I want to reduce the size between cells in row.
Now it looks like:
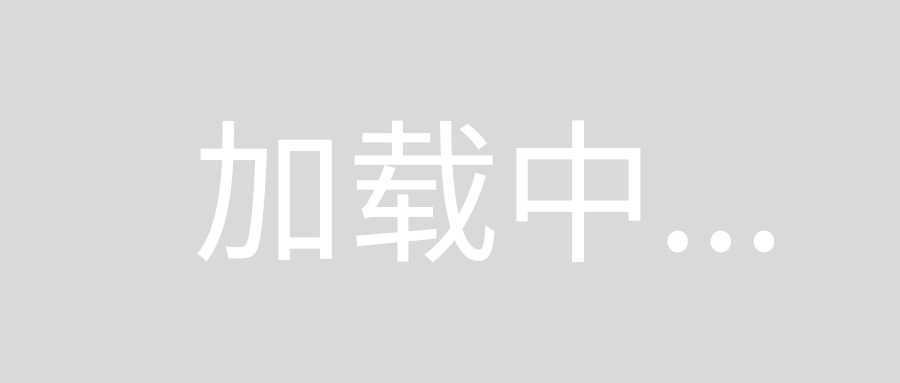
I'm trying this, for reduce the size between them:
let layout: UICollectionViewFlowLayout = UICollectionViewFlowLayout()
layout.sectionInset = UIEdgeInsets(top: 20, left: 2, bottom: 10, right: 2)
layout.minimumInteritemSpacing = 0
layout.minimumLineSpacing = 0
but it doesn't help me. What I do wrong?
//Objective c
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath {
return CGSizeMake((collectionView.frame.size.width/3)-20, 100);
}
// Swift 2.0
func collectionView(collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAtIndexPath indexPath: NSIndexPath) -> CGSize {
return CGSizeMake((collectionView.frame.size.width / 3) - 20, 100)
}
// Swift 3.0
override func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: CGFloat((collectionView.frame.size.width / 3) - 20), height: CGFloat(100))
}
Go to the storyboard , right click on collection view and 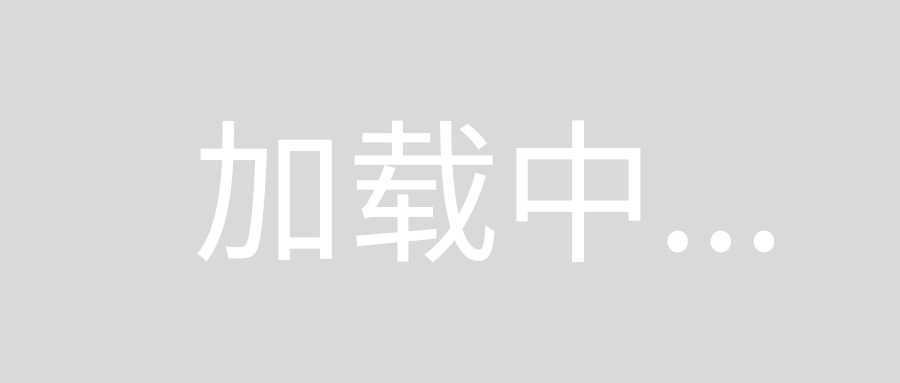
And Check your minimum spacing between the cell and reduce it to zero .
On your code, you have to create a IBOutlet to your collection view, and then assign the collectionViewLayout as such:
let layout: UICollectionViewFlowLayout = UICollectionViewFlowLayout()
layout.sectionInset = UIEdgeInsets(top: 20, left: 2, bottom: 10, right: 2)
layout.minimumInteritemSpacing = 0
layout.minimumLineSpacing = 0
collectionView!.collectionViewLayout = layout
Its simple
let layout = contactsCollectionView.collectionViewLayout as? UICollectionViewFlowLayout
layout?.minimumLineSpacing = 8
Swift 5 Programmatically
lazy var collectionView: UICollectionView = {
let layout = UICollectionViewFlowLayout()
layout.scrollDirection = .horizontal
//Provide Width and Height According to your need
let width = UIScreen.main.bounds.width / 4
let height = UIScreen.main.bounds.height / 10
layout.itemSize = CGSize(width: width, height: height)
//Make Space as Zero
layout.minimumInteritemSpacing = 0
layout.minimumLineSpacing = 0
return UICollectionView(frame: self.view.frame, collectionViewLayout: layout)
}()
You can provide much more customization inside the lazy collectionView
Block.
If you create CollectionView
In StoryBoard
or Xib
,Go to StoryBoard
or Xib
and set these Values from
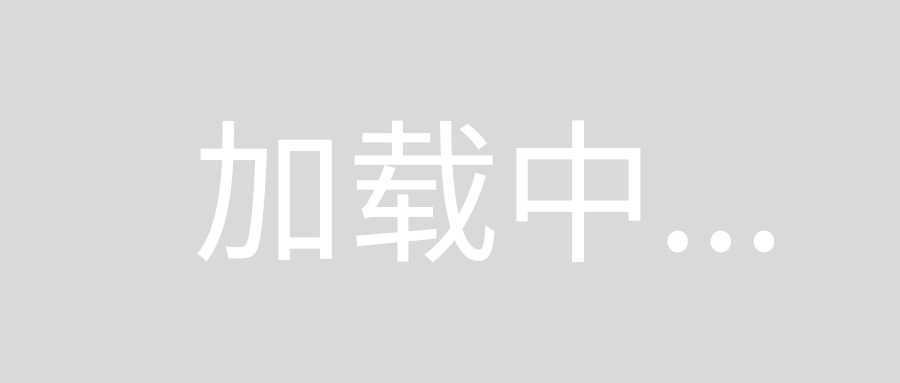
extension ViewController: UICollectionViewDelegateFlowLayout {
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
let collectionWidth = collectionView.bounds.width
return CGSize(width: collectionWidth, height: collectionWidth/2)
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumLineSpacingForSectionAt section: Int) -> CGFloat {
return 0
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, minimumInteritemSpacingForSectionAt section: Int) -> CGFloat {
return 100
}
}