I have a problem with the color of my UITabBarItems when I run on iOS 13 simulators, using Xcode 11, beta 2. I have made a sample project from scratch, and everything works correctly when I do not specify a bar tint color. However, when I do specify a custom bar tint color via Interface Builder, I get this:
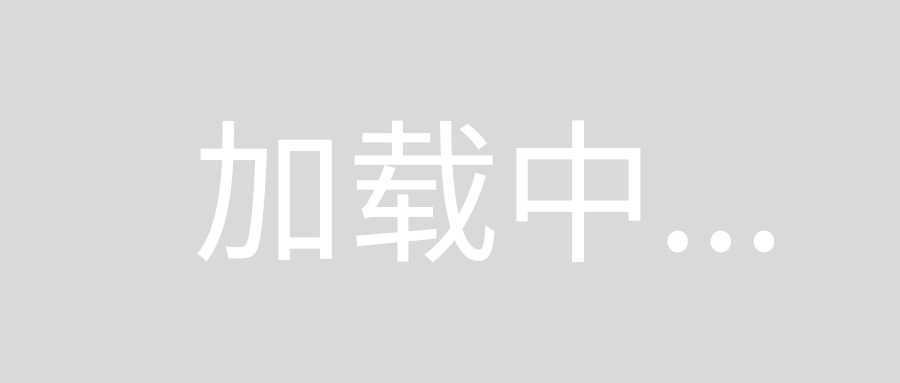
All items icons in the tab bar have the selected color if I set the "Bar Tint" property in Interface Builder to anything but clear. When it is set to clear, the icons are colored properly. The icons are also colored properly if I compile and run in an iOS 12 simulator.
This seems like a bug in Xcode 11, but maybe I'm missing something?
On the surface, this might seem like a bug, however you can mitigate it by defining an .unselectedItemTintColor on your UITabBar instance.
self.tabBar.unselectedItemTintColor = [UIColor lightGrayColor];
There is a new appearance API in iOS 13. To color tabbar item's icon and text correctly using Xcode 11.0 you can use it like this:
if #available(iOS 13, *) {
let appearance = UITabBarAppearance()
appearance.backgroundColor = .white
appearance.shadowImage = UIImage()
appearance.shadowColor = .white
appearance.stackedLayoutAppearance.normal.iconColor = .black
appearance.stackedLayoutAppearance.normal.titleTextAttributes = [NSAttributedString.Key.foregroundColor: UIColor.black]
appearance.stackedLayoutAppearance.selected.iconColor = .red
appearance.stackedLayoutAppearance.selected.titleTextAttributes = [NSAttributedString.Key.foregroundColor: UIColor.red]
self.tabBar.standardAppearance = appearance
}
Use the attribute field "Image Tint" in IB.
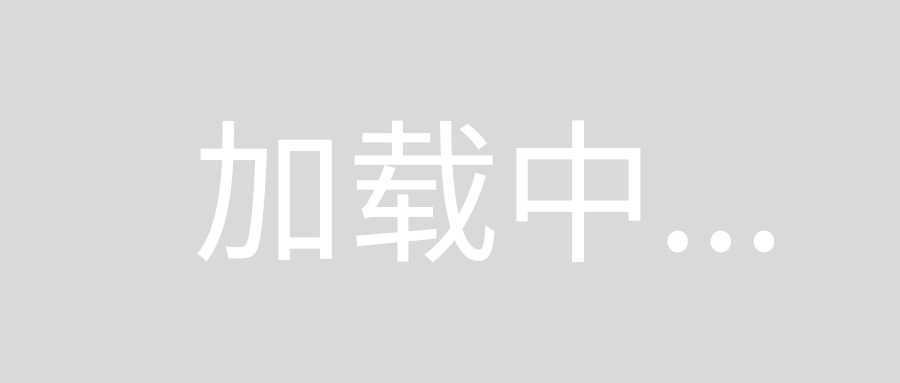
Apple's own Podcasts App has the same issue. This is a bug currently.
For Objective C you can use in AppDelegate:
[[UITabBar appearance] setTintColor:[UIColor whiteColor]];
[[UITabBar appearance] setUnselectedItemTintColor:[UIColor colorWithRed:255.0f/255.0f green:255.0f/255.0f blue:255.0f/255.0f alpha:0.65f]];
self.tabBarController?.tabBar.unselectedItemTintColor = UIColor.lightGray
This works for me in swift 4. Just put this in the override func viewWillDisappear(_ animated: Bool)
method and this will update as the view is changing.
So it will look something like this
override func viewWillDisappear(_ animated: Bool) {
self.tabBarController?.tabBar.unselectedItemTintColor = UIColor.lightGray
}
Even if this is a bug(I'm not sure) you can use this to change the color of the tab bar item by using any color of you choice