可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have an asp.net solution using entity framework 6 to mysql server.
now I have to work on that solution on a new machine,
but I have some problems:
1) when building the solution I get error: "The ADO.NET provider with invariant name 'MySql.Data.MySqlClient' is either not registered in the machine or application config file".
App.config:
<entityFramework>
<providers>
<provider invariantName="MySql.Data.MySqlClient" type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6, Version=6.9.9.0, Culture=neutral, PublicKeyToken=c5687fc88969c44d"></provider>
</providers>
</entityFramework>
<system.data>
<DbProviderFactories>
<remove invariant="MySql.Data.MySqlClient" />
<add name="MySQL Data Provider" invariant="MySql.Data.MySqlClient" description=".Net Framework Data Provider for MySQL" type="MySql.Data.MySqlClient.MySqlClientFactory, MySql.Data, Version=6.9.9.0, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
</DbProviderFactories>
</system.data>
2) when opening model.edmx file I get message:
"the entity mode designer is unable to display the file you requested".
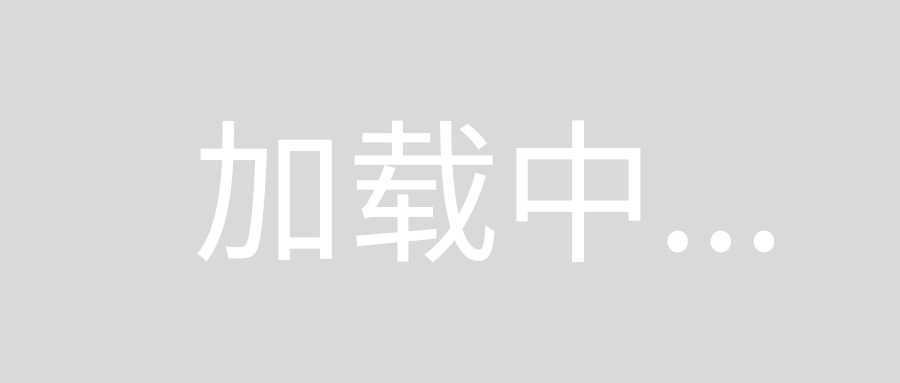
I have installed on the machine:
1) mysql connector/net 6.9.9
2) mysql for visual studio 1.2.6
3) mysql connector odbc 5.3.6.
what can I do?
回答1:
I followed most suggested solutions in the internet but for sorry all were failed. The problem occurs because visual studio lacks for MySql connector and I have solved it by installing mysql-connector-net-7.0.4 (https://downloads.mysql.com/archives/c-net/)
回答2:
I didn't have any MySql connector installed ony my machine.
Fixed it by installing nuget: MySql.ConnectorNET.Entity
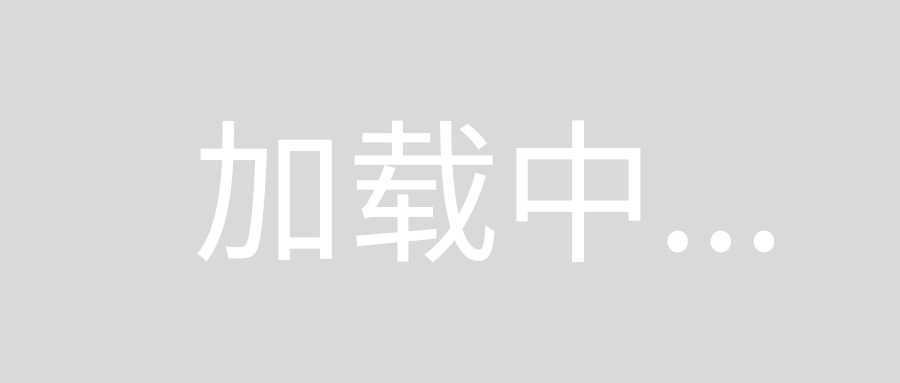
回答3:
It works for me with the following section in app.config:
<system.data>
<DbProviderFactories>
<add name="MySQL Data Provider" invariant="MySql.Data.MySqlClient" description=".Net Framework Data Provider for MySQL" type="MySql.Data.MySqlClient.MySqlClientFactory,MySql.Data" />
</DbProviderFactories>
</system.data>
回答4:
The above solutions didn't work for me. However, inspired by this answer, I was able to resolve this problem by removing version information under the entityFramework
tag in my Web.config
file:
<entityFramework>
<defaultConnectionFactory
type="MySql.Data.Entity.MySqlConnectionFactory, MySql.Data.Entity.EF6" />
<providers>
<provider invariantName="MySql.Data.MySqlClient"
type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6, Version=6.9.9.0, Culture=neutral, PublicKeyToken=c5687fc88969c44d">
</provider>
</providers>
</entityFramework>
becomes:
<entityFramework>
<defaultConnectionFactory
type="MySql.Data.Entity.MySqlConnectionFactory, MySql.Data.Entity.EF6" />
<providers>
<provider invariantName="MySql.Data.MySqlClient"
type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6">
</provider>
</providers>
</entityFramework>
ETA: As pointed out in this follow-up it may also be necessary to manually edit the version of MySql.Data
in the Web.config
file:
<dependentAssembly>
<assemblyIdentity name="MySql.Data" culture="neutral" publicKeyToken="c5687fc88969c44d" />
<bindingRedirect oldVersion="0.0.0.0-6.9.9.0" newVersion="6.9.9.0" />
</dependentAssembly>
回答5:
I have done lot of google and find the solution
Ensure MySQL.Data.dll, MySQL.Web.dll, MySQL.Data.Entity.dll, and System.Data.Entity.dll are all being copied local (right click on assembly and ensure Copy Local is set to true)
I also added the following locally as I tried to debug this problem but it was probably unnecessary
System.Data.dll [probably optional]
System.Data.Entity.Design [probably optional]
And here's the step you wont read about in many places on google or on AppHarbor!
Add the following to your web.config file:
<system.data>
<DbProviderFactories>
<clear />
<add name="MySQL Data Provider" invariant="MySql.Data.MySqlClient"
description=".Net Framework Data Provider for MySQL"
type="MySql.Data.MySqlClient.MySqlClientFactory, MySql.Data,
Version=6.4.4.0, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
</DbProviderFactories>
</system.data>
回答6:
In order to solve this problem, in addition to the changes outlined in this post, I also had to manually edit the version of MySql.Data
in web.config
like so:
<dependentAssembly>
<assemblyIdentity name="MySql.Data" culture="neutral" publicKeyToken="c5687fc88969c44d" />
<bindingRedirect oldVersion="0.0.0.0-6.9.9.0" newVersion="6.9.9.0" />
</dependentAssembly>
回答7:
Remove the old reference of MySql.Data.Entity.EF6 from the list of references. Reinstall via Nuget Package manager and it will work/
回答8:
You must add
codeConfigurationType="MySql.Data.Entity.MySqlEFConfiguration, MySql.Data.Entity.EF6"
to in your configuration file or call DbConfiguration.SetConfiguration(new MySqlEFConfiguration())
at the application start up.
回答9:
I was able to solve it by adding only the attribute "DbConfigurationType" to my context class, I found it in a codesample I had from somewhere, but can't recall the origin sorry. it is the same as Dmitry's answer but using an attribute instead as implementation
[DbConfigurationType(typeof(MySqlEFConfiguration))]
public class MySqlWeatherContext : DbContext
{
public MySqlWeatherContext() : base("context"){}
public DbSet<Measurepoint> Measurepoints { get; set; }
}
This is my config file:
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<configSections>
<section name="entityFramework" type="System.Data.Entity.Internal.ConfigFile.EntityFrameworkSection, EntityFramework, Version=6.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false" />
</configSections>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.7.2" />
</startup>
<connectionStrings>
<add name="context" providerName="MySql.Data.MySqlClient" connectionString="" />
</connectionStrings>
<entityFramework>
<defaultConnectionFactory type="System.Data.Entity.Infrastructure.SqlConnectionFactory, EntityFramework"/>
<providers>
<provider invariantName="System.Data.SqlClient" type="System.Data.Entity.SqlServer.SqlProviderServices, EntityFramework.SqlServer" />
<provider invariantName="MySql.Data.MySqlClient" type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6, Version=6.10.9.0, Culture=neutral, PublicKeyToken=c5687fc88969c44d" />
</providers>
</entityFramework>
</configuration>