I want to scale my y-var by multiplying it by a number, say 10, in ggplot. The problem is this is in a Shiny app and the variable must be passed as a character string, i.e. input$variable
.
How can I multiply one of the variables in aes_string()
the same way I would in aes()
? Here is an example of when it fails:
library(ggplot2)
ggplot(data = subset(mtcars, cyl == 4), aes_string(x = "wt", y = "mpg")) +
geom_line(size = 1.5, color = "#00868B") +
geom_line(data = subset(mtcars, cyl == 8), aes_string(x = "wt", y = "mpg" * 10))
Error in "mpg" * 10 : non-numeric argument to binary operator
You can use tidyeval
approach introduced in ggplot2 v3.0.0
# install.packages("ggplot2", dependencies = TRUE)
library(ggplot2)
var1 <- "wt"
var2 <- "mpg"
multiplier <- 10
ggplot(data = subset(mtcars, cyl == 4),
aes(x = !! rlang::sym(var1), y = !! rlang::sym(var2))) +
geom_line(size = 1.5, color = "#00868B") +
geom_line(data = subset(mtcars, cyl == 8),
aes(x = !! rlang::sym(var1), y = !! rlang::sym(var2) * multiplier))
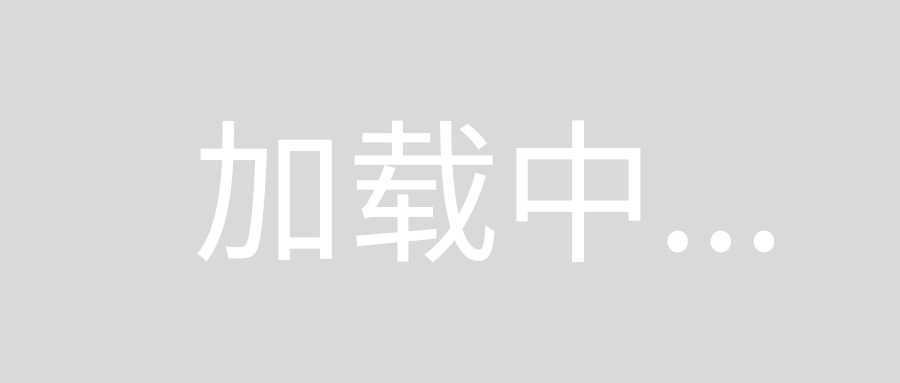
Or put everything in a function
plot_select_vars <- function(var1, var2, multiplier) {
var1 <- rlang::sym(var1)
var2 <- rlang::sym(var2)
ggplot(data = subset(mtcars, cyl == 4),
aes(x = !! var1, y = !! var2)) +
geom_line(size = 1.5, color = "#00868B") +
geom_line(data = subset(mtcars, cyl == 8),
aes(x = !! var1, y = !! var2 * multiplier))
}
plot_select_vars(var1, var2, multiplier)
Created on 2018-06-06 by the reprex package (v0.2.0).
I prefer to use get
instead of aes_string
to call variables inside ggplot2
and it works with value modification, for example:
library(ggplot2)
X <- "wt"
Y <- "mpg"
ggplot(subset(mtcars, cyl == 4), aes(get(X), get(Y))) +
geom_line() +
geom_line(data = subset(mtcars, cyl == 8), aes(y = get(Y) * 10)) +
labs(x = X,
y = Y)
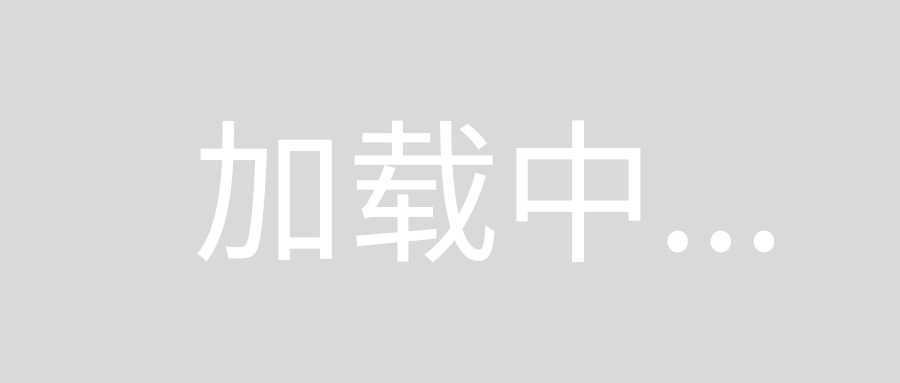
PS: you don't need to call wt
in second aes
as it's the same "variable" as in first aes
.