I'm having a problem using RxJS with Angular 2. Most of methods suggested from Typescript definition file are not defined on my Observable object like...
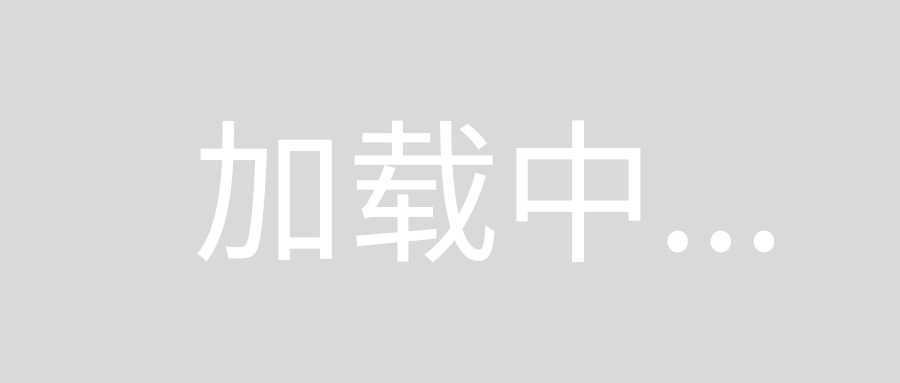
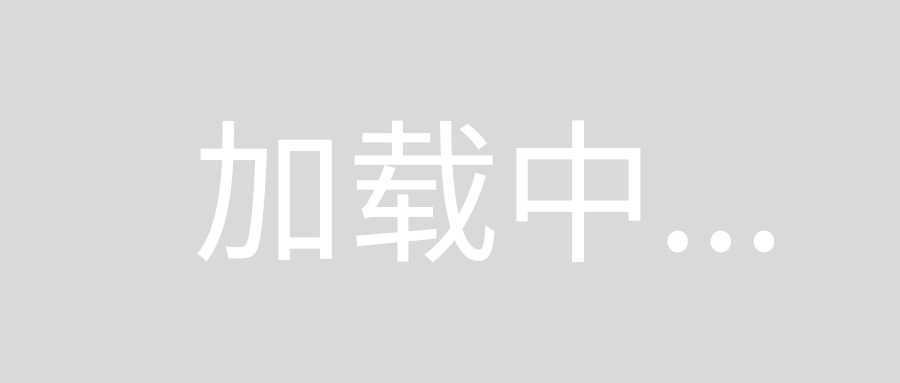
then I figured out, that methods does not exists on the Observable prototype.
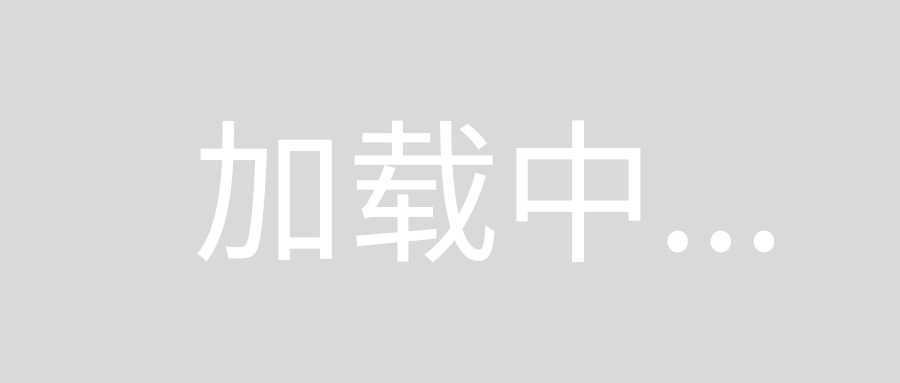
I know a lot of things changed from version 4 to 5,so do I miss something?
Browserify added it for me...
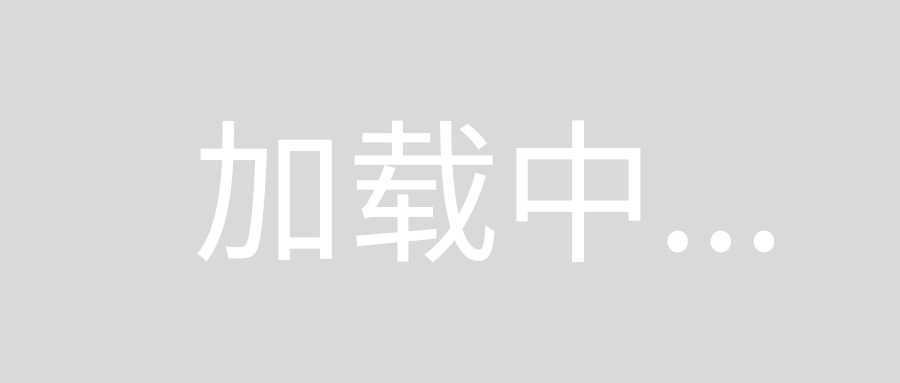
Without seeing your actual code, I can't tell you exactly what to add to fix it.
But the general problem is this: RxJS 5 is not included with Angular 2 any longer now that it has entered the Beta stage. You will need to import either the operator(s) you want, or import them all. The import statements looks like this:
import 'rxjs/add/operator/map'; // imports just map
import 'rxjs/add/operator/mergeMap'; // just mergeMap
import 'rxjs/add/operator/switchMap'; // just switchMap
import {delay} from 'rxjs/operator/delay'; // just delay
or like
import 'rxjs/Rx'; // import everything
To determine the path to your desired module, look at the source tree. Every import with add
will add properties to Observable
or Observable.prototype
. Without add
, you'd need to do import {foo} from 'rxjs/path/to/foo'
.
You will also need to make sure that RxJS is being brought into the project correctly. Something like this would go into your index.html file:
System.config({
map: {
'rxjs': 'node_modules/rxjs' // this tells the app where to find the above import statement code
},
packages: {
'app': {defaultExtension: 'js'}, // if your app in the `app` folder
'rxjs': {defaultExtension: 'js'}
}
});
System.import('app/app'); // main file is `app/app.ts`
If you use Webpack to build the Angular 2 app like in this Github project (like I did), then you don't need that System
stuff and the imports should do it.
Yes, in Angular 2.0 you have to include the operators/observables you need.
I do it like this:
import 'rxjs/operator/map';
import 'rxjs/operator/delay';
import 'rxjs/operator/mergeMap';
import 'rxjs/operator/switchMap';
import 'rxjs/observable/interval';
import 'rxjs/observable/forkJoin';
import 'rxjs/observable/fromEvent';
However, you also need to configure this in System.js
System.config({
defaultJSExtensions: true,
paths: {
'rxjs/observable/*' : './node_modules/rxjs/add/observable/*.js',
'rxjs/operator/*' : './node_modules/rxjs/add/operator/*.js',
'rxjs/*' : './node_modules/rxjs/*.js'
}
});
Here is working code: https://github.com/thelgevold/angular-2-samples
I have a JSPM setup in my project, so adding rxjs to the path section was not enough.
jspm added the following to my SystemJS configuration (map section):
"npm:angular2@2.0.0-beta.6": {
"crypto": "github:jspm/nodelibs-crypto@0.1.0",
"es6-promise": "npm:es6-promise@3.1.2",
"es6-shim": "npm:es6-shim@0.33.13",
"process": "github:jspm/nodelibs-process@0.1.2",
"reflect-metadata": "npm:reflect-metadata@0.1.2",
"rxjs": "npm:rxjs@5.0.0-beta.0",
"zone.js": "npm:zone.js@0.5.14"
},
So if you use jspm make sure you remove the rxjs mapping above, otherwise some rxjs files will be loaded twice, once via jspm_packages and once via node_modules.