I have the following code in my service
myService.ts
makeHttpGetRequest(url){
return Observable.interval(config.SUPERVISOR_REFRESH_INTERVAL * 1000)
.switchMap(() => this.http.get(url))
.map(res => res.json())
.timeout(config.REQUEST_TIMEOUT * 1000, new Error('Time out occurred'))
}
In my component file,
myComponent.ts
ngOnInit(){
this._myService.makeHttpGetRequest(myurl)
.subscribe(
data => {
this.supervisorServers = data;
}
},
error => {
this.error = true;
console.log(error); //gives an object at this point
this.showError(error);
}
);
}
I want to print the error message in case of for e.g. Invailid url.
When I print the error, I get an object (probably response object) like the following:
Object { _body: error, status: 200, statusText: "Ok", headers: Object, type: 3, url: null }
If I open this, I cannot find the error message. Is there a better way to get precies error messages?
The error is contained in the body of the response even in this case. See the error event handler onError function:
- https://github.com/angular/angular/blob/master/modules/@angular/http/src/backends/xhr_backend.ts#L96
You can access it using the json method on the response:
error => {
this.error = true;
console.log(error.json()); //gives the object object
this.showError(error.json());
}
Edit
I investigated a bit more this issue. In fact, you can't have the exact message. I mean the net::ERR_NAME_NOT_RESOLVED
. XHR doesn't provide it. That said, you can see that the status
of XHR is 0
. This could be an hint that there was a problem the request when sending the request (it's not actually sent).
error => {
(...)
var err = error.json();
var status = err.currentTarget.status;
(...)
}
See this question:
- XMLHttpRequest() & net::ERR_NAME_NOT_RESOLVED
I try error.error and work to me. Its get the Object message error.
EDIT:
Sorry!!
Try this:
error => {
this.error = true;
console.log(error.error);
this.showError(error.error);
}
Using Angular 5+ you can actually just use error.message
.
error => {
console.log(error.message);
this.showError(error.message);
}
In my case I am using Ionic 3 with an alert
error => {
loading.dismiss();
let alert = this.alertCtrl.create({
title: 'Error: ',
message: error.message,//Displays http error message in alert
buttons: [
{
text: 'Dismiss',
role: 'Yes',
handler: () => {}
}
]
});
alert.present();
}
Here is a snippet of the error object in the console when logged:
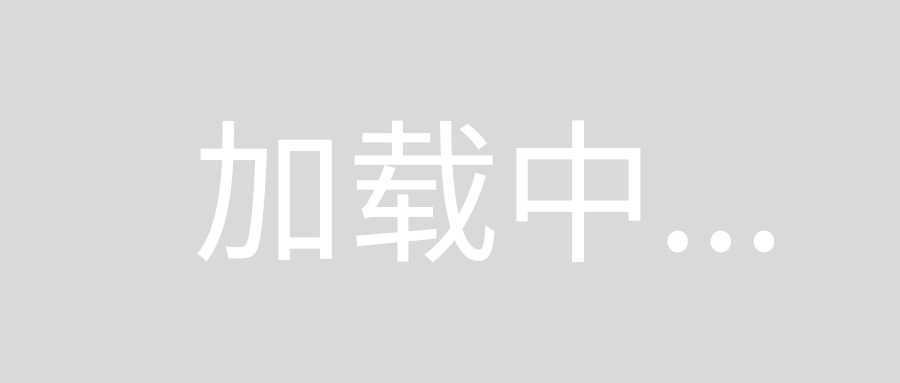
You can stringify the error object to print its string value
const errStr = JSON.stringify(error);
console.log(errStr);