可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have successfully built my Spring MVC project with mvn clean package
by following this tutorial.
Now I am trying to run the service with:
mvn clean package && java -jar target/gs-serving-web-content-0.1.0.jar
But I get this error:
Failed to load Main-Class manifest attribute from
target/gs-serving-web-content-0.1.0.jar
Am I missing something?
回答1:
If you are working with Spring Boot this will solve your problem:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>1.2.5.RELEASE</version>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
Reference Guide | Spring Boot Maven Plugin
回答2:
You might be missing Spring Boot Maven Plugin.
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
回答3:
You have to specifiy it in your pom.xml - This will make your jar executable with all dependencies (replace your.main.class
):
<!-- setup jar manifest to executable with dependencies -->
<plugin>
<artifactId>maven-assembly-plugin</artifactId>
<configuration>
<descriptorRefs>
<descriptorRef>jar-with-dependencies</descriptorRef>
</descriptorRefs>
<archive>
<manifest>
<mainClass>your.main.class</mainClass>
</manifest>
</archive>
</configuration>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>single</goal>
</goals>
</execution>
</executions>
</plugin>
回答4:
check the order of
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
it should be above
dockerfile-maven-plugin else repackage will happen
this solved my problem of no main attribute in manifest.
回答5:
You are missing maven-jar-plugin
in which you need to add manifest
tag.
回答6:
For spring boot, I've created a MANIFEST.MF file inside META-INF folder.
in my STS IDE, I placed the META-INFO folder inside the src/main/resources folder
like so:
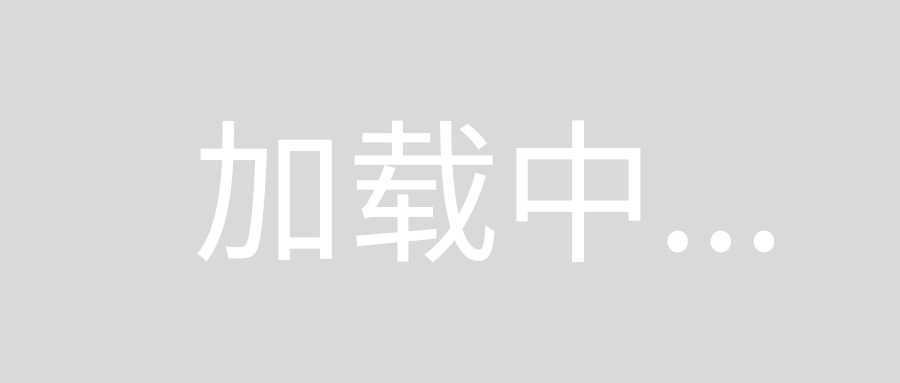
the content of the MANIFEST.MF file:
Manifest-Version: 1.0
Implementation-Title: bankim
Implementation-Version: 1.5.6.RELEASE
Archiver-Version: Plexus Archiver
Built-By: Yourname
Implementation-Vendor-Id: com.bankim
Spring-Boot-Version: 1.5.6.RELEASE
Implementation-Vendor: Pivotal Software, Inc.
Main-Class: org.springframework.boot.loader.JarLauncher
Start-Class: com.bankim.BankimApplication
Spring-Boot-Classes: BOOT-INF/classes/
Spring-Boot-Lib: BOOT-INF/lib/
Created-By: Apache Maven 3.3.9
Build-Jdk: 1.8.0_131
Implementation-URL: http://projects.spring.io/spring-boot/bankim/
- Every mention of "bankim"/"Bankim" refers to my project name, so replace it with your project name that
- take special note of the "Start-Class" value. it should contain the "path" to the class that has your main method.
- the row:
Main-Class: org.springframework.boot.loader.JarLaunchershould be left as-is.
****the above manifest was created for me by using the "spring-boot-maven-plugin" mentioned above by "Mradul Pandey" (answered Sep 2 '15 at 4:50)
Hope this helps
回答7:
I had the spring-boot-maven-plugin
but still I was getting the error regarding main class.
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
Finally I had to use the maven-jar-plugin
and add the mainClass
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>2.4</version>
<configuration>
<archive>
<manifest>
<mainClass>com.org.proj.App</mainClass>
</manifest>
</archive>
</configuration>
</plugin>
And it was good to go!
回答8:
Use spring-boot-maven-plugin
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
and be sure you set property start-class
in the pom.xml
<properties>
<!-- The main class to start by executing "java -jar" -->
<start-class>org.example.DemoApplication</start-class>
</properties>
Alternatively, the main class can be defined as the mainClass element of the spring-boot-maven-plugin in the plugin section of your pom.xml:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
<configuration>
<classifier>spring-boot</classifier>
<mainClass>${start-class}</mainClass>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>