- (IBAction)submitButtonClicked:(id)sender{
NSLog(@"here");
SuccessOrFailViewController *sfvc = [[SuccessOrFailViewController alloc] init];
[self presentViewController:sfvc animated:NO completion:NULL];
}
I am trying to open a new page when a user clicks the submit button in the app. However, this opens completely black screen with nothing on it. How do I make it open the viewController instead ?
You are not entering the nib name for the user Interface:
SuccessOrFailViewController *sfvc = [[SuccessOrFailViewController alloc] initWithNibName:@"SuccessOrFailViewController" bundle:nil];
[self presentViewController:sfvc animated:NO completion:NULL];
For storyboard this is the way to go:
UIStoryboard *storyboard = [UIStoryboard storyboardWithName:@"MainStoryboard" bundle:nil];
SuccessOrFailViewController *sfvc = [storyboard instantiateViewControllerWithIdentifier:@"SuccessOrFailViewController"];
[sfvc setModalPresentationStyle:UIModalPresentationFullScreen];
[self presentModalViewController:sfvc animated:YES];
Swift
var next = self.storyboard?.instantiateViewControllerWithIdentifier("DashboardController") as! DashboardController
self.presentViewController(next, animated: true, completion: nil)
don't forget to set ViewController StoryBoard Id
in StoryBoard
-> identity inspector
Incase anyone else finds this, make sure you haven't set self.view.backgroundColor = UIColor.clearColor()
in the view to be shown... This solved it for me.
For Storyboard iOS7
UIStoryboard *storyboard = [UIStoryboard storyboardWithName:@"MainStoryboard" bundle:nil];
SuccessOrFailViewController *sfvc = [storyboard instantiateViewControllerWithIdentifier:@"SuccessOrFailViewController"];
[sfvc setModalPresentationStyle:UIModalPresentationFullScreen];
[self presentViewController:sfvc animated:YES completion:nil];
I've had this happen after adding and deleting View Controllers in the Storyboard and forgetting to update the View Controller with the latest Storyboard identifier.
For example, if you are programmatically moving to a new View Controller, like this (note Identifier in the code is "FirstUserVC"):
let firstLaunch = (storyboard?.instantiateViewControllerWithIdentifier("FirstUserVC"))! as UIViewController
self.navigationController?.pushViewController(firstLaunch, animated: true)
The make sure in Interface Builder you have the Storyboard ID set like this with FirstUserVC listed in the Identity for Storyboard ID:
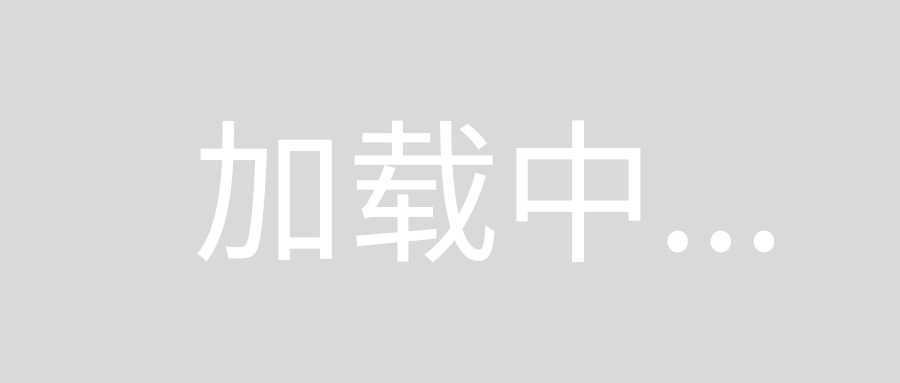