My app/JFrame, using Borderlayout, has a toolbar at the top or north, a statusbar at the bottom or south and a JPanel.JTabbedPane.JScrollPane.JTable in the center. The JPanel is always a fixed size which is roughly adjustable using the various set*Size() methods applied in various combinations to the various components. But it's always a fixed size and always has east and west gaps. The north and south components stay fixed height and resize horizontally as one would expect.
Surely this is not a new or unique design.
Is this normal behaviour?
Is there some trick I've missed?
This is characteristic of retaining the default FlowLayout
of JPanel
and adding the panel to the center of a BorderLayout
. The example below compares panels having FlowLayout
, the default, or GridLayout
. For contrast, the two are added to a GridLayout
, which allows expansion in a manner similar to that of BorderLayout
center.
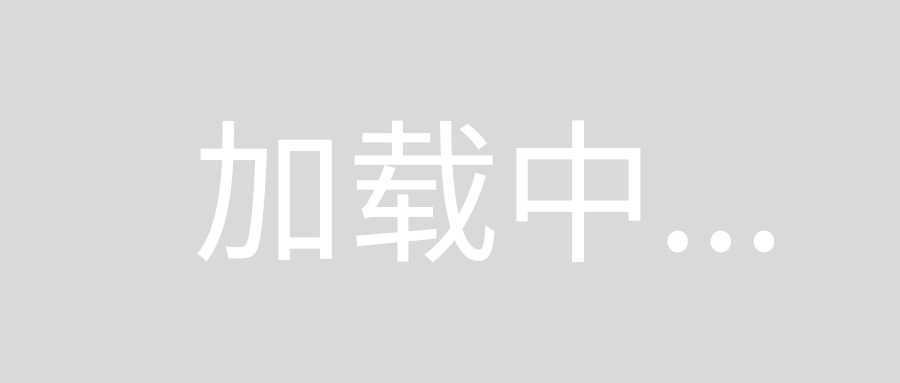
import java.awt.EventQueue;
import java.awt.GridLayout;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTree;
/** @see http://stackoverflow.com/questions/5822810 */
public class LayoutPanel extends JPanel {
public LayoutPanel(boolean useGrid) {
if (useGrid) {
this.setLayout(new GridLayout());
} // else default FlowLayout
this.add(new JTree());
}
private static void display() {
JFrame f = new JFrame("LayoutPanels");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setLayout(new GridLayout(1, 0));
f.add(new LayoutPanel(false));
f.add(new LayoutPanel(true));
f.pack();
f.setLocationRelativeTo(null);
f.setVisible(true);
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
display();
}
});
}
}