I'm having a problem with regards of implementing a resolver on my routes as it has no issue until I include InitialDataResolver
on my routing module.
pages-routing.module.ts
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { FrontComponent } from '../layouts/front/front.component';
import { HomeComponent } from './home/home.component';
import { DocsComponent } from './docs/docs.component';
import { InitialDataResolver } from './../shared/resolvers/initial-data.resolver';
const routes: Routes = [
{
path: '',
component: FrontComponent,
children: [
{ path: '', component: HomeComponent },
{ path: 'docs', component: DocsComponent }
],
resolve: {
init: InitialDataResolver
},
}
];
@NgModule({
imports: [ RouterModule.forChild(routes) ],
exports: [ RouterModule ],
providers: [ InitialDataResolver ]
})
export class PagesRoutingModule { }
initial-data.resolver.ts
import { Injectable } from '@angular/core';
import { ActivatedRouteSnapshot, Resolve, RouterStateSnapshot } from '@angular/router';
import { Observer } from 'rxjs/Observer';
import { AppInitService } from '../services/app-init.service';
import { Observable } from 'rxjs/Observable';
@Injectable()
export class InitialDataResolver implements Resolve<any> {
constructor(private appInitService: AppInitService) {}
resolve(route: ActivatedRouteSnapshot,
state: RouterStateSnapshot): Observable<any> {
return Observable.create((observer: Observer<any>) => {
this.appInitService.init()
.subscribe(data => {
this.appInitService.preload();
observer.next(data);
observer.complete();
});
});
}
}
The error that I'm encountering is ERROR Error: "[object Object]"
. see the snapshot below:
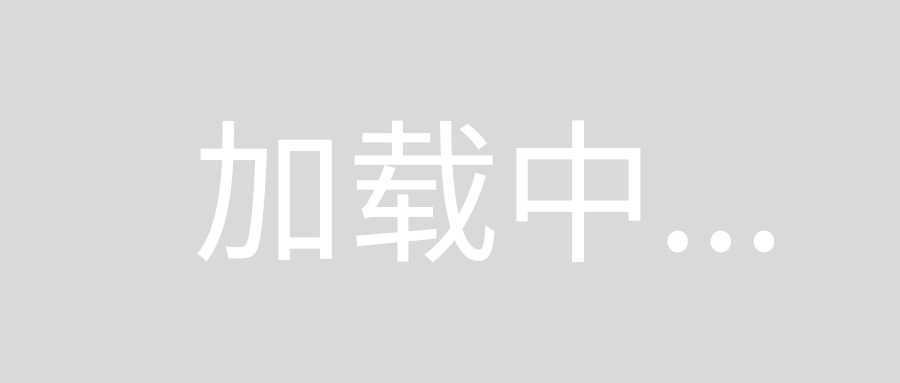
This lack of detailed error occurred when using Mozilla Firefox
. so what you need to do is to switch over to Google Chrome
to see the specific error.
UPDATED:
You can also Store the error as Global Variable
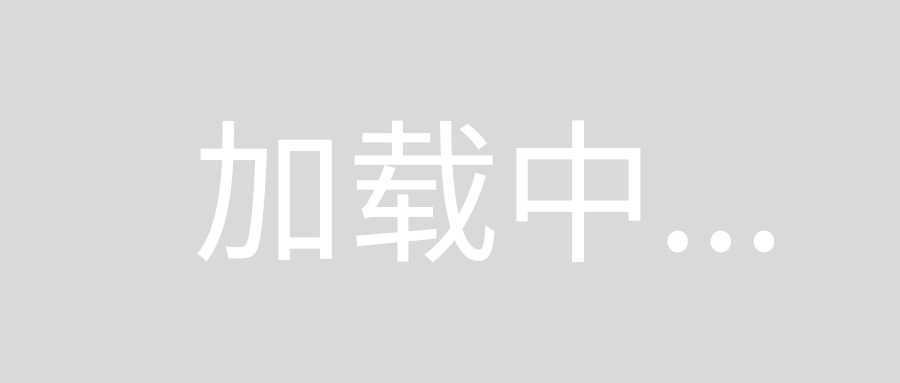
then you can type temp0.message
to see the actual error message
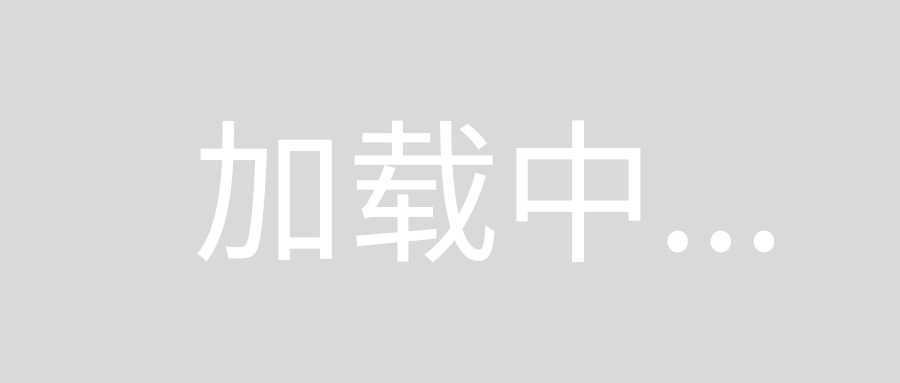
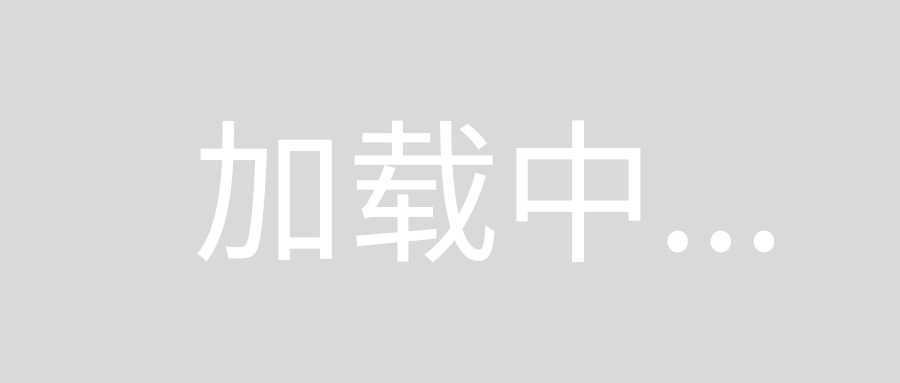
In console you can store errors like
ERROR Error: "[object Object]"
as global variable
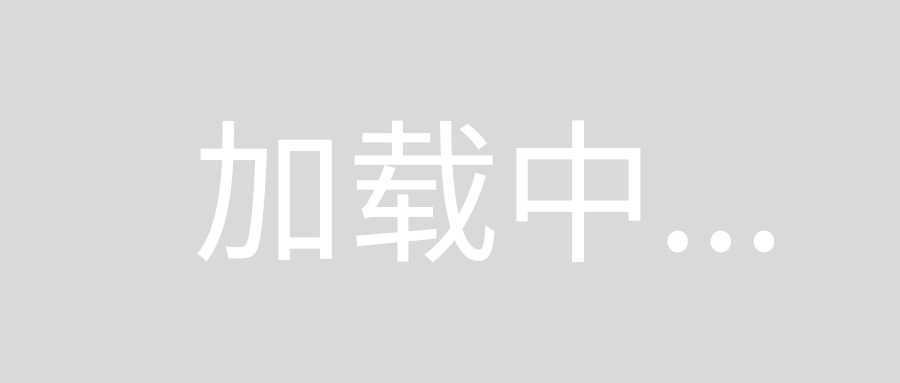
and then get error message from object temp0.message
I have found solution for detailed error working in firefox. Its based on defining custom error handler and inspecting error properties yourself.
After these steps, the errors were displayed properly, no need to switch to chrome.
- Define custom error handler class:
import { ErrorHandler } from '@angular/core'
export class MyErrorHandler implements ErrorHandler {
handleError(error: any) {
// console.error(Object.getOwnPropertyNames(error))
// Object.getOwnPropertyNames(error).forEach(p => console.error(error[p]))
console.error(error.fileName, error.lineNumber, ':', error.columnNumber, '\n', error.message, error.rejection)
}
}
- Then register it as provider in main component
@NgModule({
declarations: []
imports: []
providers: [{provide: ErrorHandler, useClass: MyErrorHandler}], // <-- register MyErrorHandler
bootstrap: [RootComponent]
})
export class AppModule { }
resolve(route: ActivatedRouteSnapshot,
state: RouterStateSnapshot): Observable<any> {
return Observable.create((observer: Observer<any>) => {
this.appInitService.init()
.subscribe(data => {
this.appInitService.preload();
observer.next(data);
observer.complete();
});
}).map(item => !!item)
}
The code above will work for you.
if your rxjs version is 6.x you will use .pipe(map(item => !!item))
This error comes due to wrong router declaration. Also exact error never going to come in Firefox. Check route file in chrome. #angularjs-route
The error could hide details on firefox if you build without --prod and host in web server, try to build with --prod if you are hosting on a web server to get details about the error.
one-time solution :
find function defaultErrorLogger in dist/vendor.bundle.js and add:
for (var _i = 1; _i < arguments.length; _i++) {
values[_i - 1] = arguments[_i];
}
console.log(arguments);
console.error.apply(console, values); code here
then refresh page without recompiling
Mainly this error is found when using database object from '@angular/fire'
this can be fixed by using this.db.object('/' + ....).subscribe(...)