This question already has an answer here:
-
Only the last Object is added to the ArrayList
4 answers
-
Add an object to an ArrayList and modify it later
4 answers
While adding objects to list I was able to see the object is replacing all values in the list.
Please check the below image and notice the code in for loop for duplicates of object in the list.
public static void main(String args[]) {
ArrayList<Modelclass> al = new ArrayList<Modelclass>();
Modelclass obj = new Modelclass();
for (int i = 0; i < 10; i++) {
obj.setName(2 + i);
obj.setRoolno(4 + i);
System.out.println(obj);
//if (!al.equals(obj)) {
al.add(obj);
System.out.println(obj.getName() + "" + obj.getRoolno());
//}
}
}
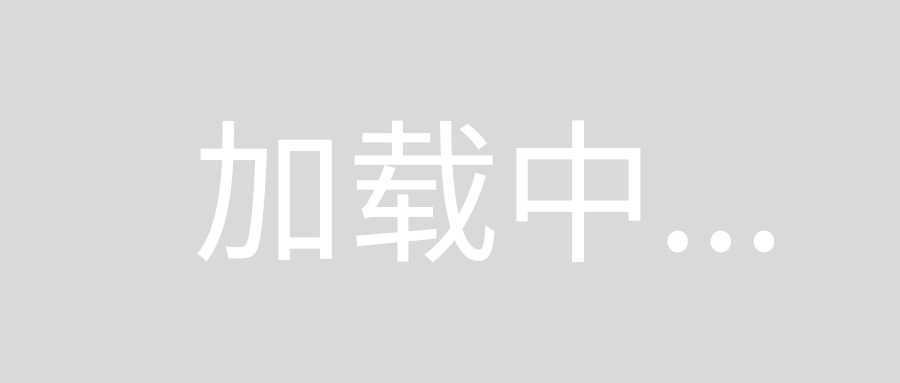
You are always adding the same
Modelclass obj = new Modelclass();
That you created outside of the for loop. Then, inside the loop, you are modifying the values.
Since it is always a reference to the same object, you are modifying all of the items in the ArrayList.
Try this instead:
for (int i = 0; i < 10; i++) {
Modelclass obj = new Modelclass(); //This is the key to solve it.
obj.setName(2 + i);
obj.setRoolno(4 + i);
System.out.println(obj);
al.add(obj);
System.out.println(obj.getName() + "" + obj.getRoolno());
}
Your obj
variable is only being instantiated once, but being added to the list multiple times. Whenever you update the members of obj
, you are updating the same piece of memory and thus every list reference shows the same (last added) data.
I guess you are new in Java? Just create new instances in the loop will work.
ArrayList<ModelClass> al = new ArrayList<ModelClass>();
for(int i=0; i<10; i++){
ModelClass obj = new ModelClass();
obj.setName(2+i);
obj.setRoolno(4+i);
al.add(obj);
}
Your problem is that you're referencing the same object cause you created the object before the loop. Should be
public static void main(String args[]) {
ArrayList<Modelclass> al = new ArrayList<Modelclass>();
for (int i = 0; i < 10; i++) {
Modelclass obj = new Modelclass();
obj.setName(2 + i);
obj.setRoolno(4 + i);
System.out.println(obj);
//if (!al.equals(obj)) {
al.add(obj);
System.out.println(obj.getName() + "" + obj.getRoolno());
//}
}
}