可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I'm trying to create my app component, but Dagger does not generate my app component.
here is MyApplication class
class MyApplication : Application() {
companion object {
@JvmStatic lateinit var graph: ApplicationComponent
}
@Inject
lateinit var locationManager : LocationManager
override fun onCreate() {
super.onCreate()
graph = DaggerApplicationComponent.builder().appModule(AppModule(this)).build()
graph.inject(this)
}
}
and here is my AppComponent class
@Singleton
@Component(modules = arrayOf(AppModule::class))
interface ApplicationComponent {
fun inject(application: MyApplication)
}
here is screenshot
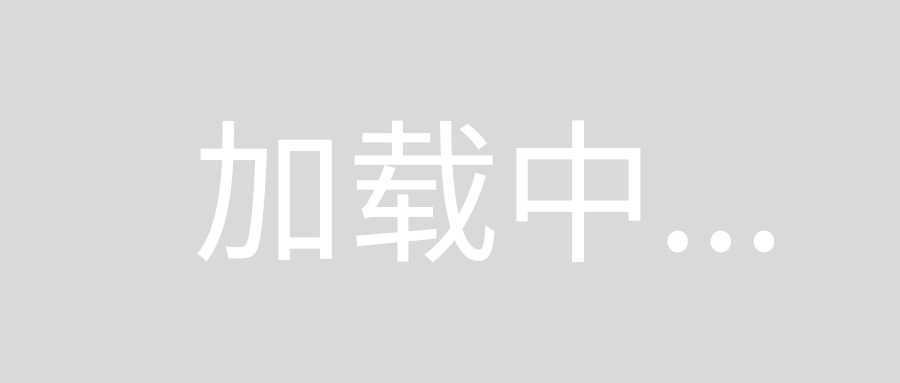
this is my project on github
here is error log
Error:(7, 48) Unresolved reference: DaggerApplicationComponent
Error:(28, 17) Unresolved reference: DaggerApplicationComponent
Error:Execution failed for task ':app:compileDebugKotlin'.
> Compilation error. See log for more details
Information:BUILD FAILED
Information:Total time: 21.184 secs
Error:e: .../MyApplication.kt: (7, 48): Unresolved reference: DaggerApplicationComponent
e: Unresolved reference: DaggerApplicationComponent
FAILURE: Build failed with an exception.
* What went wrong:
Execution failed for task ':app:compileDebugKotlin'.
> Compilation error. See log for more details
* Try:
Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output.
Information:4 errors
Information:0 warnings
Information:See complete output in console
回答1:
my solution is to add
apply plugin: 'kotlin-kapt'
and remove
kapt {
generateStubs = true
}
回答2:
This helped me to fix this issue
Add this in the top of the build.gradle
apply plugin: 'kotlin-kapt'
Inside android
tag add
kapt {
generateStubs = true
}
And then replace
annotationProcessor 'com.google.dagger:dagger-compiler:2.11'
to
kapt 'com.google.dagger:dagger-compiler:2.11'
Now Rebuild
the project by
Build -> Rebuild project
回答3:
Please try enabling stubs generation, this might be the reason why the class is not visible at this stage of the build process. In your build.gradle
file, top level:
kapt {
generateStubs = true
}
回答4:
I've already downloaded your Github project. Thanks for sharing!
The answer for your issue is pretty simple:
Build -> Rebuild project
Dagger dependencies files will be recreated and app after would launched with any problem.
I checked already this with Android Studio 2.1.2 version. It works
回答5:
you should remove
kapt {
generateStubs = true}
and add to the top of application gradle file
apply plugin: 'kotlin-kapt'
then Dagger will take care of the rest :)
回答6:
Also for Java users, the solution is simply to Rebuild your project.
回答7:
In my case missing kapt compiler dependency. Please make sure to have below dependency too.
kapt 'com.google.dagger:dagger-compiler:2.15'
app build.gradle
apply plugin: 'com.android.application'
apply plugin: 'kotlin-android'
apply plugin: 'kotlin-kapt'
apply plugin: 'kotlin-android-extensions'
android {
compileSdkVersion 27
defaultConfig {
applicationId "com.usb.utility"
minSdkVersion 14
targetSdkVersion 27
versionCode 1
versionName "1.0"
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
implementation "org.jetbrains.kotlin:kotlin-stdlib-jre7:$kotlin_version"
implementation 'com.android.support:appcompat-v7:27.1.0'
implementation 'com.android.support.constraint:constraint-layout:1.0.2'
compile 'com.google.dagger:dagger-android:2.15'
compile 'com.google.dagger:dagger-android-support:2.15' // if you use the support libraries
kapt 'com.google.dagger:dagger-android-processor:2.15'
kapt 'com.google.dagger:dagger-compiler:2.15'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'com.android.support.test:runner:1.0.1'
androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.1'
}
回答8:
Only two steps should be required for mixed Java/Kotlin projects:
- Add
apply plugin: 'kotlin-kapt'
to the top of the build file
- Replace
annotationProcessor 'com.google.dagger:dagger-compiler:2.14.1'
with kapt 'com.google.dagger:dagger-compiler:2.14.1'
回答9:
Answer is simple :
Build -> Rebuild project
It works for me.
回答10:
Add this on Top of build.gradlle
apply plugin: 'kotlin-kapt'
Add this in Dependencies
kapt 'com.google.dagger:dagger-compiler:2.15'
kapt 'com.google.dagger:dagger-android-processor:2.15'
After that rebuild your project . i hope it will work.
Thanks
回答11:
Please try adding this to your build.gradle
android {
dexOptions {
incremental false
}
EDIT: apparently a year later this can happen if you don't apply kotlin-kapt
plugin. Also make sure you use kapt
instead of annotationProcessor
.