So I am designing a JFrame using Eclipse WindowBuilder. This specific frame is an error message stating that the user provided invalid credentials. I have added a button to exit the frame and I now need to display the actual error message "The login credentials specified are invalid. Please provide valid credentials."
I have done some searching and everyone says to use a JLabel, but when I create my JLabel and enter the text to it, there is no wordwrap or anything so I can't fit the label inside my frame.
What is an easy way to simply display a message in the center of the JFrame?
To create a label for text:
JLabel label1 = new JLabel("Test");
To change the text in the label:
label1.setText("Label Text");
And finally to clear the label:
label1.setText("");
And all you have to do is place the label in your layout, or whatever layout system you are using, and then just add it to the JFrame...
Instead of wasting your time to design a JFrame
just to display a error message, you can use an JOptionPane
which is by default modal:
import javax.swing.JOptionPane;
public class Main {
public static void main(String[] args) {
JOptionPane.showMessageDialog(null, "Your message goes here!","Message", JOptionPane.ERROR_MESSAGE);
}
}
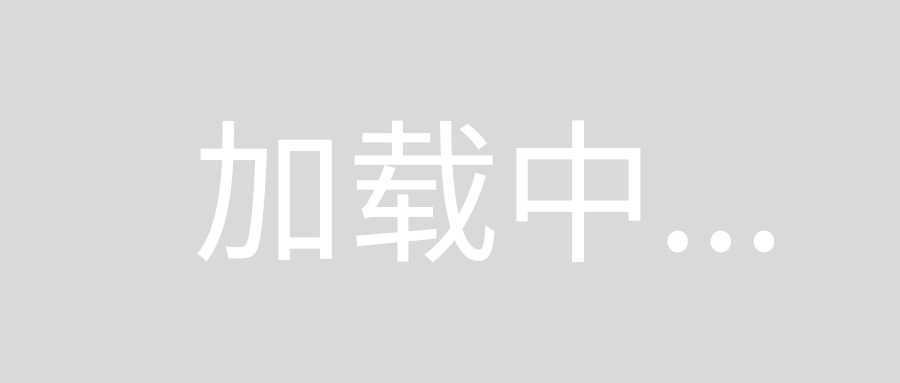
P.S. Stop using Windowbuilder if you want to learn Swing.
when I create my JLabel and enter the text to it, there is no wordwrap or anything
HTML formatting can be used to cause word wrap in any Swing component that offers styled text. E.G. as demonstrated in this answer.
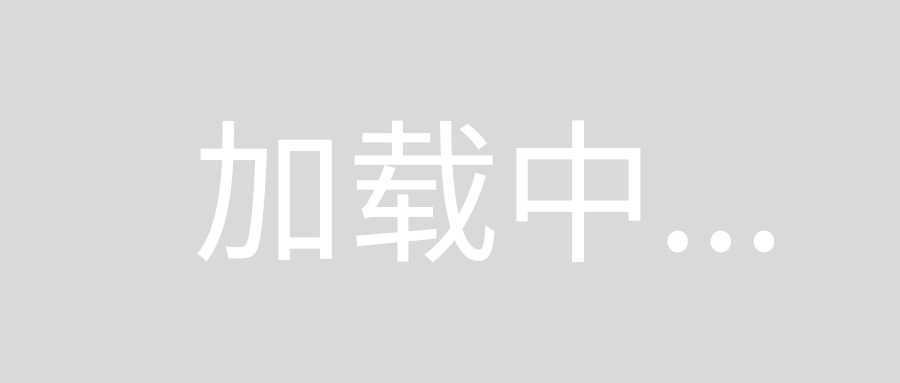
You can add a multi-line label with the following:
JLabel label = new JLabel("My label");
label.setText("<html>This is a<br>multline label!<br> Try it yourself!</html>");
From here, simply add the label to the frame using the add() method, and you're all set!
The easiest way to add a text to a JFrame:
JFrame window = new JFrame("JFrame with text");
window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
window.setLayout(new BorderLayout());
window.add(new JLabel("Hello World"), BorderLayout.CENTER);
window.pack();
window.setVisible(true);
window.setLocationRelativeTo(null);