HTML
<input class="button" type="button" onclick="$.reload('results')" value="Search">
I don't have an id or name for this . Hence am writing
FirefoxDriver driver = new FirefoxDriver();
driver.get("http://....");
driver.findElement(By.cssSelector("input[value=Search]")).click();
But click() is not happening.
Tried
driver.findElement(By.cssSelector(".button[value=Search]")).click();
Tried
value='Search' (single quotes).
these Selectors are working in
.button[value=Search] {
padding: 10px;
}
input[value=Search] {
padding: 10px;
}
i would inject piece of js to be confident in resolving this issue:
first of all locate element using DOM (verify in firebug):
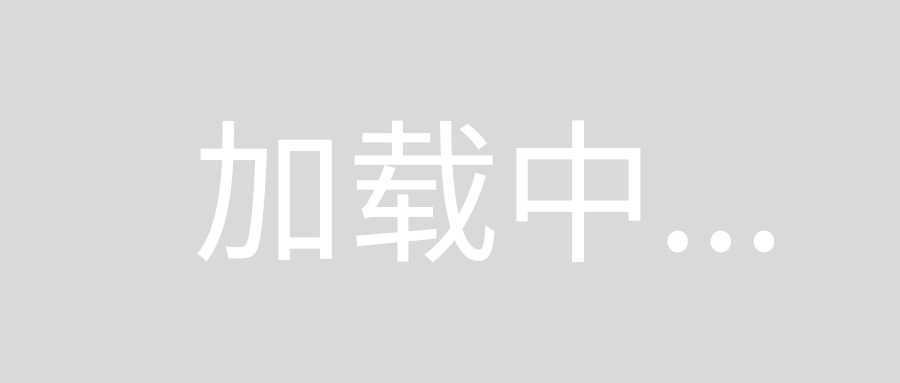
public void jsClick(){
JavascriptExecutor js = (JavascriptExecutor) driver;
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("document.getElementsByTagName('button')[0].click();");
js.executeScript(stringBuilder.toString());
}
jsClick();
from the retrospective of your element it be like:
....
stringBuilder.append("document.getElementsByTagName('input')[0].click();");
....
Please, note: document.getElementsByTagName('input')
returns you an array of DOM elements. And indexing it properly e.g. document.getElementsByTagName('input')[0], document.getElementsByTagName('input')1, document.getElementsByTagName('input')[2]....
,etc you will be able to locate your element.
Hope this helps you.
Regards.
Please use the below code.
driver.findElement(By.cssSelector("input[value=\"Search\"]")).click();
It works for me. And make sure that the name is "Search", coz it is case sensitive.
Thanks
Are you sure that using this CSS-selector (input[value=Search]
) on your page you have only one result?
single quotes are missing in your code, the [value=Search] should be replaced with [value='Search'].
first you have to check if the selector u are using will work or not..
If you are using chrome or FF,you can follow these steps,
go to the page where button (to be clicked) is present,
open web console and type in the following and click enter..
$("input[value='Search']")
or
$("input[value='Search'][type='button']")
or
$("input[value='Search'][type='button'].button")
you will get a list of elements which can be accessed using this selector, if that list contains only one element (button that you want to click), then this selector is valid for your use..otherwise u'l have to try some other selector..
If any of the above selector is valid,u'l have to change your code accordingly..
driver.findElement(By.cssSelector("input[value='Search'][type='button'].button")).click();