I've looked up some documentations and examples under the http://developers.itextpdf.com/examples.
I know iText is able to generate tagged pdf from scratch, but is it possible to insert alternative text to images in an existing tagged pdf (without changing anything else)? I need to implement this feature in a program without using GUI applications (such as Adobe Acrobat Pro). Thanks.
Please take a look at the AddAltTags example.
In this example, we take a PDF with images of a fox and a dog where the Alt keys are missing: no_alt_attribute.pdf
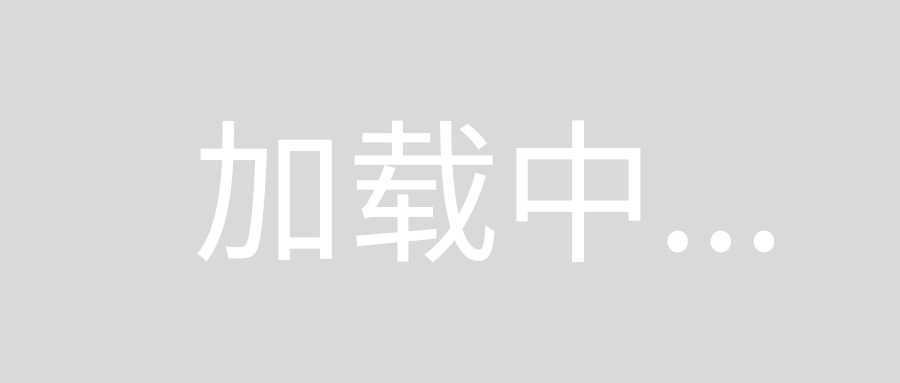
Code can't recognize a fox or a dog, so we create a new document with Alt attributes saying "Figure without an Alt description": added_alt_attributes.pdf)
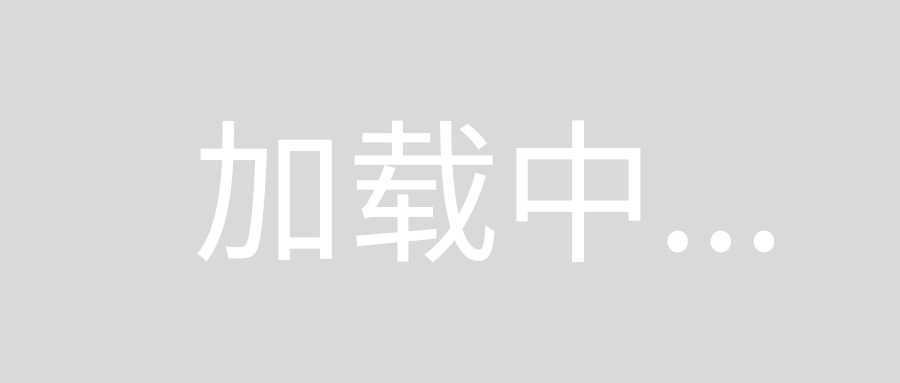
We add this description by walking through the structure tree, looking for structural elements marked as /Figure
elements:
public void manipulatePdf(String src, String dest)
throws IOException, DocumentException {
PdfReader reader = new PdfReader(src);
PdfDictionary catalog = reader.getCatalog();
PdfDictionary structTreeRoot =
catalog.getAsDict(PdfName.STRUCTTREEROOT);
manipulate(structTreeRoot);
PdfStamper stamper = new PdfStamper(
reader, new FileOutputStream(dest));
stamper.close();
}
public void manipulate(PdfDictionary element) {
if (element == null)
return;
if (PdfName.FIGURE.equals(element.get(PdfName.S))) {
element.put(PdfName.ALT,
new PdfString("Figure without an Alt description"));
}
PdfArray kids = element.getAsArray(PdfName.K);
if (kids == null) return;
for (int i = 0; i < kids.size(); i++)
manipulate(kids.getAsDict(i));
}
You can easily port this Java example to C#:
- Get the root dictionary from the
PdfReader
object,
- Get the root of the structure tree (a dictionary),
- Loop over all the kids of every branch of that tree,
- When a lead is a figure, add an
/Alt
entry.
Once this is done, use PdfStamper
to save the altered file.