可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
If EditText
is empty then Login Button
has to be disabled. And if EditText
has some texts then Login Button
has to be enabled. Well you can see this method on Instagram Login.
Both fields are empty, Sign in Button
is DISABLED.
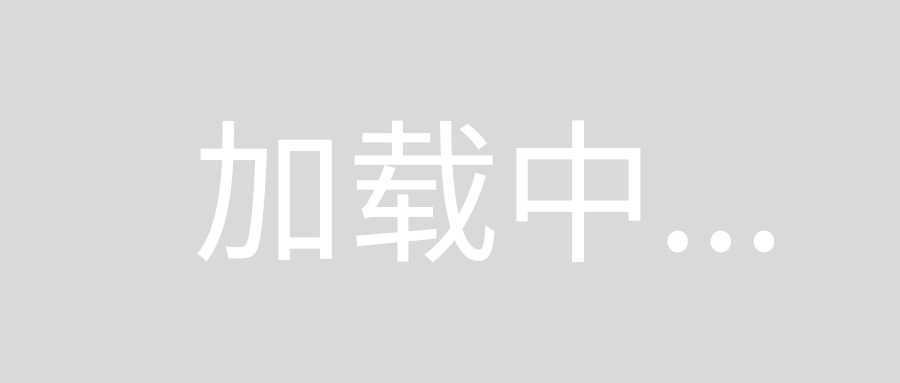
Here Password field is empty, so still Sign inButton
is DISABLED.
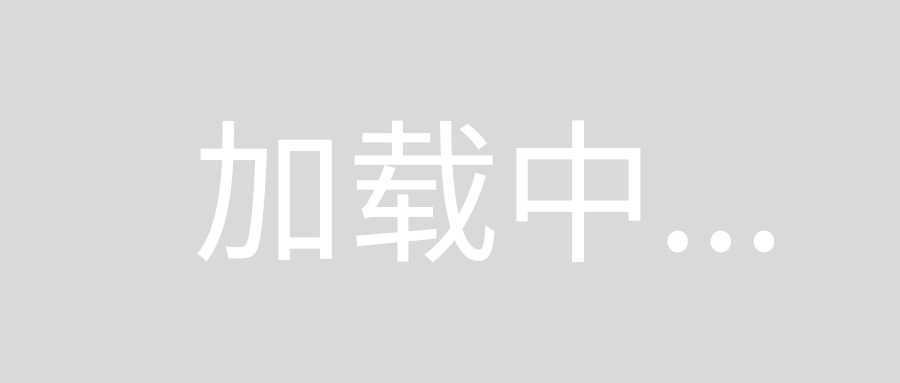
Here both Username and Password field is not empty, So Sign inButton
is ENABLED.
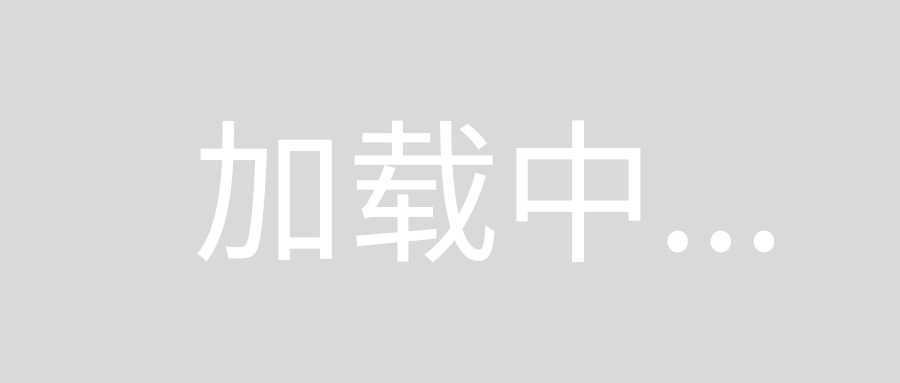
how to achieve these steps??
here is my code and it doesn't work..
EditText et1,et2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login_check);
et1 = (EditText) findViewById(R.id.editText1);
et2 = (EditText) findViewById(R.id.editText2);
Button b = (Button) findViewById(R.id.button1);
String s1 = et1.getText().toString();
String s2 = et2.getText().toString();
if(s1.equals("")|| s2.equals("")){
b.setEnabled(false);
} else {
b.setEnabled(true);
}
}
回答1:
heres what you are looking for :
private EditText et1,et2;
// create a textWatcher member
private TextWatcher mTextWatcher = new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence charSequence, int i, int i2, int i3) {
}
@Override
public void onTextChanged(CharSequence charSequence, int i, int i2, int i3) {
}
@Override
public void afterTextChanged(Editable editable) {
// check Fields For Empty Values
checkFieldsForEmptyValues();
}
};
void checkFieldsForEmptyValues(){
Button b = (Button) findViewById(R.id.button1);
String s1 = et1.getText().toString();
String s2 = et2.getText().toString();
if(s1.equals("")|| s2.equals("")){
b.setEnabled(false);
} else {
b.setEnabled(true);
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login_check);
et1 = (EditText) findViewById(R.id.editText1);
et2 = (EditText) findViewById(R.id.editText2);
// set listeners
et1.addTextChangedListener(mTextWatcher);
et2.addTextChangedListener(mTextWatcher);
// run once to disable if empty
checkFieldsForEmptyValues();
}
回答2:
You need to implement TextWatcher on EditText
to achieve the result.
EditText et1, et2;
Button b;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
et1 = (EditText) findViewById(R.id.editText1);
et2 = (EditText) findViewById(R.id.editText2);
b = (Button) findViewById(R.id.button1);
checkValidation();
et1.addTextChangedListener(mWatcher);
et2.addTextChangedListener(mWatcher);
}
private void checkValidation() {
// TODO Auto-generated method stub
if ((TextUtils.isEmpty(et1.getText()))
|| (TextUtils.isEmpty(et2.getText())))
b.setEnabled(false);
else
b.setEnabled(true);
}
TextWatcher mWatcher = new TextWatcher() {
@Override
public void onTextChanged(CharSequence s, int start, int before,
int count) {
// TODO Auto-generated method stub
checkValidation();
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count,
int after) {
// TODO Auto-generated method stub
}
@Override
public void afterTextChanged(Editable s) {
// TODO Auto-generated method stub
}
};
回答3:
You need to track user's action inside EditText
using TextWatcher
object:
myEditText.addTextChangedListener(new TextWatcher()
{
@Override
public void onTextChanged(CharSequence s, int start, int before, int count)
{
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after)
{
}
@Override
public void afterTextChanged(Editable s)
{
if (s.length() > 1)
{
//enable button
} else
//disable
}
});
回答4:
try this:
EditText et1,et2;
Button b;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login_check);
et1 = (EditText) findViewById(R.id.editText1);
et2 = (EditText) findViewById(R.id.editText2);
b = (Button) findViewById(R.id.button1);
et1.addTextChangedListener(new TextWatcher() {
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
String s1 = et1.getText().toString();
String s2 = et2.getText().toString();
if(s1.equals("") && s2.equals("")){
b.setEnabled(false);
}
else if(!s1.equals("")&&s2.equals("")){
b.setEnabled(false);
}
else if(!s2.equals("")&&s1.equals(""){
b.setEnabled(false);
}
else {
b.setEnabled(true);
}
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count,
int after) {
// TODO Auto-generated method stub
}
@Override
public void afterTextChanged(Editable s) {
// TODO Auto-generated method stub
}
});
et2.addTextChangedListener(new TextWatcher() {
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
String s1 = et1.getText().toString();
String s2 = et2.getText().toString();
if(s1.equals("") && s2.equals("")){
b.setEnabled(false);
}
else if(!s1.equals("")&&s2.equals("")){
b.setEnabled(false);
}
else if(!s2.equals("")&&s1.equals(""){
b.setEnabled(false);
}
else {
b.setEnabled(true);
}
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count,
int after) {
// TODO Auto-generated method stub
}
@Override
public void afterTextChanged(Editable s) {
// TODO Auto-generated method stub
}
});
}
回答5:
You need to attach a TextWatcher that is called whenever the text in one of the EditText fields is changed.
private EditText mName;
private EditText mPassword;
private Button mButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login_check);
mName = (EditText) findViewById(R.id.editText1);
mPassword = (EditText) findViewById(R.id.editText2);
mButton = (Button) findViewById(R.id.button1);
mName.addTextChangedListener(mWatcher);
mPassword.addTextChangedListener(mWatcher);
}
private TextWatcher mWatcher = new TextWatcher() {
@Override
public void afterTextChanged(Editable s) {
boolean nameNotEmpty = mName.getText().length()>0;
boolean pwNotEmpty = mPassword.getText().length()>0;
mButton.setEnabled(nameNotEmpty && pwNotEmpty);
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {}
};
回答6:
private TextWatcher mPhoneNumberEtWatcher = new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence charSequence, int i, int i1, int i2) {}
@Override
public void onTextChanged(CharSequence charSequence, int i, int i1, int i2) {
if (charSequence.length() >= 10) {
mPhoneImg.setImageDrawable(getResources().getDrawable(R.drawable.phone_activate));
if (mPasswordEt.getText().toString().length() >= 5) {
mLoginBtn.setEnabled(true);
}
} else {
mPhoneImg.setImageDrawable(getResources().getDrawable(R.drawable.phone));
mLoginBtn.setEnabled(false);
}
}
@Override
public void afterTextChanged(Editable editable) {
}
};
mPhoneNumberEt.addTextChangedListener(mPhoneNumberEtWatcher);
You should use TextWatcher. That will call method after typing of user. And you can check length and somthing else of your edit text.
回答7:
try this
if(s1.equals("") && s2.equals(""))
{
b.setEnabled(true);
// to change color of the button you need to apply style to the button[here refer custom bg][1]
}
else
{
b.setEnabled(false);
//do nothing or display toast msg
}
回答8:
I only want to add that the check will NOT work if the InputType
of the EditText
is a password (or similar) and the fuction to proof the length of the text (see other answers) is called from
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {...}
So it is important to call the check from
@Override
public void afterTextChanged(Editable s) {...}
回答9:
hey if want to use the code cut the button needs to change color if the editText1_id and the editText1_passcode is in and in 4 digits
checkValidation();
editText1_id.addTextChangedListener(mWatcher);
editText1_passcode.addTextChangedListener(mWatcher);
}
private void checkValidation() {
// TODO Auto-generated method stub
if ((TextUtils.isEmpty(editText1_id.getText()))
|| (TextUtils.isEmpty(editText1_passcode.getText())))
loginbtn.setEnabled(false);
else
loginbtn.setEnabled(true);
}
TextWatcher mWatcher = new TextWatcher() {
@Override
public void onTextChanged(CharSequence s, int start, int before,
int count) {
// TODO Auto-generated method stub
checkValidation();
}
@Override
public void beforeTextChanged(CharSequence s, int start, int count,
int after) {
// TODO Auto-generated method stub
}
@Override
public void afterTextChanged(Editable s) {
// TODO Auto-generated method stub
}
};
}
回答10:
1 Line Solution?
Setting up Button dependent on EditText is Super Easy in Data-Binding. You can manage it via only XML.
android:enabled="@{etName.text.length() > 5 && etPassword.text.length() > 5}"
Complete XML -
<LinearLayout
>
<EditText
android:id="@+id/etName"
/>
<EditText
android:id="@+id/etPassword"
/>
<Button
android:enabled="@{etName.text.length() > 5 && etPassword.text.length() > 5}"
/>
</LinearLayout>