallery", style: .default, handler: nil)
actionSheetAlertController.addAction(galleryActionButton)
galleryActionButton.setValue(#imageLiteral(resourceName: "gallery"), forKey: "image")
galleryActionButton.setValue(kCAAlignmentLeft, forKey: "titleTextAlignment")
actionSheetAlertController.view.tintColor = kTHEME_COLOR
self.present(actionSheetAlertController, animated: true, completion: nil)
You can do that with bellow example code.
Replace image name with your image
Swift 3
let actionSheet = UIAlertController(title: nil, message: nil, preferredStyle: .actionSheet)
let camera = UIAlertAction(title: "Camera", style: .default) { (action) in
// Do something
}
let cameraImage = UIImage(named: "icon_camera")
camera.setValue(cameraImage, forKey: "image")
camera.setValue(0, forKey: "titleTextAlignment")
actionSheet.addAction(camera)
An updated version to swift 5.0 of kCAAlignmentLeft
(as answered by @Niraj Solanki here) is CATextLayerAlignmentMode.left
So the code should be as below:
Swift 5.0
documentsActionButton.setValue(CATextLayerAlignmentMode.left, forKey: "titleTextAlignment")
As already said in comments, UIAlertController do not provide customization of its view. However, you can build your own action sheet view or use some third parties, like https://github.com/xmartlabs/XLActionController.