In my view controller, I have an UITextView. This textview is filled with a string. The string can be short or long. Depending on the length of the string, the height of the textview has to adjust.
I use storyboards and auto layout.
I have some troubles with the height of the textview.
Sometimes, the height is perfectly adjusted to the text. Sometimes, no, the text is cropped on the first line. Below, the textview is yellow. the blue is a containerview inside a scrollview. Purple is the place for a picture.
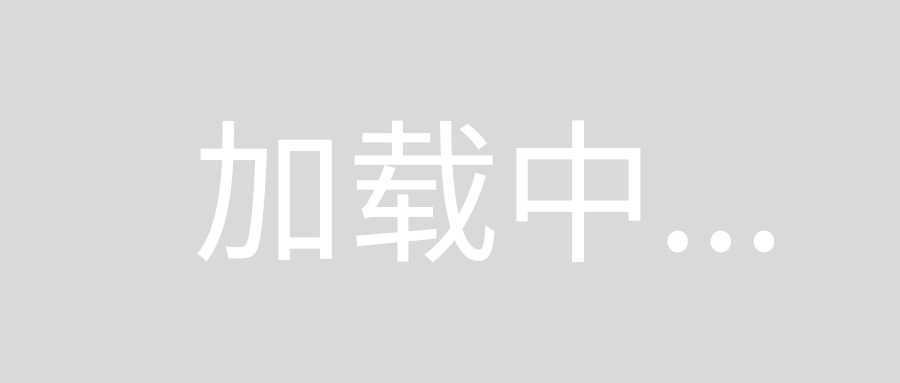
The texts are from my core data base, they are string attributes.
Between the screen 1 and 2, the only thing changed is the string.
If I print the strings in the console I have the correct texts :
my amazing new title
Another funny title for demo
The constraints of my textview :
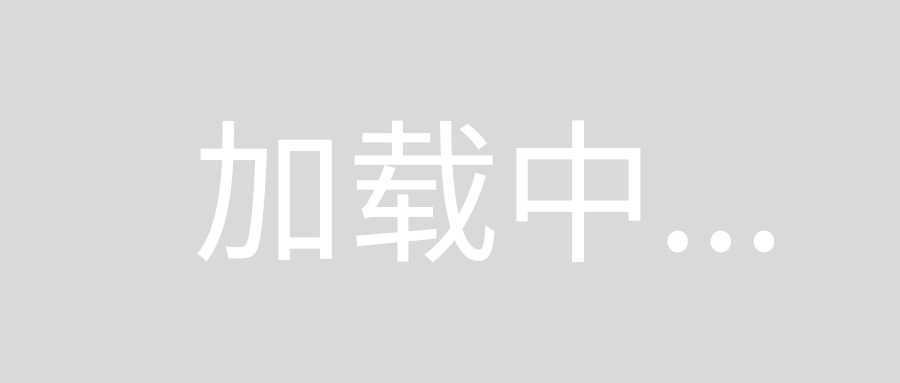
I don't understand why I have 2 different displays.
EDIT
I tried the @Nate advice, in viewDidLoad, I added:
myTextViewTitle.text="my amazing new title"
myTextViewTitle.setContentHuggingPriority(1000, forAxis: UILayoutConstraintAxis.Vertical)
myTextViewTitle.setContentCompressionResistancePriority(1000, forAxis: UILayoutConstraintAxis.Vertical)
myTextViewTitle.setTranslatesAutoresizingMaskIntoConstraints(false)
let views = ["new_view": myTextViewTitle]
var constrs = NSLayoutConstraint.constraintsWithVisualFormat("|-8-[new_view]-8-|", options: NSLayoutFormatOptions(0), metrics: nil, views: views)
self.view.addConstraints(constrs)
self.view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:|-8-[new_view]", options: NSLayoutFormatOptions(0), metrics: nil, views: views))
self.myTextViewTitle.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:[new_view(220@300)]", options: NSLayoutFormatOptions(0), metrics: nil, views: views))
No results. With or without height contraint added for my textview in interface builder...
EDIT 2
I need an UITextView and not an UIlabel, because of the back button, to use an exclusion path.
let exclusionPathBack:UIBezierPath = UIBezierPath(rect: CGRect(x:backButton.bounds.origin.x, y:backButton.bounds.origin.y, width:backButton.bounds.width+10, height:backButton.bounds.height))
myTextViewTitle.textContainer.exclusionPaths=[exclusionPathBack]
I found a solution.
I was focus on the height of my UITextView, then I read a post talking about the aspect ratio. I wanted to try and that worked!
So in the storyboard, I added an aspect ratio for the textview, and I checked "Remove at build time" option.
In viewDidLayoutSubviews(), I wrote :
override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()
let contentSize = self.myTextViewTitle.sizeThatFits(self.myTextViewTitle.bounds.size)
var frame = self.myTextViewTitle.frame
frame.size.height = contentSize.height
self.myTextViewTitle.frame = frame
aspectRatioTextViewConstraint = NSLayoutConstraint(item: self.myTextViewTitle, attribute: .Height, relatedBy: .Equal, toItem: self.myTextViewTitle, attribute: .Width, multiplier: myTextViewTitle.bounds.height/myTextViewTitle.bounds.width, constant: 1)
self.myTextViewTitle.addConstraint(aspectRatioTextViewConstraint!)
}
It works perfectly.
First, disable UITextView's scrollable.
Two options:
- uncheck Scrolling Enabled in .xib.
- [TextView setScrollEnabled:NO];
Create a UITextView and connect it with IBOutlet (TextView).
Add a UITextView height constraint with default height, connect it with IBOutlet (TextViewHeightConstraint).
When you set your UITextView’s text asynchronously you should calculate the height of UITextView and set UITextView’s height constraint to it.
Sample code:
CGSize sizeThatFitsTextView = [TextView sizeThatFits:CGSizeMake(TextView.frame.size.width, MAXFLOAT)];
TextViewHeightConstraint.constant = sizeThatFitsTextView.height;
In Swift 3.0, where tvHeightConstraint is the height constraint of the subject TextView (if using for multiple textviews, would add it to the function inputs):
func textViewDidChange(textView: UITextView) {
let size = textView.sizeThatFits(CGSize(width: textView.frame.size.width, height: CGFloat.greatestFiniteMagnitude))
if size.height != tvHeightConstraint.constant && size.height > textView.frame.size.height{
tvHeightConstraint.constant = size.height
textView.setContentOffset(CGPoint.zero, animated: false)
}
}
H/T to @cmi and @Pablo Ruan
In swift 2.3:
func textViewDidChange(textView: UITextView) {
let size = textView.sizeThatFits(CGSize(width: textView.frame.size.width, height: CGFloat.max))
if size.height != TXV_Height.constant && size.height > textView.frame.size.height{
TXV_Height.constant = size.height
textView.setContentOffset(CGPointZero, animated: false)
}
}
Why not have an image for the back button and a UILabel for your text, and use autolayout to position them? And maybe a third view for the yellow background.
My simple solution to an extremely similar problem:
I used labels with the number of lines set to 0 instead of trying to use text fields or views. Then I clipped the leading and trailing edges to the labels' containers which constrain their widths in my case, with 0 as the constant for the constraints. The catch is to set the number of lines to 0 to allow the labels to grow or shrink according to their content. The text never gets chopped and the height of the labels never exceed their contents' for all of the different size classes I configured for the widths (the container widths in my case). Tested for IOS 8.0 and later (also make sure 'explicit' is unchecked next to 'Preferred Width' attributes for all lables for the label widths to adjust for different size classes) - works perfectly.
I have adapted your code and used it for both: layout subview and textViewDidChange. Thank you very much that made my day after an hour of troubleshooting...
override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()
textViewDidChange(self.recordingTitleTV)
}
func textViewDidChange(_ textView: UITextView) {
let contentSize = textView.sizeThatFits(textView.bounds.size)
var frame = textView.frame
frame.size.height = contentSize.height
textView.frame = frame
let aspectRatioTextViewConstraint = NSLayoutConstraint(item: textView, attribute: .height, relatedBy: .equal, toItem: textView, attribute: .width, multiplier: textView.bounds.height/textView.bounds.width, constant: 1)
textView.addConstraint(aspectRatioTextViewConstraint)
}