How can I make an underlined text in UILabel
?
I had tried by making a UILabel
with height 0.5f and place it under the text. This line label is not visible when I am running my app in iPhone 4.3 and iPhone 4.3 simulator, but it is visible in iPhone 5.0 simulator onwards. Why?
How can I do this?
Objective-C
iOS 6.0 > version
UILabel
supports NSAttributedString
NSMutableAttributedString *attributeString = [[NSMutableAttributedString alloc] initWithString:@"Hello Good Morning"];
[attributeString addAttribute:NSUnderlineStyleAttributeName
value:[NSNumber numberWithInt:1]
range:(NSRange){0,[attributeString length]}];
Swift
let attributeString: NSMutableAttributedString = NSMutableAttributedString(string: "Hello Good Morning")
attributeString.addAttribute(NSUnderlineStyleAttributeName, value: 1, range: NSMakeRange(0, attributeString.length))
Definition :
- (void)addAttribute:(NSString *)name value:(id)value range:(NSRange)aRange
Parameters List:
name : A string specifying the attribute name. Attribute keys can be supplied by another framework or can be custom ones you define. For information about where to find the system-supplied attribute keys, see the overview section in NSAttributedString Class Reference.
value : The attribute value associated with name.
aRange : The range of characters to which the specified attribute/value pair applies.
Now use like this:
yourLabel.attributedText = [attributeString copy];
iOS 5.1.1 < version
You needs 3 party attributed Label
to display attributed text
:
1) Refer TTTAttributedLabel link. Its best third party attributed
Label
to display
attributed text
.
2) refer OHAttributedLabel for third party attributed
Label
I am using Xcode 9 and iOS 11.
To make the UILabel with an underline beneath it.
You can use both
1. Using code
2. Using xib
Using code:
NSMutableAttributedString *attributeString = [[NSMutableAttributedString alloc] initWithString:@"I am iOS Developer"];
[attributeString addAttribute:NSUnderlineStyleAttributeName
value:[NSNumber numberWithInt:1]
range:(NSRange){0,[attributeString length]}];
lblAttributed.attributedText = attributeString;
Using Xib:
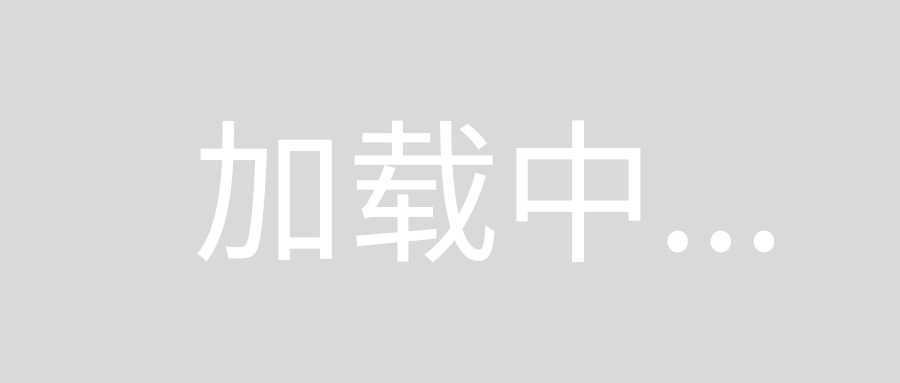
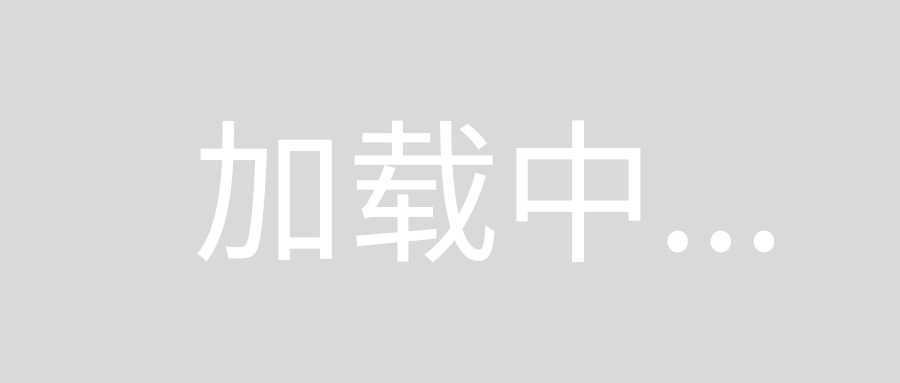
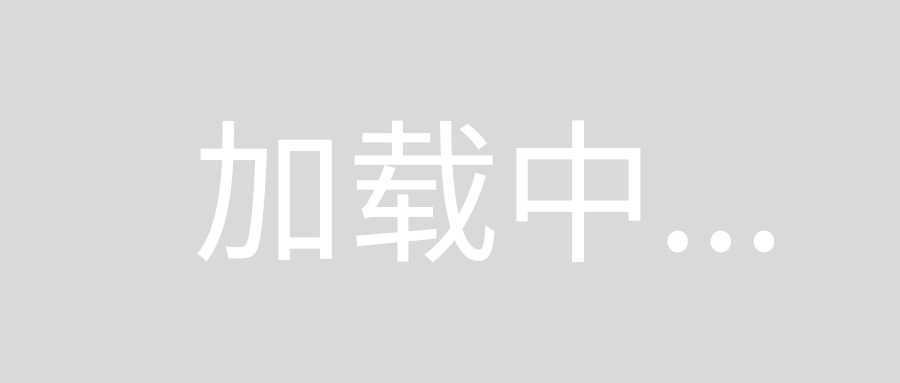
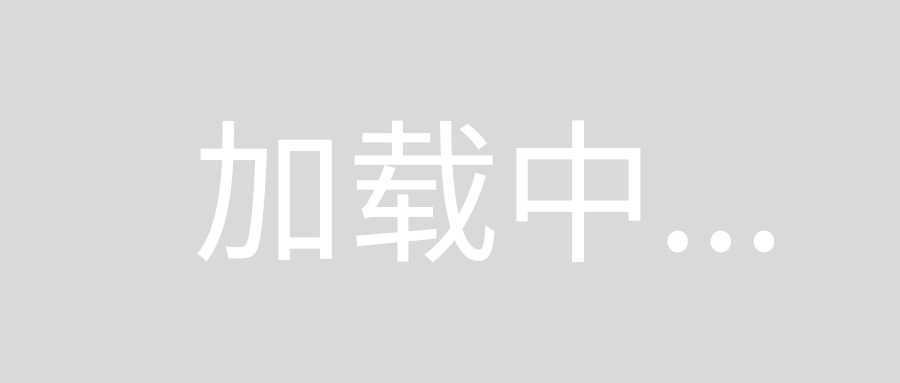
We can also use for button too.
The Designer Way - Highly Customisable Version
I personally prefer to customise my design(s) as much as possible. Sometimes, you will find that using the built-in underline tool is not easily customisable.
Here is how I do it:
1 First I create a UIView for the underline. You can then choose your background color, either in IB or programmatically. Constraints are key here.
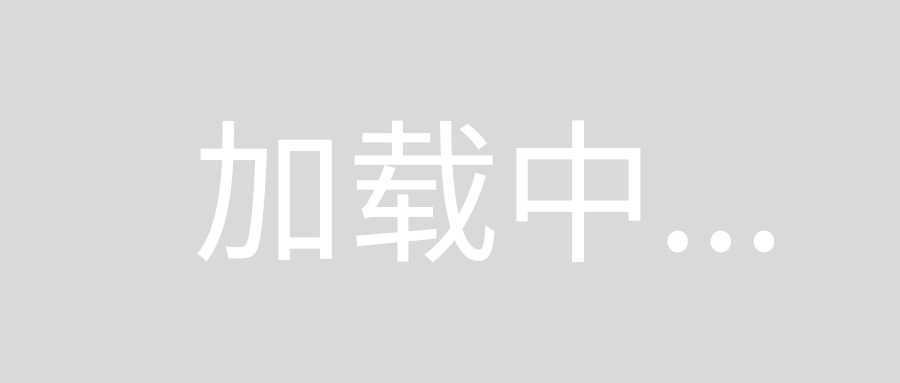
2 Then, you can customise the underline as much as you like. For example, to adapt the width of the underline for better visual effect, create an outlet connection to a constraint in Interface Builder
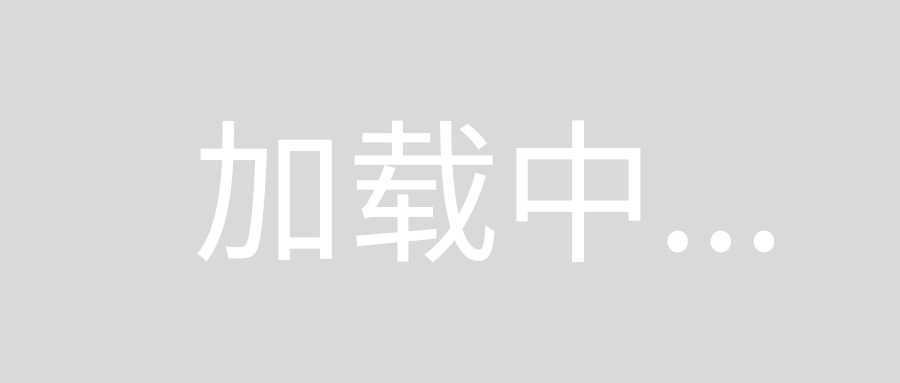
3 You can then easily programmatically customise your underline view. For example here, to give you an idea we will make the underline 20 px wider than the title "Breads":
var originalString: String = "Breads"
let myString: NSString = originalString as NSString
let size: CGSize = myString.size(attributes: [NSFontAttributeName: UIFont.systemFont(ofSize: 14.0)])
self.widthUnderlineTitle.constant = size.width + 20
Results:
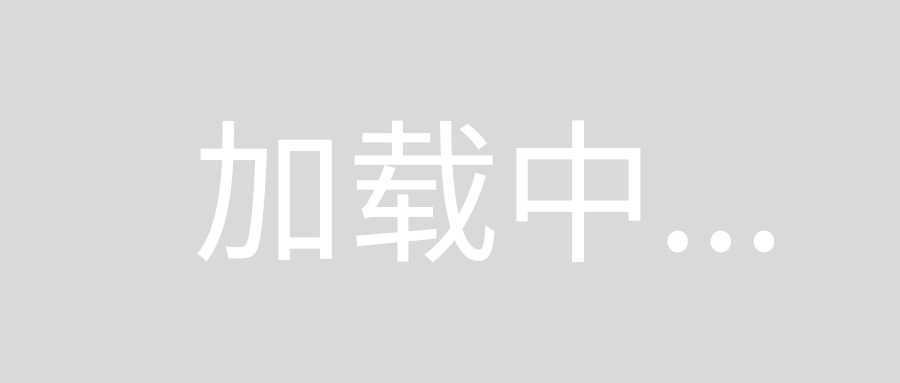
Take a look at TTTAttributedLabel. If you're allowed to use 3d-party components just replace your UILabels with TTTAttributedLabel where you need it (drop-in replacement). Works with iOS < 6.0!
Swift extension on UILabel:
Still needs improvement, any thoughts are welcome:
extension UILabel{
func underLine(){
if let textUnwrapped = self.text{
let underlineAttribute = [NSUnderlineStyleAttributeName: NSUnderlineStyle.StyleSingle.rawValue]
let underlineAttributedString = NSAttributedString(string: textUnwrapped, attributes: underlineAttribute)
self.attributedText = underlineAttributedString
}
}
}
One of the cons i can think of right now is that it will need to be called each time text changes, and there is no actual way of turning it off...
Swift 4.0
var attributeString = NSMutableAttributedString(string: "Hello Good Morning")
attributeString.addAttribute(NSUnderlineStyleAttributeName, value: 1, range: NSRange)
Swift 5
let attributeString: NSMutableAttributedString = NSMutableAttributedString(string: yourLabel.text ?? "")
attributeString.addAttribute(NSAttributedString.Key.underlineStyle, value: 1, range: NSMakeRange(0, attributeString.length))
yourLabel.attributedText = attributeString