I'm looking for an easy way to query my database in firebase using swift to retrieve a random object. I've read a lot of threads and there doesn't seem to be an easy way. One example showed it can be done be creating a sequential number but there's no information on how to create this sequential number for each record.
So either I need information on how to create a sequential number each time a record is created or if someone knows an easy way to retrieve a random record from a database that would be very helpful. In swift preferably.
My Database structure:
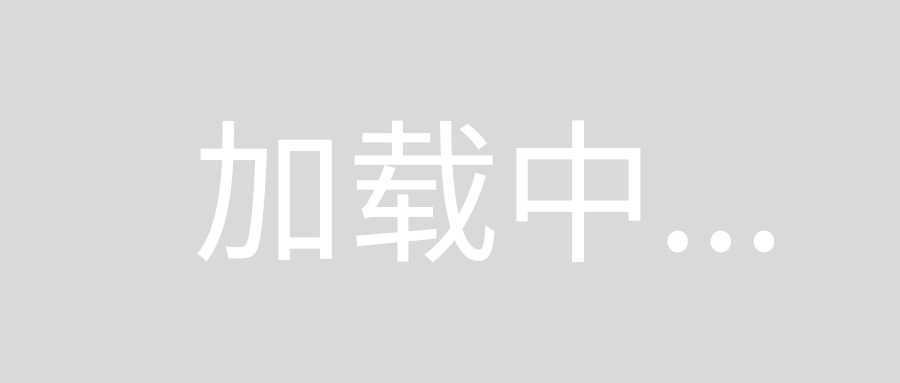
QUERY RANDOM OBJECT IN FIREBASE < VERY SIMPLE SOLUTION > SWIFT 4
One thing that you could try is to restructure your data like this:
- profiles
- 1jon2jbn1ojb3pn231 //Auto-generated id from firebase.
- jack@hotmail.com
- oi12y3o12h3oi12uy3 //Auto-generated id from firebase.
- susan@hotmail.com
- ...
Firebase's auto-generated id's are sorted in lexicographical order by key, when they are sent to Firebase, so you can easily create a function like this:
func createRandomIndexForFirebase() -> String {
let randomIndexArray = ["a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z","0","1","2","3","4","5","6","7","8","9"]`
let randomIndex = Int.random(in: 0..<randomIndexArray.endIndex)
//In lexicographical order 'A' != 'a' so we use some clever logic to randomize the case of any letter that is chosen.
//If a numeric character is chosen, .capitalized will fail silently.
return (randomIndex % 2 == 0) ? randomIndexArray[randomIndex] : randomIndexArray[randomIndex].capitalized
}
Once you get a random index you can create a firebase query to grab a random profile.
var ref: DatabaseReference? = Database.database().reference(fromURL: "<DatabaseURL>")
ref?.child("profiles").queryOrderedByKey().queryStarting(atValue: createRandomIndexForFirebase()).queryLimited(toFirst: 1).observeSingleEvent(of: .value, with: { snapshot in
//Use a for-loop in case you want to set .queryLimited(toFirst: ) to some higher value.
for snap in snapshot.children {
guard let randomProfile = snap as? DataSnapshot else { return }
//Do something with your random profiles :)
}
}
Database.database().reference().child("profiles").observeSingleEvent(of: .value) { (snapshot) in
if let snapshots = snapshot.children.allObjects as? [DataSnapshot] {
// do random number calculation
let count = snapshots.count
return snapshots[Int(arc4random_uniform(UInt32(count - 1)))]
}