I am getting { text/plain {NULL} }
when I am using ClipData
but if I use deprecated method mClipboard.getText()
it is working just fine.
if (mClipboard.getPrimaryClipDescription().hasMimeType(ClipDescription.MIMETYPE_TEXT_PLAIN)) {
ClipData clipData = mClipboard.getPrimaryClip();
ClipData.Item item = clipData.getItemAt(0);
Log.d(TAG, clipData.toString());
Log.d(TAG, mClipboard.getText());
}
Update
Issue exists in Samsung galaxy Tab 3.
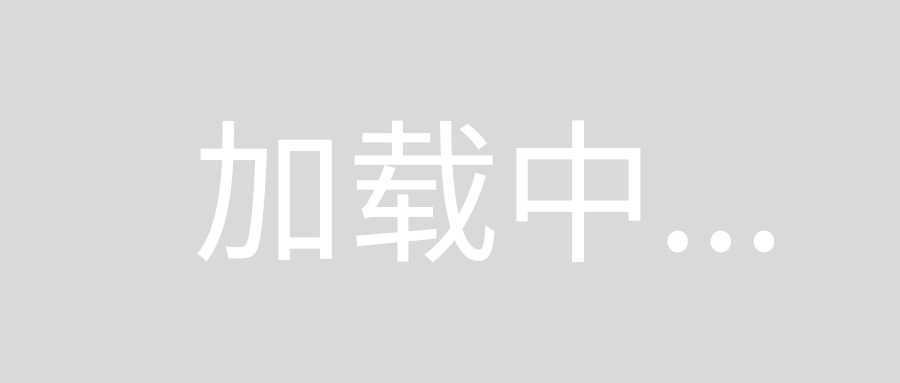
The reason of your problem is unknown. since it works on a device which I tested on (S6 5.0). You may want to look at the implementation of deprecated getText()
method:
public CharSequence getText() {
ClipData clip = getPrimaryClip();
if (clip != null && clip.getItemCount() > 0) {
return clip.getItemAt(0).coerceToText(mContext);
}
return null;
}
In order to obtain the text It uses the method coerceToText() . according to description of this method:
* Turn this item into text, regardless of the type of data it
* actually contains.
Therefore , I presume the deprecation of method getText() is due to a performance concern or something else.
Anyway. Since method getText()
uses API which is not deprecated, as a workaround you can use some part of the source of this method(specifically, method coerceToText()
) if calling recommended API returns null:
ClipboardManager mclipboard =(ClipboardManager) getSystemService(CLIPBOARD_SERVICE);
boolean isTextPlain = mclipboard.getPrimaryClip().getDescription().hasMimeType(ClipDescription.MIMETYPE_TEXT_PLAIN);
CharSequence text = null;
if (isTextPlain){
ClipData clipData = mclipboard.getPrimaryClip();
ClipData.Item item = clipData.getItemAt(0);
if ( item!= null ){
text = item.getText();
if (text == null){
// taken from source of clipData.getText() method
text = item.coerceToText(this);
}
}
}